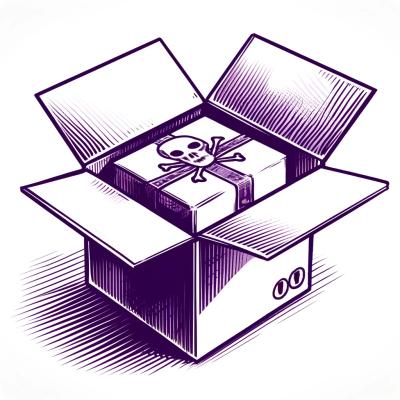
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@eslint-community/eslint-utils
Advanced tools
@eslint-community/eslint-utils is a utility library for working with ESLint. It provides a set of tools to help with creating, testing, and debugging ESLint rules and plugins.
getStaticValue
The `getStaticValue` function evaluates the static value of a given AST node. This is useful for determining the value of constants and literals in the code.
const { getStaticValue } = require('@eslint-community/eslint-utils');
const espree = require('espree');
const ast = espree.parse('const foo = 42;', { ecmaVersion: 2020 });
const node = ast.body[0].declarations[0].init;
const value = getStaticValue(node);
console.log(value); // { value: 42 }
ReferenceTracker
The `ReferenceTracker` class helps track references to global variables and properties. This is useful for identifying where and how certain variables or properties are used in the code.
const { ReferenceTracker } = require('@eslint-community/eslint-utils');
const espree = require('espree');
const eslintScope = require('eslint-scope');
const ast = espree.parse('const foo = { bar: 42 }; foo.bar;', { ecmaVersion: 2020 });
const scopeManager = eslintScope.analyze(ast);
const tracker = new ReferenceTracker(scopeManager);
for (const { node } of tracker.iterateGlobalReferences({ foo: { bar: true } })) {
console.log(node); // Node representing `foo.bar`
}
getFunctionHeadLocation
The `getFunctionHeadLocation` function returns the location of the function head, which includes the function keyword, name, and parameters. This is useful for reporting issues related to function definitions.
const { getFunctionHeadLocation } = require('@eslint-community/eslint-utils');
const espree = require('espree');
const ast = espree.parse('function foo() {}', { ecmaVersion: 2020 });
const node = ast.body[0];
const location = getFunctionHeadLocation(node, { sourceCode: { text: 'function foo() {}' } });
console.log(location); // { start: { line: 1, column: 0 }, end: { line: 1, column: 13 } }
ESLint is a tool for identifying and reporting on patterns found in ECMAScript/JavaScript code. It is highly configurable and can be extended with custom rules and plugins. While @eslint-community/eslint-utils provides utilities for working with ESLint, ESLint itself is the core tool for linting JavaScript code.
eslint-plugin-import is a plugin that provides linting rules for validating proper imports. It helps ensure that import statements are valid and follow best practices. While @eslint-community/eslint-utils provides general utilities for ESLint, eslint-plugin-import focuses specifically on import/export syntax.
eslint-plugin-react is a plugin that provides linting rules for React applications. It helps enforce best practices and catch common issues in React code. While @eslint-community/eslint-utils provides general utilities for ESLint, eslint-plugin-react focuses specifically on React.
This package provides utility functions and classes for make ESLint custom rules.
For examples:
getStaticValue
evaluates static value on AST.ReferenceTracker
checks the members of modules/globals as handling assignments and destructuring.See documentation.
See releases.
Welcome contributing!
Please use GitHub's Issues/PRs.
npm test
runs tests and measures coverage.npm run clean
removes the coverage result of npm test
command.npm run coverage
shows the coverage result of the last npm test
command.npm run lint
runs ESLint.npm run watch
runs tests on each file change.FAQs
Utilities for ESLint plugins.
The npm package @eslint-community/eslint-utils receives a total of 24,346,481 weekly downloads. As such, @eslint-community/eslint-utils popularity was classified as popular.
We found that @eslint-community/eslint-utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.