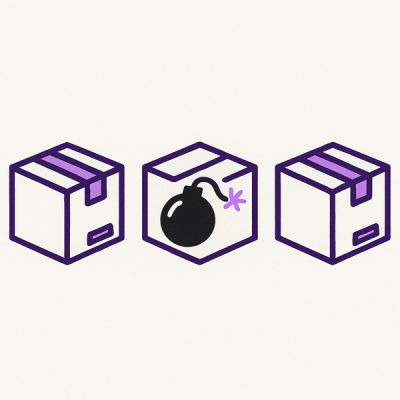
Research
Security News
The Landscape of Malicious Open Source Packages: 2025 Mid‑Year Threat Report
A look at the top trends in how threat actors are weaponizing open source packages to deliver malware and persist across the software supply chain.
@figliolia/data-structures
Advanced tools
Type-safe and efficient data-structures for everyday programming.
In this library you'll find a few of the common data-structures I find myself creating over and over again across my projects. When using this library you'll have access to:
All of which are documented and type-safe.
npm i @figliolia/data-structures
# or
yarn add @figliolia/data-structures
A generic graph construct for string and number values
import { Graph, NodeCache } from "@figliolia/data-structures";
const cache = new NodeCache();
const root = cache.create(1);
const node2 = cache.create(2);
root.addEdge(node2);
root.addEdge(cache.create(4));
node2.addEdge(cache.create(3));
root.DFS(node => console.log(node.value)); // 1, 2, 3, 4
root.BFS(node => console.log(node.value)); // 1, 2, 4, 3
A doubly linked list mimicking the interface of JavaScript arrays
import { LinkedList } from "@figliolia/data-structures";
const list = new LinkedList<number>();
list.push(1);
list.push(2);
list.push(3);
for(const item of list) {
console.log(item); // 1, 2, 3
}
list.pop(); // 3 -> O(1)
list.shift() // 1 -> O(1)
list.push(3) // O(1)
list.unshift(1) // O(1)
A heap that remains sorted descendingly
import { MaxHeap } from "@figliolia/data-structures";
const heap = new MaxHeap<number>(value => value);
heap.push(3);
heap.push(2);
heap.push(1);
heap.pop() // 3
const complexDataHeap = new MaxHeap<{ id: number, name: string }>(value => value.id);
complexDataHeap.push({ id: 3, name: "Jeff" });
complexDataHeap.push({ id: 2, name: "Steve" });
complexDataHeap.push({ id: 1, name: "Dave" });
complexDataHeap.pop() // { id: 3, name: "Jeff" }
A heap that remains sorted ascendingly
import { MinHeap } from "@figliolia/data-structures";
const heap = new MinHeap<number>(value => value);
heap.push(1);
heap.push(2);
heap.push(3);
heap.pop() // 1
const complexHeap = new MinHeap<{ id: number, name: string }>(
value => value.id // numeric value extractor
);
complexHeap.push({ id: 1, name: "Jeff" });
complexHeap.push({ id: 2, name: "Steve" });
complexHeap.push({ id: 3, name: "Dave" });
complexHeap.pop() // { id: 1, name: "Jeff" }
A stack maintaining a reference to it's highest weighted item
import { MaxStack } from "@figliolia/data-structures";
const stack = new MaxStack<number>(value => value);
stack.push(1); // max = 1
stack.push(2); // max = 2
stack.push(3); // max = 3
stack.max // 3
stack.pop() // max = 2
A stack maintaining a reference to it's lowest weighted item
import { MinStack } from "@figliolia/data-structures";
const stack = new MinStack<number>(value => value);
stack.push(3); // min = 3
stack.push(2); // min = 2
stack.push(1); // min = 1
stack.min // 1
stack.pop() // min = 2
A bucket queue that sorts elements based on the priority level specified
import { PriorityQueue } from "@figliolia/data-structures";
const queue = new PriorityQueue<number>();
queue.push(1 /* priority */, 3 /* value */);
queue.push(2, 2);
queue.push(3, 1);
queue.length // 3
// queue = [[3], [2], [1]]
while(!queue.isEmpty) {
queue.pop() // 3, 2, 1
}
A basic queue with enqueue, dequeue and peek methods
import { Queue } from "@figliolia/data-structures";
const queue = new Queue<number>();
queue.enqueue(1);
queue.enqueue(2);
queue.enqueue(3);
queue.peek(); // 1
queue.dequeue(); // 1
A basic stack with push, pop and peek methods
import { Stack } from "@figliolia/data-structures";
const stack = new Stack<number>();
stack.push(1);
stack.push(2);
stack.push(3);
stack.peek(); // 3
stack.pop(); // 3
A graph-like data structure for optimized search over multiple strings
import { Trie } from "@figliolia/data-structures";
const dictionary = new Trie();
dictionary.add("hello");
dictionary.add("goodbye");
dictionary.add("helpful");
dictionary.search("hello"); // true
dictionary.search("help", false); // true
A wrapper around the native JavaScript Map that assigns an auto-incrementing ID to each value added. It provides a stack-like interface with the ability to access and remove items in 0(1) time
import { QuickStack } from "@figliolia/data-structures";
const stack = new QuickStack<() => void>();
const ID1 = stack.push(function one() {});
const ID2 = stack.push(function two() {});
stack.pop() // function two() {}
stack.pop() // function one() {}
stack.get(/* ID */) // retrieves an item by ID 0(1)
stack.delete(/* ID */) // deletes an item by ID 0(1)
A wrapper around the native JavaScript Map that assigns an auto-incrementing ID to each value added. It provides a queue-like interface with the ability to access and remove items in 0(1) time
import { QuickQueue } from "@figliolia/data-structures";
const queue = new QuickQueue<() => void>();
const ID1 = queue.enqueue(function one() {});
const ID2 = queue.enqueue(function two() {});
queue.dequeue() // function one() {}
queue.dequeue() // function two() {}
queue.get(/* ID */) // retrieves an item by ID 0(1)
queue.delete(/* ID */) // deletes an item by ID 0(1)
Logarithmic searching for sorted lists
import { binarySearch } from "@figliolia/data-structures";
binarySearch([1, 2, 3, 4], 3) // true
binarySearch([1, 2, 3, 4], 5) // false
binarySearch(
[
{ id: 1, name: "Jeff" },
{ id: 2, name: "Steve" },
{ id: 3, name: "Dave" },
{ id: 4, name: "Alex" },
],
{ id: 3, name: "Dave" },
item => item.id
) // true
FAQs
Efficient data structures for every day programming
The npm package @figliolia/data-structures receives a total of 12 weekly downloads. As such, @figliolia/data-structures popularity was classified as not popular.
We found that @figliolia/data-structures demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A look at the top trends in how threat actors are weaponizing open source packages to deliver malware and persist across the software supply chain.
Security News
ESLint now supports HTML linting with 48 new rules, expanding its language plugin system to cover more of the modern web development stack.
Security News
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.