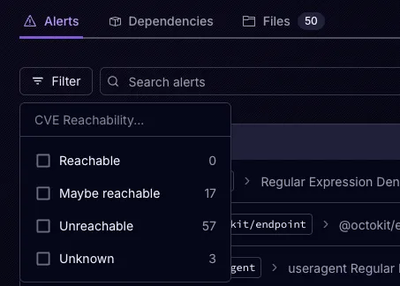
Product
Announcing Precomputed Reachability Analysis in Socket
Socket’s precomputed reachability slashes false positives by flagging up to 80% of vulnerabilities as irrelevant, with no setup and instant results.
@geprog/node-migrate-ts
Advanced tools
Abstract migration framework using TypeScript.
A migration is a simple Migration
object containing a unique id
and implementations for up
and down
.
If necessary up
and down
can be async.
// exampleMigration.ts
import { Migration } from '@geprog/node-migrate-ts';
export const exampleMigration: Migration = {
id: 'exampleMigration', // unique migration identifier
up() {
console.log('Do some migrations in here');
},
down() {
console.log('Revert your migration in here');
},
};
The state of migrations can be stored anywhere by passing a MigrationStore
implementation to up
Currently implemented MigrationStore
s are:
Contributions are very welcome! :wink:
This example uses MongoMigrationStore
as MigrationStore
.
import { Migration, MongoMigrationStore, up } from '@geprog/node-migrate-ts';
import { MongoClient } from 'mongodb';
import { exampleMigration } from './exampleMigration';
// migrations are applied in the order defined here
const migrations: Migration[] = [exampleMigration];
const client = await MongoClient.connect('mongodb://user:password@localhost:27017/database?authSource=admin', {
useUnifiedTopology: true,
});
const db = client.db();
const migrationStore = new MongoMigrationStore();
migrationStore.init({
db,
migrationsCollection: 'migrations',
});
await up({ migrations, migrationStore });
await client.close();
To avoid a lot of boilerplate in the migrations, a context can be passed to up.
This context will then be available in the migrations up
and down
functions.
To clarify, here is a modification of the above example to pass the database connection to all migrations:
import { Migration, MongoMigrationStore, up } from '@geprog/node-migrate-ts';
-import { MongoClient } from 'mongodb';
+import { Db, MongoClient } from 'mongodb';
import { exampleMigration } from './exampleMigration';
+declare module '@geprog/node-migrate-ts' {
+ interface MigrationContext {
+ db: Db;
+ }
+}
+
// migrations are applied in the order defined here
const migrations: Migration[] = [exampleMigration];
const client = await MongoClient.connect('mongodb://user:password@localhost:27017/database?authSource=admin', {
useUnifiedTopology: true,
});
const db = client.db();
const migrationStore = new MongoMigrationStore();
migrationStore.init({
db,
migrationsCollection: 'migrations',
});
-await up({ migrations, migrationStore });
+await up({ migrations, migrationStore, context: { db } });
await client.close();
The migrations can now use the database connection from the context.
import { Migration } from '@geprog/node-migrate-ts';
import { ObjectId } from 'mongodb';
export const exampleMigration: Migration = {
id: 'exampleMigration',
async up(context) {
if (!context || !context.db) {
throw new Error('Please pass a context with a db object');
}
const { db } = context;
console.log('Migrate some mongodb here');
},
async down(context) {
if (!context || !context.db) {
throw new Error('Please pass a context with a db object');
}
const { db } = context;
console.log('Migrate some mongodb here');
},
};
This project is based on:
FAQs
Abstract migration framework using TypeScript
The npm package @geprog/node-migrate-ts receives a total of 3 weekly downloads. As such, @geprog/node-migrate-ts popularity was classified as not popular.
We found that @geprog/node-migrate-ts demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket’s precomputed reachability slashes false positives by flagging up to 80% of vulnerabilities as irrelevant, with no setup and instant results.
Product
Socket is launching experimental protection for Chrome extensions, scanning for malware and risky permissions to prevent silent supply chain attacks.
Product
Add secure dependency scanning to Claude Desktop with Socket MCP, a one-click extension that keeps your coding conversations safe from malicious packages.