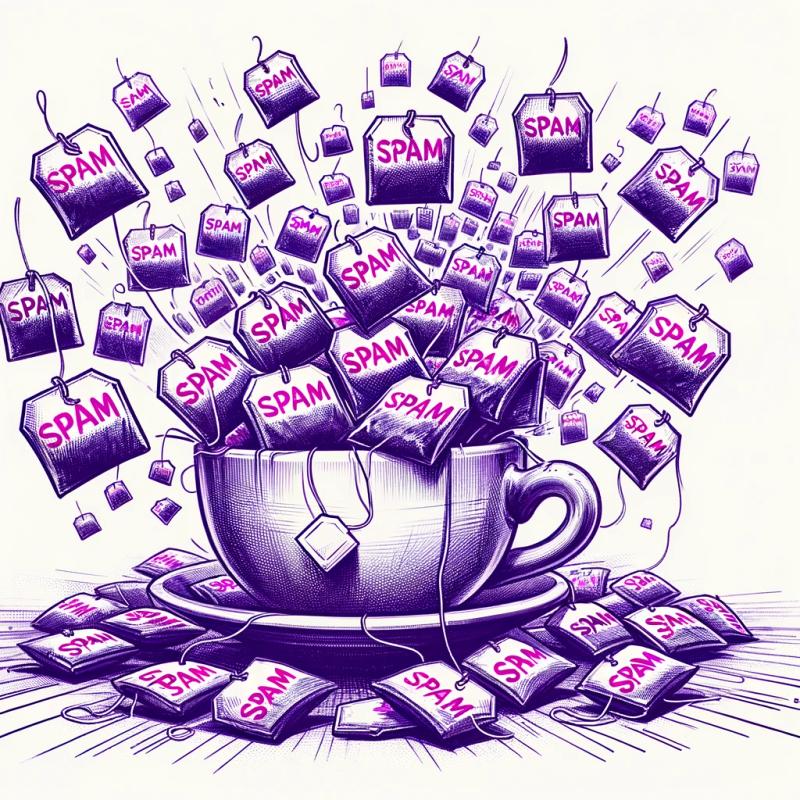
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@ceeblue/webrtc-client
Advanced tools
Readme
Requirements | Usage | Examples | Building locally | Documentation | Contribution | License
The Ceeblue WebRTC Client is a generic client library designed to simplify the implementation of WebRTC functionalities for Ceeblue customers.
The client library addresses common challenges faced by developers:
Item | Description |
---|---|
Ceeblue Account | To create a Stream, you will need an active account with Ceeblue Streaming Cloud. A trial account is sufficient. If you do not have one yet, you can request one on the Ceeblue website. |
Stream | To use this library, you'll first need to create a stream either through our Rest API or on the dashboard. Use the following steps:
|
Node Package Manager (npm) | Download and install from https://nodejs.org/en/download |
http-server | (Optional) Simple, zero-configuration command-line static HTTP server The http-server is useful to explore the WebRTC client examples or the documentation locally when you do not have a host. To start the server, use the following command: http-server . -p 8081 |
Add the library as a dependency to your npm project using:
npm install @ceeblue/webrtc-client
Then import the library into your project with:
import * as WebRTC from '@ceeblue/webrtc-client';
💡 TIP
- If your project uses TypeScript, it is recommended to set
"target": "ES6"
in your configuration. This setting aligns with our usage of ES6 features and ensures that your build will succeed. For those requiring a backwards-compatible UMD (Universal Module Definition) version, a local build is advised. Defining the compiler option"moduleResolution": "Node"
in tsconfig.json helps with import errors by ensuring that TypeScript uses the correct strategy for resolving imports based on the targeted Node.js version.
{ "compilerOptions": { "target": "ES6", "moduleResolution": "Node" } }
To publish the stream <streamName>
to <endpoint>
, use the Streamer class and the variables you saved while setting up the stream in the dashboard Requirements. For a full example, see push.html in Examples.
import Streamer as WebRTC from '@ceeblue/webrtc-client';
const streamer = new Streamer();
streamer.onStart = stream => {
console.log('start streaming');
}
streamer.onStop = _ => {
console.log('stop streaming');
}
navigator.mediaDevices
.getUserMedia({ audio: true, video: true })
.then(stream => {
streamer.start(stream, {
host: <endpoint>,
streamName: <streamName>,
iceServer: {
urls: ['turn:' + <endPoint> + ':3478?transport=tcp', 'turn:' + <endPoint> + ':3478'],
username: 'csc_demo', credential: 'UtrAFClFFO'
}
}
});
To play the stream <streamName>
from <endPoint>
, use the Player class and the variables you saved while setting up the stream in the dashboard Requirements. For a full example, see play.html in Examples.
import Player as WebRTC from '@ceeblue/webrtc-client';
const player = new Player();
player.onStart = stream => {
videoElement.srcObject = stream;
console.log('start playing');
}
player.onStop = _ => {
console.log('stop playing');
}
streamer.start(stream, {
host: <endPoint>,
streamName: <streamName>,
iceServer: {
urls: ['turn:' + <endPoint> + ':3478?transport=tcp', 'turn:' + <endPoint> + ':3478'],
username: 'csc_demo', credential: 'UtrAFClFFO'
}
}
To understand how to use the library through examples, we provide three illustrations of its implementation:
In your project directory, if you have installed the simple-http package, execute the following command from the Terminal prompt by navigating to:
http-server . -p 8081
Navigate to the specified address in your browser, making sure to replace any placeholders in the URL with the variables you have copied during the stream setup in the dashboard.
http://localhost:8081/examples/streamer.html?host=<endpoint>&stream=<streamName>
Click on Start streaming. Upon doing so, a live stream from your webcam will initiate. Should your browser request permission to access your camera, ensure to grant it.
In the address bar of a separate browser window, enter the following address, making sure to replace the placeholders in the URL with the variables you have copied while configuring the stream setup in the dashboard.
http://localhost:8081/examples/player.html?host=<endpoint>&stream=<streamName>
Click Play to start watching the live stream.
webrtc-client
folder and run npm install
to install packages dependencies.npm run build
. The output will be five files placed in the /dist/ folder:
git clone https://github.com/CeeblueTV/webrtc-client.git
cd webrtc-client
npm install
npm run build
💡 TIP
To build a UMD (Universal Module Definition) version compatible with older browsers, you can run the following command:
npm run build:es5
To automatically build bundles when any modification has been applied, you can run a watch command. The watch command will continuously monitor your project files for changes and rebuild the bundles accordingly.
npm run watch
Or for ES5 (UMD):
npm run watch:es5
This monorepo also contains built-in documentation about the APIs in the library, which can be built using the following npm command:
npm run build:docs
You can access the documentation by opening the index.html file in the docs folder with your browser (./docs/index.html
), or, if you have installed and started the http-server, by navigating to:
http://localhost:8081/docs/
All contributions are welcome. Please see our contribution guide for details.
By contributing code to this project, you agree to license your contribution under the GNU Affero General Public License.
FAQs
Ceeblue WebRTC Client
The npm package @ceeblue/webrtc-client receives a total of 100 weekly downloads. As such, @ceeblue/webrtc-client popularity was classified as not popular.
We found that @ceeblue/webrtc-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.