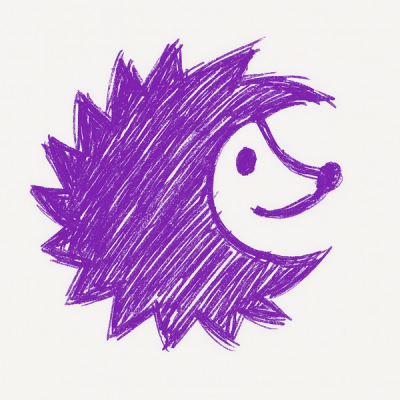
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
@functionalities/formhandler
Advanced tools
A frontend utility package for handling forms
Form Handler is a frontend library made to make input validation in forms easier. It handles submissions, error handling, flash messages, and recaptcha with Clourflares Turnstile and Google Recaptcha V2.
npm install @functionalities/formhandler --save
import FormHandler from "@functionalities/formhandler";
new FormHandler("#form");
<form id="form" action="http://localhost:4646/form" method="post">
<div data-input-relation="name">
<input type="text" placeholder="Name" name="name" required />
<div class="error-container">
<p data-input-error="name"></p>
</div>
</div>
<div data-input-relation="email">
<input type="email" placeholder="Email Address" name="email" required />
<div class="error-container">
<p data-input-error="email"></p>
</div>
</div>
<input type="submit" value="Submit" class="submit" />
</form>
data-input-relation
If this attribute is present on an element, whenever the input with the same name fails, the error class will get applied to it.
data-input-error
An element with this attribute will have its innerHTML set to the first error message that corresponds to the input on validation failing.
We currently support Cloudflare's Turnstile and Google Recaptcha V2 for our Recaptcha. These will append the corresponding script to the site and handle loading and refreshing Recaptcha tokens.
This is an optional module.
Import and initialise the Turnstile class and pass it to your FormHandler instance. This requires one parameter which is the site key.
import FormHandler, { Turnstile } from "@functionalities/formhandler";
new FormHandler("#form", {
recaptcha: new Turnstile("site_key")
});
This will be accessible on the backend on the body through the
cf-turnstile-response
key.
Import and initialise the GoogleV2 class and pass it to your FormHandler instance. This requires one parameter which is the site key.
import FormHandler, { GoogleV2 } from "@functionalities/formhandler";
new FormHandler("#form", {
recaptcha: new GoogleV2("site_key")
});
This will be accessible on the backend of the body through the
g-recaptcha-response
key.
An optional module that allows messages to be toggled on the DOM. It is up to you to style.
import FormHandler, { FlashMessage } from "@functionalities/formhandler";
new FormHandler("#form", {
flashMessage: new FlashMessage("#flash-message", 5000)
});
<div id="flash-message">
<p data-flash-message-message></p>
<button data-flash-message-close>Close</button>
</div>
data-flash-message-close
Any element with this attribute that resides in the flash message container, will have a click event set that will close the popup.
data-flash-message-message
One element should have this attribute set. The innerHTML of this element will be set to the message.
is-active
will be set on the container.is-success
set on it.is-error
set on it.Here is the config typescript interface:
interface Config {
recaptcha?: Turnstile | GoogleV2;
resetOnSuccess?: boolean;
flashMessage?: FlashMessage;
disableSubmit?: boolean;
validate?: {
onChange?: boolean;
onSubmit?: boolean;
};
localisation?: {
validationError?: string;
error?: string;
success?: string;
};
errorClass?: string;
action?: string;
customValidation?: Array<CustomValidation>;
attributes?: {
inputRelation?: string;
inputError?: string;
};
onSuccess?: (form: HTMLFormElement, res: any) => void;
onError?: (form: HTMLFormElement, res: any) => void;
}
This has been covered here.
default: undefined
If enabled the form will reset on success.
default: true
This has been covered here.
default: undefined
If enabled this will set the disabled attribute on all of the form submit inputs when it is loading.
default: true
default: both set to true
The default messages are passed to the FlashMessage instance.
The default error is appended to all invalid inputs, input relations, and input error elements.
default: 'error'
This is the URL used on the POST request. It defaults to the action attribute set on the form element, but if not present it will either use this or an empty string.
default: the forms action value or an empty string
This allows you to define custom validation beyond what the browser has built in. Here is an example:
import FormHandler from "@functionalities/formhandler";
new FormHandler("#form", {
customValidation: [
{
name: "firstName", // this is your inputs name
validator: (value) => {
if (value === "John") return ""; // '' represents no error
else return "Validation failed. First name is not equal to 'John'."; // this is your error
},
},
],
});
This allows you to update the default attributes used to define an input relation and an input error element.
On successful submission, this callback is fired. It receives the form and the response from the POST request as its parameters. You can use this to extend some functionality.
default: undefined
On a failed submission this callback is fired. It receives the form and the response from the POST request as its parameters. You can use this to extend some functionality.
default: undefined
FAQs
A frontend utility package for handling forms
The npm package @functionalities/formhandler receives a total of 0 weekly downloads. As such, @functionalities/formhandler popularity was classified as not popular.
We found that @functionalities/formhandler demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.