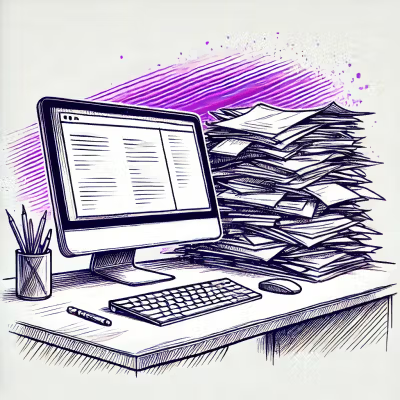
Security News
High Salaries No Longer Enough to Attract Top Cybersecurity Talent
A survey of 500 cybersecurity pros reveals high pay isn't enough—lack of growth and flexibility is driving attrition and risking organizational security.
@drivekyte/react-native-kustomer
Advanced tools
$ yarn add @drivekyte/react-native-kustomer
Set Kotlin version to 1.7.0
Linking the package manually is not required anymore with Autolinking.
$ react-native link @drivekyte/react-native-kustomer
Include the library in your android/app/build.gradle
:
gradle implementation 'com.kustomer.kustomersdk:kustomersdk:0.3.7'
<dependency>
<groupId>com.kustomer.kustomersdk</groupId>
<artifactId>kustomersdk</artifactId>
<version>0.3.7</version>
<type>pom</type>
</dependency>
The preferred installation method is with CocoaPods. Add the following to your Podfile
:
pod 'Kustomer', :git => 'https://github.com/kustomer/customer-ios.git', :tag => '0.3.9'
For Carthage, add the following to your Cartfile
:
github "kustomer/customer-ios" ~> 0.3.9
Add the following three activities to your AndroidManifest.xml
:
<activity android:name="com.kustomer.kustomersdk.Activities.KUSSessionsActivity" android:configChanges="orientation|screenSize|keyboardHidden" android:theme="@style/CustomKustomerTheme" />
<activity android:name="com.kustomer.kustomersdk.Activities.KUSChatActivity" android:configChanges="orientation|screenSize|keyboardHidden" android:theme="@style/CustomKustomerTheme" />
<activity android:name="com.kustomer.kustomersdk.Activities.KUSKnowledgeBaseActivity" android:configChanges="orientation|screenSize|keyboardHidden" android:theme="@style/CustomKustomerTheme" />
Initialize Kustomer in your MainApplication
onCreate
function:
import com.kustomer.kustomersdk.Kustomer;
public class MainApplication extends Application implements ReactApplication {
@Override
public void onCreate() {
Kustomer.init(this, API_KEY);
}
}
To customize Kustomer's theme, add a new style in styles.xml
overwriting the values you want to replace:
<style name="MySupportTheme" parent="CustomKustomerTheme">
<item name="colorPrimary">@color/kusToolbarBackgroundColor</item>
<item name="colorPrimaryDark">@color/kusStatusBarColor</item>
<item name="colorAccent">@color/kusColorAccent</item>
<item name="kus_back_image">@drawable/ic_arrow_back_black_24dp</item>
<item name="kus_dismiss_image">@drawable/ic_close_black_24dp</item>
<item name="kus_new_session_button_image">@drawable/ic_edit_white_20dp</item>
</style>
To edit Kustomer's UI colors, add the desired color to be replaced in colors.xml
:
<!-- CHAT HISTORY SCREEN -->
<color name="kusToolbarBackgroundColor">#000000</color>
<color name="kusStatusBarColor">#000000</color>
<color name="kusToolbarTintColor">#DD2C00</color>
<color name="kusSessionListBackground">#909090</color>
<color name="kusSessionItemBackground">#909090</color>
<color name="kusSessionItemSelectedBackground">#55FFFFFF</color>
<color name="kusSessionTitleColor">#FFFFFF</color>
<color name="kusSessionDateColor">#FFFFFF</color>
<color name="kusSessionSubtitleColor">#FFFFFF</color>
<color name="kusSessionUnreadColor">#FFFFFF</color>
<color name="kusSessionUnreadBackground">#3F51B5</color>
<color name="kusSessionPlaceholderBackground">#909090</color>
<color name="kusSessionPlaceholderLineColor">#55FFFFFF</color>
<color name="kusNewSessionButtonColor">#DD2C00</color>
<color name="kusNewSessionTextColor">#FFFFFF</color>
<!-- CHAT SCREEN -->
<color name="kusToolbarNameColor">#FFFFFF</color>
<color name="kusToolbarGreetingColor">#FFFFFF</color>
<color name="kusToolbarSeparatorColor">#BDBDBD</color>
<color name="kusToolbarUnreadTextColor">#FFFFFF</color>
<color name="kusToolbarUnreadBackground">#aacc0000</color>
<color name="kusEmailInputBackground">#FFFFFF</color>
<color name="kusEmailInputBorderColor">#DD2C00</color>
<color name="kusEmailInputPromptColor">#FFFFFF</color>
<color name="kusChatListBackground">#909090</color>
<color name="kusChatItemBackground">#909090</color>
<color name="kusChatTimestampTextColor">#FFFFFF</color>
<color name="kusCompanyBubbleColor">#000000</color>
<color name="kusCompanyTextColor">#FFFFFF</color>
<color name="kusUserBubbleColor">#DD2C00</color>
<color name="kusUserTextColor">#000000</color>
<color name="kusSendButtonColor">#DD2C00</color>
<color name="kusInputBarTintColor">#DD2C00</color>
<color name="kusInputBarHintColor">#EEEEEE</color>
<color name="kusInputBarTextColor">#FFFFFF</color>
<color name="kusInputBarBackground">#000000</color>
<color name="kusInputBarSeparatorColor">#BDBDBD</color>
<color name="kusInputBarAttachmentIconColor">#FFFFFF</color>
<color name="kusOptionPickerSeparatorColor">#BDBDBD</color>
<color name="kusOptionPickerButtonBorderColor">#2962FF</color>
<color name="kusOptionPickerButtonTextColor">#2962FF</color>
<color name="kusOptionPickerButtonBackground">#F5F5F5</color>
<!-- SATISFACTION FORM -->
<color name="kusCSatRatingPromptTextColor">#FFFFFF</color>
<color name="kusCSatQuestionsTextColor">#FFFFFF</color>
<color name="kusCSatRatingLabelsTextColor">#FFFFFF</color>
<color name="kusCSatFeedbackTextColor">#FFFFFF</color>
<color name="kusCSatEditTextColor">#DD2C00</color>
<color name="kusCSatCommentBorderColor">#FFFFFF</color>
<color name="kusCSatCommentInputTextColor">#FFFFFF</color>
<color name="kusCSatSubmitButtonColor">#DD2C00</color>
<color name="kusCSatSubmitTextColor">#000000</color>
Initialize Kustomer in your AppDelegate.m
didFinishLaunchingWithOptions
function:
#import <Kustomer/Kustomer.h>
@implementation AppDelegate
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions
{
[Kustomer initializeWithAPIKey:@"API_KEY"];
return YES;
}
Import the library to use its methods:
import KustomerSDK from '@drivekyte/react-native-kustomer';
Identify current user with a token, in order to recover its previous data, such as conversations and profile
KustomerSDK.identify(token);
Add data to the customer profile. You can use some pre-defined fields, or create custom ones from the dashboard.
KustomerSDK.describeCustomer(data);
Unlink the current identified user
KustomerSDK.resetTracking();
Show Kustomer's chat support UI
KustomerSDK.presentSupport();
Get how many open conversations the user has
await KustomerSDK.openConversationsCount();
Show Kustomer's Knowledge Base (FAQ) website in a browser modal
KustomerSDK.presentKnowledgeBase();
KustomerSDK.setCurrentPageName(screen);
Customize Kustomer's UI using your own style. For Android, check the Configure section above.
KustomerSDK.customLayout(layout);
The fields email
and phone
are mandatory. custom
can contain any number of custom fields, which can be created from the Kustomer dashboard.
type customerData {
email: String,
phone: String,
custom: {String}
}
All values are String
that contain a HEX color (#FFFFFF
), except for keyboardAppearance
, which should be either set to light
or dark
.
Use the fields for which you want to replace the color, the default color will be used if the field is missing.
type layout {
NavigationBar: {
background: String,
tint: String,
name: String,
greeting: String
},
SessionsTable: {
background: String
},
ChatSessionTableCell: {
background: String,
selectedBackground: String,
title: String,
date: String,
subtitle: String
},
ChatPlaceholderTableCell: {
background: String,
line: String
},
NewSessionButton: {
color: String
},
ChatTable: {
background: String
},
ChatMessageTableCell: {
background: String,
companyText: String,
companyBubble: String,
userBubble: String
},
TypingIndicator: {
color: String
},
InputBar: {
sendButton: String,
tint: String,
placeholder: String,
text: String,
background: String,
keyboardAppearance: 'light' | 'dark'
},
Rating: {
lowScaleLabel: String,
highScaleLabel: String
},
FeedbackTableCell: {
feedbackText: String,
editText: String
},
SatisfactionFormTableCell: {
submitButtonBackground: String,
submitButtonText: String,
commentQuestion: String,
commentBoxBorder: String,
satisfactionQuestion: String,
commentBoxText: String,
introductionQuestion: String
},
Email: {
background: String,
border: String,
prompt: String
},
EndChat: {
background: String,
text: String,
border: String
}
}
FAQs
Kustomer V2 SDK for React Native
The npm package @drivekyte/react-native-kustomer receives a total of 34 weekly downloads. As such, @drivekyte/react-native-kustomer popularity was classified as not popular.
We found that @drivekyte/react-native-kustomer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A survey of 500 cybersecurity pros reveals high pay isn't enough—lack of growth and flexibility is driving attrition and risking organizational security.
Product
Socket, the leader in open source security, is now available on Google Cloud Marketplace for simplified procurement and enhanced protection against supply chain attacks.
Security News
Corepack will be phased out from future Node.js releases following a TSC vote.