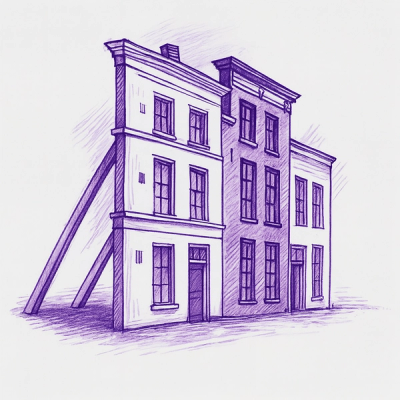
Security News
Potemkin Understanding in LLMs: New Study Reveals Flaws in AI Benchmarks
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
@coderich/autograph
Advanced tools
:heavy_check_mark: MongoDB :heavy_check_mark: Neo4j
AutoGraph is a unified API to query and mutate data defined in a GraphQL Schema. It's a data resolver and business logic handler that can be used in and outside of a GraphQL server.
Features include:
:fire: If you're looking to build a GraphQL Server API, check out AutoGraphServer!
First, install AutoGraph via NPM:
npm i @coderich/autograph --save
To get started, create a Resolver
. Each Resolver
provides a context to run queries for a given Schema
.
const { Resolver } = require('@coderich/autograph');
const resolver = new Resolver(schema);
That's it! Now you're ready to use the resolver to query and mutate data.
Refer to the documentation below for how to define a schema.
Each Resolver
treats your schema definition as a graph of connected nodes. To begin a query or mutation, you must first identify a node in the graph as your starting point.
Identify a node, returns a QueryBuilder
.
const queryBuilder = resolver.match('Person');
Identify a node, returns a Transaction
.
const txn = resolver.transaction('Person');
FAQs
AutoGraph
The npm package @coderich/autograph receives a total of 720 weekly downloads. As such, @coderich/autograph popularity was classified as not popular.
We found that @coderich/autograph demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.