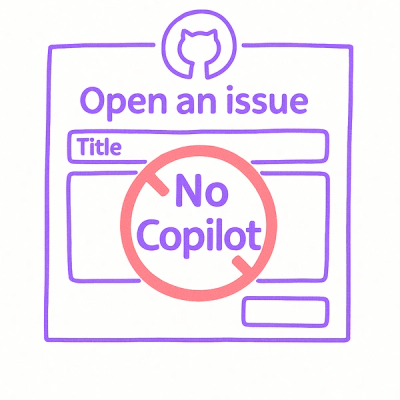
Security News
Open Source Maintainers Demand Ability to Block Copilot-Generated Issues and PRs
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
@datafire/google_factchecktools
Advanced tools
Client library for Fact Check Tools API
npm install --save @datafire/google_factchecktools
let google_factchecktools = require('@datafire/google_factchecktools').create({
access_token: "",
refresh_token: "",
client_id: "",
client_secret: "",
redirect_uri: ""
});
.then(data => {
console.log(data);
});
Exchange the code passed to your redirect URI for an access_token
google_factchecktools.oauthCallback({
"code": ""
}, context)
object
string
object
string
string
string
string
string
Exchange a refresh_token for an access_token
google_factchecktools.oauthRefresh(null, context)
This action has no parameters
object
string
string
string
string
string
Search through fact-checked claims.
google_factchecktools.factchecktools.claims.search({}, context)
object
string
: The BCP-47 language code, such as "en-US" or "sr-Latn". Can be used to restrict results by language, though we do not currently consider the region.integer
: The maximum age of the returned search results, in days. Age is determined by either claim date or review date, whichever is newer.integer
: An integer that specifies the current offset (that is, starting result location) in search results. This field is only considered if page_token
is unset. For example, 0 means to return results starting from the first matching result, and 10 means to return from the 11th result.integer
: The pagination size. We will return up to that many results. Defaults to 10 if not set.string
: The pagination token. You may provide the next_page_token
returned from a previous List request, if any, in order to get the next page. All other fields must have the same values as in the previous request.string
: Textual query string. Required unless review_publisher_site_filter
is specified.string
: The review publisher site to filter results by, e.g. nytimes.com.string
(values: 1, 2): V1 error format.string
: OAuth access token.string
(values: json, media, proto): Data format for response.string
: JSONPstring
: Selector specifying which fields to include in a partial response.string
: API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.string
: OAuth 2.0 token for the current user.boolean
: Returns response with indentations and line breaks.string
: Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.string
: Upload protocol for media (e.g. "raw", "multipart").string
: Legacy upload protocol for media (e.g. "media", "multipart").List the ClaimReview
markup pages for a specific URL or for an organization.
google_factchecktools.factchecktools.pages.list({}, context)
object
integer
: An integer that specifies the current offset (that is, starting result location) in search results. This field is only considered if page_token
is unset, and if the request is not for a specific URL. For example, 0 means to return results starting from the first matching result, and 10 means to return from the 11th result.string
: The organization for which we want to fetch markups for. For instance, "site.com". Cannot be specified along with an URL.integer
: The pagination size. We will return up to that many results. Defaults to 10 if not set. Has no effect if a URL is requested.string
: The pagination token. You may provide the next_page_token
returned from a previous List request, if any, in order to get the next page. All other fields must have the same values as in the previous request.string
: The URL from which to get ClaimReview
markup. There will be at most one result. If markup is associated with a more canonical version of the URL provided, we will return that URL instead. Cannot be specified along with an organization.string
(values: 1, 2): V1 error format.string
: OAuth access token.string
(values: json, media, proto): Data format for response.string
: JSONPstring
: Selector specifying which fields to include in a partial response.string
: API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.string
: OAuth 2.0 token for the current user.boolean
: Returns response with indentations and line breaks.string
: Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.string
: Upload protocol for media (e.g. "raw", "multipart").string
: Legacy upload protocol for media (e.g. "media", "multipart").Create ClaimReview
markup on a page.
google_factchecktools.factchecktools.pages.create({}, context)
object
string
(values: 1, 2): V1 error format.string
: OAuth access token.string
(values: json, media, proto): Data format for response.string
: JSONPstring
: Selector specifying which fields to include in a partial response.string
: API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.string
: OAuth 2.0 token for the current user.boolean
: Returns response with indentations and line breaks.string
: Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.string
: Upload protocol for media (e.g. "raw", "multipart").string
: Legacy upload protocol for media (e.g. "media", "multipart").Delete all ClaimReview
markup on a page.
google_factchecktools.factchecktools.pages.delete({
"name": ""
}, context)
object
string
: The name of the resource to delete, in the form of pages/{page_id}
.string
(values: 1, 2): V1 error format.string
: OAuth access token.string
(values: json, media, proto): Data format for response.string
: JSONPstring
: Selector specifying which fields to include in a partial response.string
: API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.string
: OAuth 2.0 token for the current user.boolean
: Returns response with indentations and line breaks.string
: Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.string
: Upload protocol for media (e.g. "raw", "multipart").string
: Legacy upload protocol for media (e.g. "media", "multipart").Get all ClaimReview
markup on a page.
google_factchecktools.factchecktools.pages.get({
"name": ""
}, context)
object
string
: The name of the resource to get, in the form of pages/{page_id}
.string
(values: 1, 2): V1 error format.string
: OAuth access token.string
(values: json, media, proto): Data format for response.string
: JSONPstring
: Selector specifying which fields to include in a partial response.string
: API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.string
: OAuth 2.0 token for the current user.boolean
: Returns response with indentations and line breaks.string
: Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.string
: Upload protocol for media (e.g. "raw", "multipart").string
: Legacy upload protocol for media (e.g. "media", "multipart").Update for all ClaimReview
markup on a page Note that this is a full update. To retain the existing ClaimReview
markup on a page, first perform a Get operation, then modify the returned markup, and finally call Update with the entire ClaimReview
markup as the body.
google_factchecktools.factchecktools.pages.update({
"name": ""
}, context)
object
string
: The name of this ClaimReview
markup page resource, in the form of pages/{page_id}
. Except for update requests, this field is output-only and should not be set by the user.string
(values: 1, 2): V1 error format.string
: OAuth access token.string
(values: json, media, proto): Data format for response.string
: JSONPstring
: Selector specifying which fields to include in a partial response.string
: API key. Your API key identifies your project and provides you with API access, quota, and reports. Required unless you provide an OAuth 2.0 token.string
: OAuth 2.0 token for the current user.boolean
: Returns response with indentations and line breaks.string
: Available to use for quota purposes for server-side applications. Can be any arbitrary string assigned to a user, but should not exceed 40 characters.string
: Upload protocol for media (e.g. "raw", "multipart").string
: Legacy upload protocol for media (e.g. "media", "multipart").object
: Information about the claim.
string
: The date that the claim was made.array
: One or more reviews of this claim (namely, a fact-checking article).
string
: A person or organization stating the claim. For instance, "John Doe".string
: The claim text. For instance, "Crime has doubled in the last 2 years."object
: Information about the claim author.
string
: Corresponds to ClaimReview.itemReviewed.author.image
.string
: Corresponds to ClaimReview.itemReviewed.author.jobTitle
.string
: A person or organization stating the claim. For instance, "John Doe". Corresponds to ClaimReview.itemReviewed.author.name
.string
: Corresponds to ClaimReview.itemReviewed.author.sameAs
.object
: Information about the claim rating.
integer
: For numeric ratings, the best value possible in the scale from worst to best. Corresponds to ClaimReview.reviewRating.bestRating
.string
: Corresponds to ClaimReview.reviewRating.image
.string
: Corresponds to ClaimReview.reviewRating.ratingExplanation
.integer
: A numeric rating of this claim, in the range worstRating — bestRating inclusive. Corresponds to ClaimReview.reviewRating.ratingValue
.string
: The truthfulness rating as a human-readible short word or phrase. Corresponds to ClaimReview.reviewRating.alternateName
.integer
: For numeric ratings, the worst value possible in the scale from worst to best. Corresponds to ClaimReview.reviewRating.worstRating
.object
: Information about a claim review.
string
: The language this review was written in. For instance, "en" or "de".string
: The date the claim was reviewed.string
: Textual rating. For instance, "Mostly false".string
: The title of this claim review, if it can be determined.string
: The URL of this claim review.object
: Information about the claim review author.
string
: Corresponds to ClaimReview.author.image
.string
: Name of the organization that is publishing the fact check. Corresponds to ClaimReview.author.name
.object
: Fields for an individual ClaimReview
element. Except for sub-messages that group fields together, each of these fields correspond those in https://schema.org/ClaimReview. We list the precise mapping for each field.
array
: A list of links to works in which this claim appears, aside from the one specified in claim_first_appearance
. Corresponds to ClaimReview.itemReviewed[@type=Claim].appearance.url
.
string
string
: The date when the claim was made or entered public discourse. Corresponds to ClaimReview.itemReviewed.datePublished
.string
: A link to a work in which this claim first appears. Corresponds to ClaimReview.itemReviewed[@type=Claim].firstAppearance.url
.string
: The location where this claim was made. Corresponds to ClaimReview.itemReviewed.name
.string
: A short summary of the claim being evaluated. Corresponds to ClaimReview.claimReviewed
.string
: This field is optional, and will default to the page URL. We provide this field to allow you the override the default value, but the only permitted override is the page URL plus an optional anchor link ("page jump"). Corresponds to ClaimReview.url
object
: Holds one or more instances of ClaimReview
markup for a webpage.
array
: A list of individual claim reviews for this page. Each item in the list corresponds to one ClaimReview
element.
string
: The name of this ClaimReview
markup page resource, in the form of pages/{page_id}
. Except for update requests, this field is output-only and should not be set by the user.string
: The URL of the page associated with this ClaimReview
markup. While every individual ClaimReview
has its own URL field, semantically this is a page-level field, and each ClaimReview
on this page will use this value unless individually overridden. Corresponds to ClaimReview.url
string
: The date when the fact check was published. Similar to the URL, semantically this is a page-level field, and each ClaimReview
on this page will contain the same value. Corresponds to ClaimReview.datePublished
string
: The version ID for this markup. Except for update requests, this field is output-only and should not be set by the user.object
: Response from searching fact-checked claims.
array
: The list of claims and all of their associated information.
string
: The next pagination token in the Search response. It should be used as the page_token
for the following request. An empty value means no more results.object
: Response from listing ClaimReview
markup.
array
: The result list of pages of ClaimReview
markup.
string
: The next pagination token in the Search response. It should be used as the page_token
for the following request. An empty value means no more results.object
: Information about the publisher.
string
: The name of this publisher. For instance, "Awesome Fact Checks".string
: Host-level site name, without the protocol or "www" prefix. For instance, "awesomefactchecks.com". This value of this field is based purely on the claim review URL.object
: A generic empty message that you can re-use to avoid defining duplicated empty messages in your APIs. A typical example is to use it as the request or the response type of an API method. For instance: service Foo { rpc Bar(google.protobuf.Empty) returns (google.protobuf.Empty); } The JSON representation for Empty
is empty JSON object {}
.FAQs
DataFire integration for Fact Check Tools API
The npm package @datafire/google_factchecktools receives a total of 0 weekly downloads. As such, @datafire/google_factchecktools popularity was classified as not popular.
We found that @datafire/google_factchecktools demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Open source maintainers are urging GitHub to let them block Copilot from submitting AI-generated issues and pull requests to their repositories.
Research
Security News
Malicious Koishi plugin silently exfiltrates messages with hex strings to a hardcoded QQ account, exposing secrets in chatbots across platforms.
Research
Security News
Malicious PyPI checkers validate stolen emails against TikTok and Instagram APIs, enabling targeted account attacks and dark web credential sales.