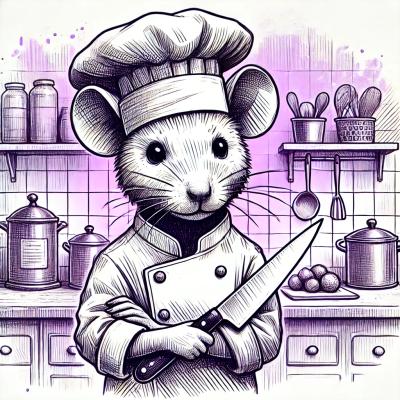
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@entropyxyz/sdk
Advanced tools
@entropyxyz/sdk / Exports
@entropyxyz/sdk
is a collection of TS packages that allow you to interact with the Entropy network. This is currently in alpha release.
This tool is early development. As such, a lot of things do not work. Feel free to play around with it and report any issues at github.com/entropyxyz/sdk.
yarn:
yarn add @entropyxyz/sdk
npm:
npm i @entropyxyz/sdk --save
endpoint
defaults to 'ws://127.0.0.1:9944' if no value is provided.
The main interface to interact with Entropy.
This class provides methods to register, check registration status, interact with programs,
and sign transactions. Users should await the ready
promise to ensure
that the class has been initialized before performing operations.
Below is an example that instantiates Entropy, deploys a program, registers using the deployed program, and signs a transaction.
Example
//store that private key
import { Keyring } from '@entropyxyz/sdk/keys'
import { wasmGlobalsReady, Entropy } from '@entropyxyz/sdk'
await wasmGlobalsReady()
const newSeed = {seed || mnemonic}
const keyring = new Keyring(account)
// you should allways store what comes from this
let persistMe = keyring.accounts.toJson()
const saveToStorage = (state) => persistMe = state
keyring.accounts.on('account-update', (fullAccount) => { saveToStorage(fullAccount) })
let entropy = New Entropy({keyring, endpoint})
// session end
// new session with same account as before
// the second time you use entropy:
const loadedFromStorage = persistMe
const newKeyring = new Keyring(loadFromStorage)
keyring.accounts.on('account-update', (fullAccountAsJSON) => { saveToStorage(fullAccountAsJSON) })
entropy = new Entropy({keyring: newKeyring, endpoint})
• new default(opts
): default
Initializes an instance of the Entropy class.
Name | Type | Description |
---|---|---|
opts | EntropyOpts | The configuration options for the Entropy instance. |
Example
new Entropy({ account: entropyAccount })
await entropy.ready
• Optional
account: EntropyAccount
Example
export interface EntropyAccount {
sigRequestKey?: Signer
programModKey?: Signer | string
programDeployKey?: Signer
verifyingKey?: string
}
Here's an overview of what each field means.
sigRequestKey or 'Signing Key'is the account on the Entropy that is used to initate signing requests
programModKey or 'Program Modification Account' is the account on the Entropy chain which may update the programs for a particular user / organization / entity.
programDeployKey or 'Deploy Key' is the key used to deploy a program that can be used to remove a program
verifyingKey is the key verification key that corresponds to a signer.
For an overview of definitions read: Terminology
• ready: Promise
<boolean
>
A promise that resolves once chacha20poly1305 cryptoLib has been loaded
The ProgramManager provides interfaces to interact with Entropy Programs. It facilitates various operations such as retrieving, setting, adding, and removing programs associated with a user account.
Features Alpha Release: This feature is currently in alpha and is subject to changes. Dev and User Flows: Supports both development and user-level interactions with programs on the Entropy network. Methods constructor Creates an instance of ProgramManager for interacting with Entropy programs.
constructor({
substrate: ApiPromise,
programModKey: Signer,
programDeployKey: Signer,
})
substrate: Instance of ApiPromise from @polkadot/api. programModKey: Signer object for program modification. programDeployKey: Optional signer object for deploying programs.
• programs: default
There are two main flows for interfacing with Entropy Programs: dev and user.
A program can be deployed by anyone without having to register with Entropy first. After being deployed, the program then gets stored in the Programs storage slot with the key being a hash of(bytecode + configuration_interface). The hash is used by a user to point to the programs they want assigned to their key, everytime a program is referenced the ref counter increments.
Here's a deeper overview of programs: programs
Dev Example
// to deploy a program
const pointer = await entropy.programs.dev.deploy('insert program bytecode')
// get a program bytecode
const fetchedProgram = await entropy.programs.dev.get('insert pointer hash')
// to remove a program
await entropy.programs.dev.remove('insert pointer hash')
User Example
// set a program to user list
await this.set(`pointer hash`, sigReqAccount, programModKey)
// get a list of user programs
await entropy.programs.get('user address')
// adds a program a list of user programs
await entropy.programs.add(
'list of program hashes',
sigReqAccount,
programModKey
)
// removes a program a list of user programs
await entropy.programs.remove(
'list of program hashes',
sigReqAccount,
programModKey
)
• registrationManager: default
▸ register(params
): Promise
<void
>
The user registers to Entropy by submitting a transaction from the 'signature request account' containing a 'program modification account', initial 'ProgramsData', and chosen key visibility mode.
ProgramsData - Is multiple Programs Instances. Which contain the program_pointer (the hash of the program you want to use) and the program_config for that program. On the evaluation of a signature request a threshold server will run all the programs and pass through the program config for that program.
The register method Registers an address with Entropy using the provided parameters.
for a more detail overview on registering read: register
Name | Type | Description |
---|---|---|
params | RegistrationParams & { account? : EntropyAccount } | The registration parameters. |
Promise
<void
>
A promise indicating the completion of the registration process.
Throws
Throws
Example
// attempt user registration
await entropy.register({
// insert address to specify ProgramModAccount
initialPrograms: [programData],
programModAccount: '5Gw3s7q9...',
})
• isRegistered: (address
: Address
) => Promise
<boolean
>
▸ (address
): Promise
<boolean
>
Name | Type |
---|---|
address | Address |
Promise
<boolean
>
Example
await entropy.isRegistered(`insert address`)
▸ getVerifyingKey(address
): Promise
<string
>
Retrieves the verifying key associated with the given address's registration record.
Name | Type | Description |
---|---|---|
address | Address | The address for which the verifying key is needed. |
Promise
<string
>
Example
const verifyingKey = await entropy.getVerifyingKey(address)
• signingManager: default
Example
// signing manager
await entropy.signingManager.sign({
sigRequestHash,
hash: this.adapters[type].hash,
type,
})
▸ sign(params
): Promise
<Uint8Array
>
Signs a signature request hash. This method involves various steps including validator selection, transaction request formatting, and submission of these requests to validators for signing. It returns the signature from the first validator after validation.
for a more detailed overview of signing read: signing
Name | Type | Description |
---|---|---|
params | SigOps | The signature operation parameters. |
Promise
<Uint8Array
>
Throws
Example
// sign transaction
await entropy.signTransaction({ txParams: basicTx, type: 'eth' })
▸ signTransaction(params
): Promise
<unknown
>
Signs a given transaction based on the provided parameters.
The signTransaction
method invokes the appropriate adapter (chain based configuration)
based on the type specified in the params
. This modular approach ensures that various
transaction types can be supported. The method performs a series of operations, starting
with the preSign
function of the selected adapter, followed by the actual signing of the
transaction request hash, and if necessary, the postSign
function of the adapter.
Name | Type | Description |
---|---|---|
params | SigTxOps | The parameters for signing the transaction. |
Promise
<unknown
>
A promise that returns the transaction signature. Note that the structure and format of this signature may differ based on the adapter.
Throws
Throws
Will throw an error if the transaction type does not have a corresponding adapter.
Example
// signing manager
await entropy.signingManager.sign({
sigRequestHash,
hash: this.adapters[type].hash,
type,
})
• substrate: ApiPromise
Example
// a simple get balance for user
const accountInfo = await entropy.substrate.query.system.account(address)
const freeBalance = hexToBigInt(accountInfo.data.free)
console.log(
`Address ${
selectedAccount.address
} has a balance of: ${freeBalance.toString()} bits`
)
Need help with something? Head over to the Entropy Community repository for support or to raise a ticket →
FAQs
JS SDK for entropy blockchain
The npm package @entropyxyz/sdk receives a total of 9 weekly downloads. As such, @entropyxyz/sdk popularity was classified as not popular.
We found that @entropyxyz/sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.