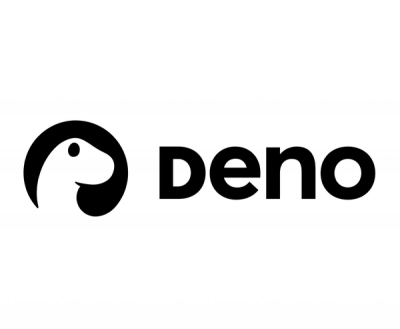
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@eroc/core
Advanced tools
core is a concept introduced by Nicholas C. Zakas in this video
It helps you create scalable applications written in Javascript, giving you some structure and patterns to keep everything separated.
Conceptually, everything in your application is a module, and your modules should work independently from each other, so if one module breaks, the others should not.
A module should never talk directly to another module, for that you use a combination of listeners and notifications.
So let's think about the twitter page, and how we could re-build it using the core concept
Everything inside a red square is a module, they work in a way that they don't depend on any other modules, and they should be programmed that way as well.
npm i @eroc/core
Raw import
import {Core} from "./node_modules/@eroc/core/dist/core.js";
With rollup, webpack or parcel
import {Core} from "@eroc/core";
NodeJs or Browserify
const {Core} = require("@eroc/core");
Let's start with the tweet module.
Core.register('tweet', function(sandbox) {
return {
init: function() {
console.log('starting tweet module');
}
}
});
This way you can register a new module, and the method init
will be called once the module is started, if it exists.
Core.start('tweet'); // Log: starting tweet module
For every module you have an enviroment called sandbox, this is the only guy your module will ever speak to.
It garantees that if your module tries to call something that doesn't exists or is broken, it won't break the module itself.
Now let's think about the tweet list, which fetches all tweets from your timeline.
Core.register('tweet-list', function(sandbox) {
return {
init: function() {
}
}
});
If you have multiple modules you can start everything using Core.start();
with no parameters, and everything will be started. But if you need some specific order for starting your modules, you can call the modules you want first, and then use Core.start()
.
You might want to stop a module in some point, this can be easily done using the method Core.stop()
.
Core.register('tweet-list', function(sandbox) {
return {
destroy: function() {
console.log('Module destroyed');
}
}
});
Core.start('tweet-list');
Core.stop('tweet-list'); // Log: Module destroyed
When you stop a module, the method destroy
will be called, if it exists.
Now, thinking about Twitter, everytime you tweet something, it should appear on your tweet list right? but since our modules don't talk directly to each other, let's use sandbox
to do this for us:
First of all, our tweet
module should notify other modules that something has happened.
Core.register('tweet', function(sandbox) {
return {
init: function(sandbox) {
// For the sake of simplicity, I'm gonna use a interval to submit tweets
setInterval(function() {
sandbox.notify({
type: 'new-tweet',
data: {
author: 'Mauricio Soares',
text: 'core is pretty #cool'
}
})
}, 5 * 1000)
}
}
});
Every 5 seconds, this module notifies everything that is listening to new-tweet
that something has happened. If nothing is listening to it, than nothing happens.
Our tweet-list
is going to listen for this notifications.
Core.register('tweet-list', function(sandbox) {
return {
init: function() {
sandbox.listen('new-tweet', this.newTweet);
},
newTweet: function(data) {
// does something with data
}
}
});
Cool right? If one of those modules stop working, than it won't break the other one!
If you have a DOM element with the same ID as the module name, it will be accessible inside the module using this.el
.
<div id="tweet"></div>
Core.register('tweet', function() {
return {
init: function() {
console.log(this.el);
}
}
});
Core.start('tweet'); // Log: <div id="tweet"></div> (DOM Reference)
If there's no DOM element, then this.el
will return null
.
// lets suppose we have jquery loaded before this
Core.register('tweet', function(sandbox) {
return {
init: function() {
jQuery('#tweet').on('click', this.newTweet);
},
newTweet: function() {
// handles click
}
};
});
A module should not talk to other modules directly anything else but the sandbox
.
This is basically how core works, below you will find the documentation of methods and parameters.
Register a new module.
moduleName
(string): The name of the moduleconstructor
(function): The implementation of the moduleUsage
Core.register('module', function() {})
Starts the named module. If a value is returned in the init
method, it can be grabbed in the return of the method Core.start
. If no parameters are passed, it starts all unstarted modules.
moduleName
(string): The name of the moduleUsage
Core.start('module');
Stops the named module. If a value is returned in the destroy
method, it can be grabbed in the return of the method Core.stop
, If no parameters are passed, it stop all modules.
moduleName
(string): The name of the moduleUsage
Core.stop('module');
Listens to other modules notifications, to overwrite a notification you must use the parameter force
notification
(string | array): The name of the notification you are listening tocallback
(function): The callback when the notification is triggeredcontext
(object): The value of this
inside the callbackforce
(boolean): If you want to overwrite a notification, use true
hereNotifies other modules
config
(object): The configuration object
type
(string): The notification that will be triggereddata
(function | string | number | boolean | array): The data that will be passed in the callbackgit checkout -b my_branch
git push origin my_branch
Please respect the indentation rules and code style.
Use 2 spaces, not tabs.
New features? Would you mind testing it? :)
You need NodeJS installed on your machine
npm i
npm run bundle
npm t
Core.stopAll
Core.startAll
x
to use
in Sandbox
(The MIT License)
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the 'Software'), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED 'AS IS', WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
FAQs
Lightweight framework for scalable applications
We found that @eroc/core demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.