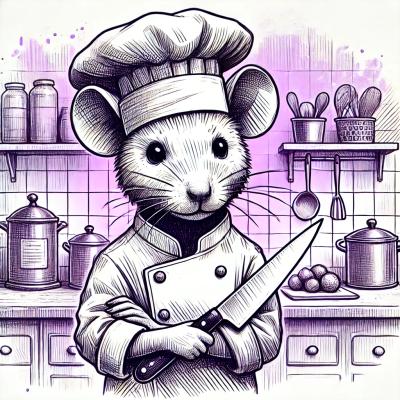
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@firebase/component
Advanced tools
The @firebase/component package is a part of the Firebase platform which provides a lightweight, service-oriented architecture to manage the dependencies between the different Firebase components. It allows developers to register and retrieve Firebase services dynamically, ensuring that each component can function independently while still being able to interact with other components in a scalable manner.
Service Registration
This feature allows developers to register a new service within the Firebase ecosystem. The code sample demonstrates how to create and register a 'logger' service that can be reused across different parts of an application.
{
import { Component, ComponentType } from '@firebase/component';
import { Logger } from './logger';
const loggerComponent = new Component('logger', () => new Logger(), ComponentType.PUBLIC);
firebase.registerComponent(loggerComponent);
}
Service Retrieval
This feature enables the retrieval of registered services. The code sample shows how to retrieve an instance of the previously registered 'logger' service, allowing it to be used within the application.
{
import { getProvider } from '@firebase/component';
const loggerProvider = getProvider(app, 'logger');
const loggerInstance = loggerProvider.getImmediate();
}
Inversify is an inversion of control library that uses TypeScript decorators to manage dependencies. It is similar to @firebase/component in that it provides dependency injection, but it is more focused on TypeScript support and uses a different syntax and methodology for registering and resolving dependencies.
BottleJS is a minimalistic dependency injection micro container. Like @firebase/component, it allows the registration and retrieval of services. However, BottleJS is not specific to Firebase and can be used in any JavaScript application, providing a more general-purpose approach compared to the Firebase-specific @firebase/component.
NOTE: This is specifically tailored for Firebase JS SDK usage, if you are not a member of the Firebase team, please avoid using this package
ES Modules
import { Component } from '@firebase/component';
FAQs
Firebase Component Platform
The npm package @firebase/component receives a total of 3,631,829 weekly downloads. As such, @firebase/component popularity was classified as popular.
We found that @firebase/component demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.