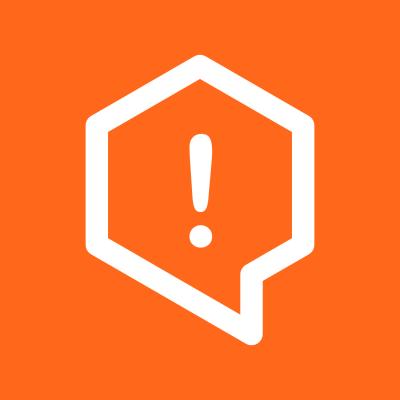
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@civic/auth
Advanced tools
- [Civic Auth Client SDK](#civic-auth-client-sdk) - [Quick Start](#quick-start) - [npm](#npm) - [yarn](#yarn) - [pnpm](#pnpm) - [bun](#bun) - [Integration](#integration) - [React](#react) - [Usage](#usage) - [The User Button](#th
Civic Auth offers a simple, flexible, and fast way to integrate authentication into your applications.
You can find the full, official documentation here, with a quick-start version in this README.
Sign up for Civic Auth in less than a minute at auth.civic.com to get your Client ID.
Install the library in your app using your installer of choice:
npm install @civic/auth
yarn add @civic/auth
pnpm install @civic/auth
bun add @civic/auth
Choose your framework for instructions on how to integrate Civic Auth into your application.
For integrating in other environments using any OIDC or OAuth 2.0-compliant client libraries, see here.
Integrate Civic Auth into your React application with ease, just wrap your app with the Civic Auth provider and add your Client ID (provided after you sign up). A working example is available in our github examples repo.
import { CivicAuthProvider, UserButton } from "@civic/auth/react";
function App({ children }) {
return (
<CivicAuthProvider clientId="YOUR CLIENT ID">
<UserButton />
{children}
</CivicAuthProvider>
)
}
The Civic Auth SDK comes with a multi-purpose styled component called the UserButton
import { UserButton, CivicAuthProvider } from '@civic/auth/react';
export function TitleBar() {
return (
<div className="flex justify-between items-center">
<h1>My App</h1>
<UserButton />
</div>
);
};
function App({ children }) {
return (
<CivicAuthProvider clientId="YOUR CLIENT ID">
<TitleBar />
</CivicAuthProvider>
)
}
This component is context-dependent. If the user is logged in, it will show their profile picture and name. If the user is not logged in, it will show a Log In button.
Use the Civic Auth SDK to retrieve user information on the frontend.
import { useUser } from '@civic/auth/react';
export function MyComponent() {
const { user } = useUser();
if (!user) return <div>User not logged in</div>
return <div>Hello { user.name }!</div>
}
We use name
as an example here, but you can call any user object property from the user fields schema, as shown below.
Civic Auth is a "low-code" solution, so all configuration takes place via the dashboard. Changes you make there will be updated automatically in your integration without any code changes. The only required parameter you need to provide is the client ID.
The integration provides additional run-time settings and hooks that you can use to customize the library's integration with your own app. If you need any of these, you can add them to the CivicAuthProvider as follows:
<CivicAuthProvider
clientId="YOUR CLIENT ID"
...other configuration
>
See below for the list of all configuration options
Field | Required | Default | Example | Description |
---|---|---|---|---|
clientId | Yes | - | 2cc5633d-2c92-48da-86aa-449634f274b9 | The key obtained on signup to auth.civic.com |
nonce | No | - | 1234 | A single-use ID used during login, binding a login token with a given client. Needed in advanced authentication processes only |
onSignIn | No | - |
| A hook that executes after a sign-in attempt, whether successful or not. |
onSignOut | No | - |
| A hook that executes after a user logs out. |
redirectUrl | No | currentURL | /authenticating | An override for the page that OAuth will redirect to to perform token-exchange. By default Civic will redirect to the current URL and Authentication will be finished by the Civic provider automatically. Only use if you'd like to have some custom display or logic during OAuth token-exchange. The redirect page must have the CivicAuthProvider running in order to finish authentication. |
modalIframe | No | true | modalIframe={true} | Set to true if you want to embed the login iframe in your app rather than opening the iframe in a modal. See Embedded Login Iframe section below. |
The display mode indicates where the Civic login UI will be displayed. The following display modes are supported:
iframe
(default): the UI loads in an iframe that shows in an overlay on top of the existing page contentredirect
: the UI redirects the current URL to a Civic login screen, then redirects back to your site when login is completenew_tab
: the UI opens in a new tab or popup window (depending on browser preferences), and after login is complete, the tab or popup closes to return the user to your siteThe full user context object (provided by useUser
) looks like this:
{
user: User | null;
// these are the OAuth tokens created during authentication
idToken?: string;
accessToken?: string;
refreshToken?: string;
forwardedTokens?: ForwardedTokens;
// functions and flags for UI and signIn/signOut
isLoading: boolean;
error: Error | null;
signIn: (displayMode?: DisplayMode) => Promise<void>;
signOut: () => Promise<void>;
}
The User
object looks like this:
type BaseUser = {
id: string;
email?: string;
name?: string;
picture?: string;
given_name?: string;
family_name?: string;
updated_at?: Date;
};
type User<T extends UnknownObject = EmptyObject> = BaseUser & T;
Where you can pass extra user attributes to the object that you know will be present in user claims, e.g.
const UserWithNickName = User<{ nickname: string }>;
Field descriptions:
Field | |
---|---|
id | The user's unique ID with respect to your app. You can use this to look up the user in the dashboard. |
The user's email address | |
name | The user's full name |
given_name | The user's given name |
family_name | The user's family name |
updated_at | The time at which the user's profile was most recently updated. |
Typically developers will not need to interact with the token fields, which are used only for advanced use cases.
Field | |
---|---|
idToken | The OIDC id token, used to request identity information about the user |
accessToken | The OAuth 2.0 access token, allowing a client to make API calls to Civic Auth on behalf of the user. |
refreshToken | The OAuth 2.0 refresh token, allowing a login session to be extended automatically without requiring user interaction. The Civic Auth SDK handles refresh automatically, so you do not need to do this. |
forwardedTokens | If the user authenticated using SSO (single-sign-on login) with a federated OAuth provider such as Google, this contains the OIDC and OAuth 2.0 tokens from that provider. |
Use forwardedTokens if you need to make requests to the source provider, such as find out provider-specific information.
An example would be, if a user logged in via Google, using the Google forwarded token to query the Google Groups that the user is a member of.
For example:
const googleAccessToken = user.forwardedTokens?.google?.accessToken;
If you want to have the Login screen open directly on a page without the user having to click on button, you can import the CivicAuthIframeContainer
component and use it along with the AuthProvider option iframeMode={"embedded"}
You just need to ensure that the CivicAuthIframeContainer
is a child under a CivicAuthProvider
import { CivicAuthIframeContainer } from "@civic/auth/react";
const Login = () => {
return (
<div class="login-container">
<CivicAuthIframeContainer />
</div>
);
};
const App = () => {
return (
<CivicAuthProvider
clientId={"YOUR CLIENT ID"}
iframeMode={"embedded"}
>
<Login />
</CivicAuthProvider>
);
}
Integrate Civic Auth into your Next.js application using the following steps (a working example is available in our github examples repo):
This is where you give your app the Client ID provided when you sign up at auth.civic.com.
The defaults should work out of the box for most customers, but if you want to configure your app, see below for details.
import { createCivicAuthPlugin } from "@civic/auth/nextjs"
import type { NextConfig } from "next";
const nextConfig: NextConfig = {
/* config options here */
};
const withCivicAuth = createCivicAuthPlugin({
clientId: 'YOUR CLIENT ID'
});
export default withCivicAuth(nextConfig)
This is where your app will handle login and logout requests.
Create this file at the following path:
src/app/api/auth/[...civicauth]/route.ts
import { handler } from '@civic/auth/nextjs'
export const GET = handler()
These steps apply to the App Router. If you are using the Pages Router, please contact us for integration steps.
Middleware is used to protect your backend routes, server components and server actions from unauthenticated requests.
Using the Civic Auth middleware ensures that only logged-in users have access to secure parts of your service.
import { authMiddleware } from '@civic/auth/nextjs/middleware'
export default authMiddleware()
export const config = {
// include the paths you wish to secure here
matcher: [ '/api/:path*', '/admin/:path*' ]
}
If you are already using middleware in your Next.js app, then you can chain them with Civic Auth as follows:
import { auth } from '@civic/auth/nextjs'
import { NextRequest, NextResponse } from "next/server";
const withCivicAuth = auth()
const otherMiddleware = (request: NextRequest) => {
console.log('my middleware')
return NextResponse.next()
}
export default withCivicAuth(otherMiddleware)
Add the Civic Auth context to your app to give your frontend access to the logged-in user.
import { CivicAuthProvider } from "@civic/auth/nextjs";
function Layout({ children }) {
return (
// ... the rest of your app layout
<CivicAuthProvider>
{children}
</CivicAuthProvider>
)
}
Unlike the pure React integration, you do not have to add your client ID again here!
Next.JS client components can use the Civic Auth React tools to obtain user information as well as display convenient login, and logout buttons. See the React Usage page for details.
Retrieve user information on backend code, such as in React Server Components, React Server Actions, or api routes using getUser
:
import { getUser } from '@civic/auth/nextjs';
const user = await getUser();
For example, in a NextJS Server Component:
import { getUser } from '@civic/auth/nextjs';
export async function MyServerComponent() {
const user = await getUser();
if (!user) return <div>User not logged in</div>
return <div>Hello { user.name }!</div>
}
The name
property is used as an example here, check out the React Usage page to see the entire basic user object structure.
Civic Auth is a "low-code" solution, so most of the configuration takes place via the dashboard. Changes you make there will be updated automatically in your integration without any code changes. The only required parameter you need to provide is the client ID.
The integration also offers the ability customize the library according to the needs of your Next.js app. For example, to restrict authentication checks to specific pages and routes in your app. You can do so inside next.config.js
as follows:
const withCivicAuth = createCivicAuthPlugin({
clientId: 'YOUR CLIENT ID'
...other config
});
export default withCivicAuth(nextConfig) // your next config here
Here are the available configuration options:
Field | Required | Default | Example | Description |
---|---|---|---|---|
clientId | Yes | - | 2cc5633d-2c92-48da-86aa-449634f274b9 | The key obtained on signup to auth.civic.com |
callbackUrl | No | /api/auth/callback | /api/myroute/callback | The path to route the browser to after a succesful login. Set this value if you are hosting your civic auth API route somewhere other than the default recommended above. |
loginUrl | No | / | /admin | The path your user will be sent to if they access a resource that needs them to be logged in. If you have a dedicated login page, you can set it here. |
logoutUrl | No | / | /goodbye | The path your user will be sent to after a successful log-out. |
include | No | ['/*'] | [ '/admin/*', '/api/admin/*' ] | An array of path globs that require a user to be logged-in to access. If not set, will include all paths matched by your Next.js middleware. |
exclude | No | - | ['public/home'] | An array of path globs that are excluded from the Civic Auth middleware. In some cases, it might be easier and safer to specify exceptions rather than keep an inclusion list up to date. |
FAQs
- [Civic Auth Client SDK](#civic-auth-client-sdk) - [Quick Start](#quick-start) - [npm](#npm) - [yarn](#yarn) - [pnpm](#pnpm) - [bun](#bun) - [Integration](#integration) - [React](#react) - [Usage](#usage) - [The User Button](#th
The npm package @civic/auth receives a total of 324 weekly downloads. As such, @civic/auth popularity was classified as not popular.
We found that @civic/auth demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.