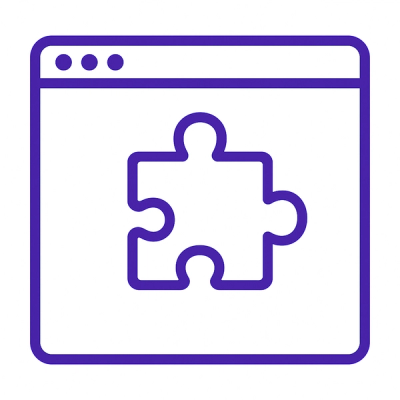
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
@generouted/react-router
Advanced tools
Check out generouted
's main docs for the features, conventions and more.
This integration is based on a Vite plugin to generate routes types for React Router with generouted
conventions. The output is saved by default at src/router.ts
and gets updated by the add/change/delete at src/pages/*
.
In case you don't have a Vite project with React and TypeScript, check Vite documentation to start a new project.
pnpm add @generouted/react-router react-router
// vite.config.ts
import { defineConfig } from 'vite'
import react from '@vitejs/plugin-react'
import generouted from '@generouted/react-router/plugin'
export default defineConfig({ plugins: [react(), generouted()] })
// src/main.tsx
import { createRoot } from 'react-dom/client'
import { Routes } from '@generouted/react-router'
// import { Routes } from '@generouted/react-router/lazy' // route-based code-splitting
createRoot(document.getElementById('root')!).render(<Routes />)
Add the home page by creating a new file src/pages/index.tsx
→ /
, then export a default component:
// src/pages/index.tsx
export default function Home() {
return <h1>Home</h1>
}
pages/_app.tsx
// src/pages/_app.tsx
import { Outlet } from 'react-router'
export default function App() {
return (
<section>
<header>
<nav>...</nav>
</header>
<main>
<Outlet />
</main>
</section>
)
}
Autocompletion for Link
, useNavigate
, useParams
and more exported from src/router.ts
// src/pages/index.tsx
import { Link, useNavigate, useParams } from '../router'
export default function Home() {
const navigate = useNavigate()
// typeof params -> { id: string; pid?: string }
const params = useParams('/posts/:id/:pid?')
// typeof params to be passed -> { id: string; pid?: string }
const handler = () => navigate('/posts/:id/:pid?', { params: { id: '1', pid: '0' } })
return (
<div>
{/** ✅ Passes */}
<Link to="/" />
<Link to="/posts/:id" params={{ id: '1' }} />
<Link to="/posts/:id/:pid?" params={{ id: '1' }} />
<Link to="/posts/:id/:pid?" params={{ id: '1', pid: 0 }} />
{/** 🔴 Error: not defined route */}
<Link to="/not-defined-route" />
{/** 🔴 Error: missing required params */}
<Link to="/posts/:id" />
<h1>Home</h1>
</div>
)
}
Although all modals are global, it's nice to co-locate modals with relevant routes.
Create modal routes by prefixing a valid route file name with a plus sign +
. Why +
? You can think of it as an extra route, as the modal overlays the current route:
// src/pages/+login.tsx
import { Modal } from '@/ui'
export default function Login() {
return <Modal>Content</Modal>
}
To navigate to a modal use useModals
hook exported from src/router.ts
:
// src/pages/_app.tsx
import { Outlet } from 'react-router'
import { useModals } from '../router'
export default function App() {
const modals = useModals()
return (
<section>
<header>
<nav>...</nav>
<button onClick={() => modals.open('/login')}>Open modal</button>
</header>
<main>
<Outlet />
</main>
</section>
)
}
With useModals
you can use modals.open('/modal-path')
and modals.close()
, and by default it opens/closes the modal on the current active route.
Both methods come with React Router's navigate()
options with one prop added at
, for optionally navigating to a route while opening/closing a modal, and it's also type-safe!
modals.open(path, options)
modals.close(options)
at
should be also a valid route path, here are some usage examples:
modals.open('/login', { at: '/auth', replace: true })
modals.open('/info', { at: '/invoice/:id', params: { id: 'xyz' } })
modals.close({ at: '/', replace: false })
MIT
FAQs
Generated file-based routes for React Router and Vite
The npm package @generouted/react-router receives a total of 34,214 weekly downloads. As such, @generouted/react-router popularity was classified as popular.
We found that @generouted/react-router demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.