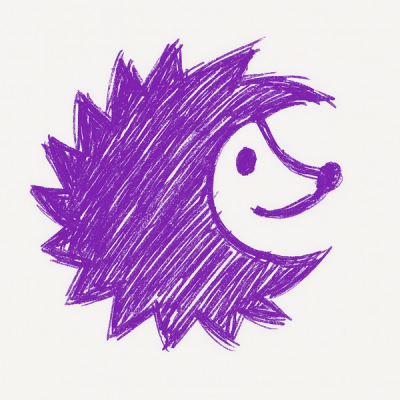
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
@flatfile/plugin-record-hook
Advanced tools
A plugin for writing custom record-level hooks in Flatfile.
This plugin provides a concise syntax for running custom logic on individual data records in Flatfile.
npm i @flatfile/plugin-record-hook @flatfile/hooks
import { recordHook } from '@flatfile/plugin-record-hook'
import type { FlatfileRecord } from '@flatfile/hooks'
Pass recordHook
to a Flatfile data listener and provide a function to run when data is added or updated.
Set up a listener to configure Flatfile and respond to data events:
import {
Client,
FlatfileVirtualMachine,
FlatfileEvent,
} from '@flatfile/listener'
const listener = Client.create((client) => {
// Set up your configuration here
})
const FlatfileVM = new FlatfileVirtualMachine()
listener.mount(FlatfileVM)
export default listener
Use the this plugin to set up a hook that responds to data changes:
import { recordHook } from '@flatfile/plugin-record-hook'
listener.use(
recordHook('my-sheet', (record: FlatfileRecord) => {
// Your logic goes here
})
)
Replace my-sheet
with the slug of the Sheet you want to attach this hook to.
Create a record hook that populates a "full name" field based on the values of first name and last name:
listener.use(
recordHook('my-sheet', (record: FlatfileRecord) => {
const firstName = record.get('firstName')
const lastName = record.get('lastName')
if (firstName && lastName && !record.get('fullName')) {
record.set('fullName', `${firstName} ${lastName}`)
record.addComment(
'fullName',
'Full name was populated from first and last name.'
)
}
return record
})
)
Use helper functions from @flatfile/plugin-transform in your record hooks for cleaner syntax.
FAQs
A plugin for running custom logic on individual data records in Flatfile.
We found that @flatfile/plugin-record-hook demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 14 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.