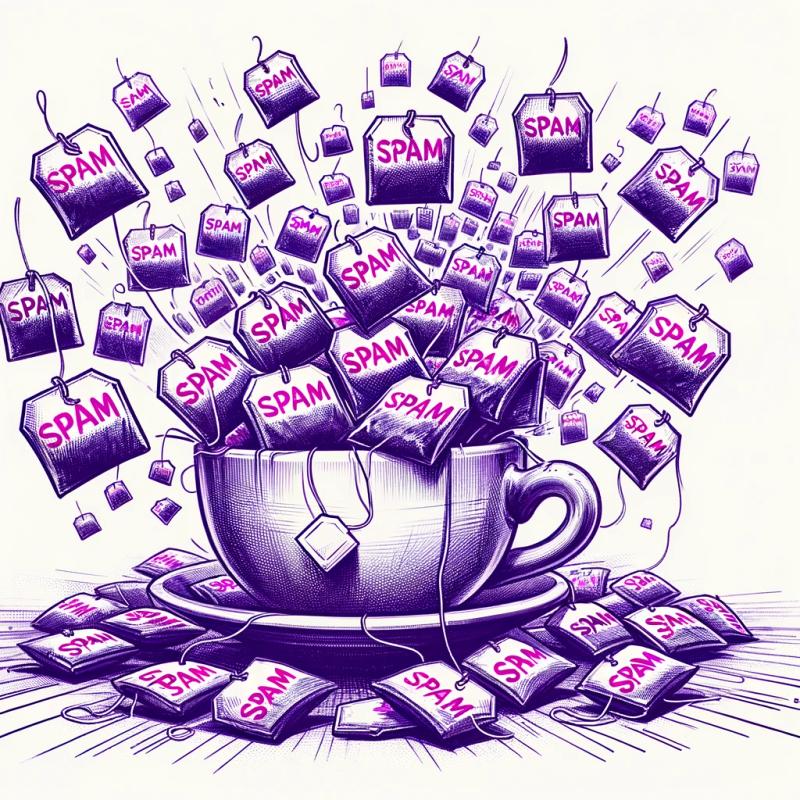
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@codesandbox/sandpack-client
Advanced tools
Readme
This is a small foundation package that sits on top of the bundler. It is framework agnostic and facilitates the handshake between your context and the bundler iframe.
import { loadSandpackClient } from "@codesandbox/sandpack-client";
const main = async () => {
const client = await loadSandpackClient("#preview", {
files: {
"/index.js": {
code: `console.log(require('uuid'))`,
},
},
entry: "/index.js",
dependencies: {
uuid: "latest",
},
});
}
FAQs
Unknown package
The npm package @codesandbox/sandpack-client receives a total of 64,726 weekly downloads. As such, @codesandbox/sandpack-client popularity was classified as popular.
We found that @codesandbox/sandpack-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.