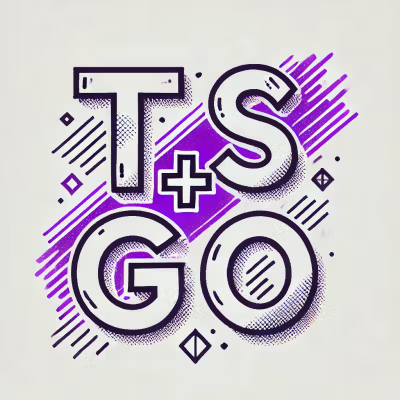
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
@drupal/once
Advanced tools
Mark DOM elements as processed to prevent multiple initializations.
<!-- Use as a module -->
<script type="module">
import once from 'https://unpkg.com/@drupal/once/src/once.js';
const elements = once('my-once-id', 'div');
// Initialize elements.
elements.forEach(el => el.innerHTML = 'processed');
</script>
<!-- Use as a regular script -->
<script src="https://unpkg.com/@drupal/once"></script>
<script>
const elements = once('my-once-id', 'div');
// Initialize elements.
elements.forEach(el => el.innerHTML = 'processed');
</script>
<!-- Using a single element as input-->
<script src="https://unpkg.com/@drupal/once"></script>
<script>
// once methods always return an array, to simplify the use with a single
// element use destructuring or the shift method.
const [myElement] = once('my-once-id', document.body);
const myElement = once('my-once-id', document.body).shift();
</script>
Array.<Element>
Ensures a JavaScript callback is only executed once on a set of elements.
Filters a NodeList or array of elements, removing those already processed
by a callback with a given id.
This method adds a data-once
attribute on DOM elements. The value of
this attribute identifies if a given callback has been executed on that
element.
Kind: global function
Returns: Array.<Element>
- An array of elements that have not yet been processed by a once call
with a given id.
Param | Type | Default | Description |
---|---|---|---|
id | string | The id of the once call. | |
selector | NodeList | Array.<Element> | Element | string | A NodeList or array of elements. | |
[context] | Document | Element | document | An element to use as context for querySelectorAll. |
Example (Basic usage)
const elements = once('my-once-id', '[data-myelement]');
Example (Input parameters accepted)
// NodeList.
once('my-once-id', document.querySelectorAll('[data-myelement]'));
// Array or Array-like of Element.
once('my-once-id', jQuery('[data-myelement]'));
// A CSS selector without a context.
once('my-once-id', '[data-myelement]');
// A CSS selector with a context.
once('my-once-id', '[data-myelement]', document.head);
// Single Element.
once('my-once-id', document.querySelector('#some-id'));
Example (Using a single element)
// Once always returns an array, even when passing a single element. Some
// forms that can be used to keep code readable.
// Destructuring:
const [myElement] = once('my-once-id', document.body);
// By changing the resulting array, es5 compatible.
const myElement = once('my-once-id', document.body).shift();
Array.<Element>
Array.<Element>
Array.<Element>
Array.<Element>
Array.<Element>
Removes a once id from an element's data-drupal-once attribute value.
If a once id is removed from an element's data-drupal-once attribute value, the JavaScript callback associated with that id can be executed on that element again.
Kind: static method of once
Returns: Array.<Element>
- A filtered array of elements that had been processed by the provided id,
and are now able to be processed again.
Param | Type | Default | Description |
---|---|---|---|
id | string | The id of a once call. | |
selector | NodeList | Array.<Element> | Element | string | A NodeList or array of elements to remove the once id from. | |
[context] | Document | Element | document | An element to use as context for querySelectorAll. |
Example (Basic usage)
const elements = once.remove('my-once-id', '[data-myelement]');
Example (Input parameters accepted)
// NodeList.
once.remove('my-once-id', document.querySelectorAll('[data-myelement]'));
// Array or Array-like of Element.
once.remove('my-once-id', jQuery('[data-myelement]'));
// A CSS selector without a context.
once.remove('my-once-id', '[data-myelement]');
// A CSS selector with a context.
once.remove('my-once-id', '[data-myelement]', document.head);
// Single Element.
once.remove('my-once-id', document.querySelector('#some-id'));
Array.<Element>
Finds elements that have been processed by a given once id.
Behaves like once and remove without changing the DOM. To select all DOM nodes processed by a given id, use find.
Kind: static method of once
Returns: Array.<Element>
- A filtered array of elements that have already been processed by the
provided once id.
Param | Type | Default | Description |
---|---|---|---|
id | string | The id of the once call. | |
selector | NodeList | Array.<Element> | Element | string | A NodeList or array of elements to remove the once id from. | |
[context] | Document | Element | document | An element to use as context for querySelectorAll. |
Example (Basic usage)
const filteredElements = once.filter('my-once-id', '[data-myelement]');
Example (Input parameters accepted)
// NodeList.
once.filter('my-once-id', document.querySelectorAll('[data-myelement]'));
// Array or Array-like of Element.
once.filter('my-once-id', jQuery('[data-myelement]'));
// A CSS selector without a context.
once.filter('my-once-id', '[data-myelement]');
// A CSS selector with a context.
once.filter('my-once-id', '[data-myelement]', document.head);
// Single Element.
once.filter('my-once-id', document.querySelector('#some-id'));
Array.<Element>
Finds elements that have been processed by a given once id.
Query the 'context' element for elements that already have the corresponding once id value.
Kind: static method of once
Returns: Array.<Element>
- A filtered array of elements that have already been processed by the
provided once id.
Param | Type | Default | Description |
---|---|---|---|
[id] | string | The id of the once call. | |
[context] | Document | Element | document | Scope of the search for matching elements. |
Example (Basic usage)
const oncedElements = once.find('my-once-id');
Example (Input parameters accepted)
// Call without parameters, return all elements with a `data-once` attribute.
once.find();
// Call without a context.
once.find('my-once-id');
// Call with a context.
once.find('my-once-id', document.head);
FAQs
Act on elements only once.
We found that @drupal/once demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.