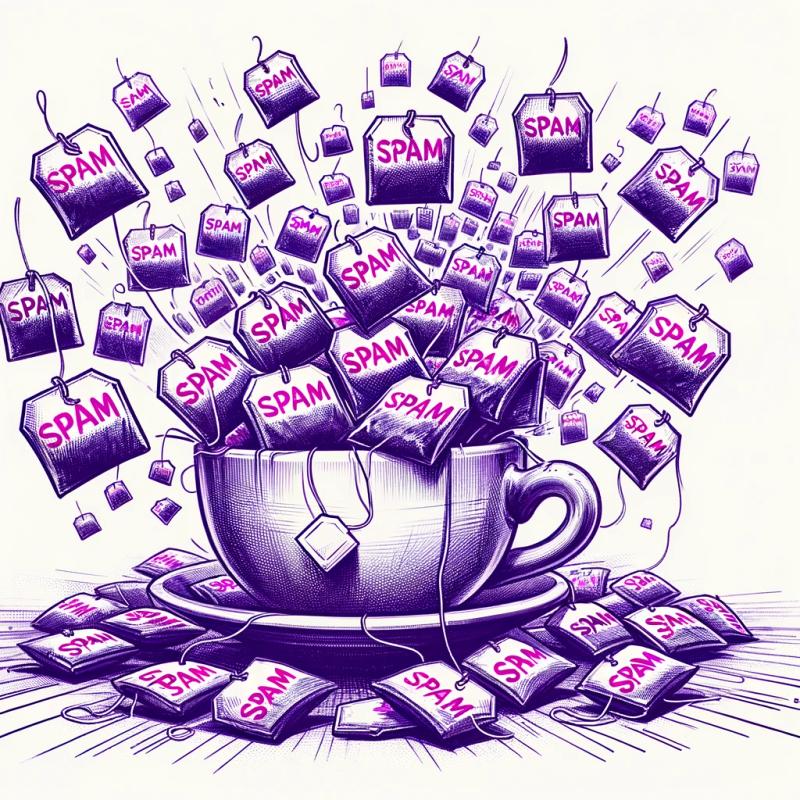
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@gofunky/trumpet
Advanced tools
Readme
the maintained version of trumpet
<table>
<tbody>blah blah blah</tbody>
<tr><td>there</td></tr>
<tr><td>it</td></tr>
<tr><td>is</td></tr>
</table>
const trumpet = require('@gofunky/trumpet')
const tr = trumpet()
tr.pipe(process.stdout)
const ws = tr.select('tbody').createWriteStream()
ws.end('<tr><td>rawr</td></tr>')
const fs = require('fs')
fs.createReadStream(__dirname + '/html/table.html').pipe(tr)
<table>
<tbody><tr><td>rawr</td></tr></tbody>
<tr><td>there</td></tr>
<tr><td>it</td></tr>
<tr><td>is</td></tr>
</table>
<html>
<head>
<title>beep</title>
</head>
<body>
<div class="a">¡¡¡</div>
<div class="b">
<span>tacos</span>
<span> y </span>
<span>burritos</span>
</div>
<div class="a">!!!</div>
</body>
</html>
const trumpet = require('@gofunky/trumpet')
const tr = trumpet()
tr.selectAll('.b span', (span) => {
span.createReadStream().pipe(process.stdout)
})
const fs = require('fs')
fs.createReadStream(__dirname + '/html/read_all.html').pipe(tr)
tacos y burritos
<html>
<body>
<div class="x">
<span>hack</span>
<span> the </span>
<span>planet</span>
</div>
</body>
</html>
const trumpet = require('@gofunky/trumpet')
const through = require('through2')
const tr = trumpet()
//select all element and apply transformation function to selections
tr.selectAll('.x span', (element) => {
//define function to transform input
const upper = through((buf) => {
this.queue(String(buf).toUpperCase())
})
//create a read/write stream for selected selement
const stream = element.createStream()
//stream the element's inner html to transformation function
//then stream the transformed output back into the element stream
stream.pipe(upper).pipe(stream)
//stream in html to trumpet and stream processed output to stdout
const fs = require('fs')
fs.createReadStream(__dirname + '/html/uppercase.html').pipe(tr).pipe(process.stdout)
<html>
<body>
<div class="x">
<span>HACK</span>
<span> THE </span>
<span>PLANET</span>
</div>
</body>
</html>
const trumpet = require('@gofunky/trumpet')
const tr = trumpet(opts)
Create a new trumpet stream. This stream is readable and writable.
Pipe an html stream into tr
and get back a transformed html stream.
Parse errors are emitted by tr
in an 'error'
event.
const elem = tr.select(selector)
Return a result object elem
for the first element matching selector
.
tr.selectAll(selector, (elem) => {})
Get a result object elem
for every element matching selector
.
elem.getAttribute(name, cb)
When the selector for elem
matches, query the case-insensitive attribute
called name
with cb(value)
.
Returns elem
.
elem.getAttributes(name, cb)
Get all the elements in cb(attributes)
as an object attributes
with
lower-case keys.
Returns elem
.
elem.setAttribute(name, value)
When the selector for elem
matches, replace the case-insensitive attribute
called name
with value
.
If the attribute doesn't exist, it will be created in the output stream.
Returns elem
.
elem.removeAttribute(name)
When the selector for elem
matches, remove the attribute called name
if it
exists.
Returns elem
.
elem.createReadStream(opts)
Create a new readable stream with the inner html content under elem
.
To use the outer html content instead of the inner, set opts.outer
to true
.
elem.createWriteStream(opts)
Create a new write stream to replace the inner html content under elem
.
To use the outer html content instead of the inner, set opts.outer
to true
.
elem.createStream(opts)
Create a new readable writable stream that outputs the content under elem
and
replaces the content with the data written to it.
To use the outer html content instead of the inner, set opts.outer
to true
.
tr.createStream(sel, opts)
Short-hand for tr.select(sel).createStream(opts)
.
tr.createReadStream(sel, opts)
Short-hand for tr.select(sel).createReadStream(opts)
.
tr.createWriteStream(sel, opts)
Short-hand for tr.select(sel).createWriteStream(opts)
.
elem.name
The element name as a lower-case string. For example: 'div'
.
Currently, these css selectors work:
*
E
E F
E > F
E + F
E.class
E#id
E[attr=value]
E[attr~=search]
E[attr|=prefix]
E[attr^=prefix]
E[attr$=suffix]
E[attr*=search]
FAQs
parse and transform streaming html using css selectors
The npm package @gofunky/trumpet receives a total of 14 weekly downloads. As such, @gofunky/trumpet popularity was classified as not popular.
We found that @gofunky/trumpet demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.