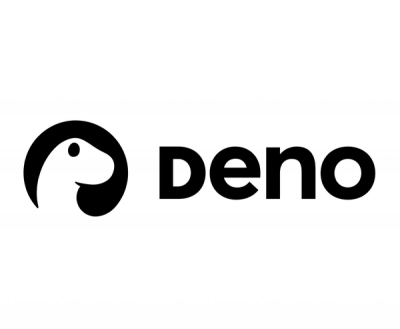
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@changesets/changelog-github
Advanced tools
A changelog entry generator for GitHub that links to commits, PRs and users
@changesets/changelog-github is an npm package designed to generate changelogs for your GitHub repository. It integrates with the Changesets workflow to automatically create detailed and formatted changelogs based on your commit history and pull requests.
Generate Changelog Entries
This feature allows you to generate a changelog entry for a specific changeset and version. The `getChangelogEntry` function takes a changeset object and a version string, and returns a formatted changelog entry.
const { getChangelogEntry } = require('@changesets/changelog-github');
async function generateChangelogEntry(changeset, version) {
const entry = await getChangelogEntry(changeset, version);
console.log(entry);
}
generateChangelogEntry({
summary: 'Added new feature',
releases: [{ name: 'my-package', type: 'minor' }]
}, '1.1.0');
Custom Changelog Templates
This feature allows you to use custom templates for generating changelog entries. By providing a template function, you can customize the format of the changelog entries to fit your project's needs.
const { getChangelogEntry } = require('@changesets/changelog-github');
async function generateCustomChangelogEntry(changeset, version) {
const entry = await getChangelogEntry(changeset, version, {
template: ({ changeset, version }) => `## ${version}\n- ${changeset.summary}`
});
console.log(entry);
}
generateCustomChangelogEntry({
summary: 'Fixed a bug',
releases: [{ name: 'my-package', type: 'patch' }]
}, '1.0.1');
conventional-changelog is a package that generates changelogs based on conventional commit messages. It is highly configurable and supports various preset configurations. Unlike @changesets/changelog-github, it focuses on conventional commit messages and offers a wide range of customization options.
standard-version is a tool that automates versioning and changelog generation based on conventional commit messages. It is similar to @changesets/changelog-github in that it helps automate the release process, but it is more focused on the entire release workflow, including version bumping and tagging.
release-it is a versatile release automation tool that can handle versioning, changelog generation, and publishing to npm and GitHub. It offers more comprehensive release management compared to @changesets/changelog-github, which is more focused on changelog generation.
FAQs
A changelog entry generator for GitHub that links to commits, PRs and users
The npm package @changesets/changelog-github receives a total of 241,839 weekly downloads. As such, @changesets/changelog-github popularity was classified as popular.
We found that @changesets/changelog-github demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.