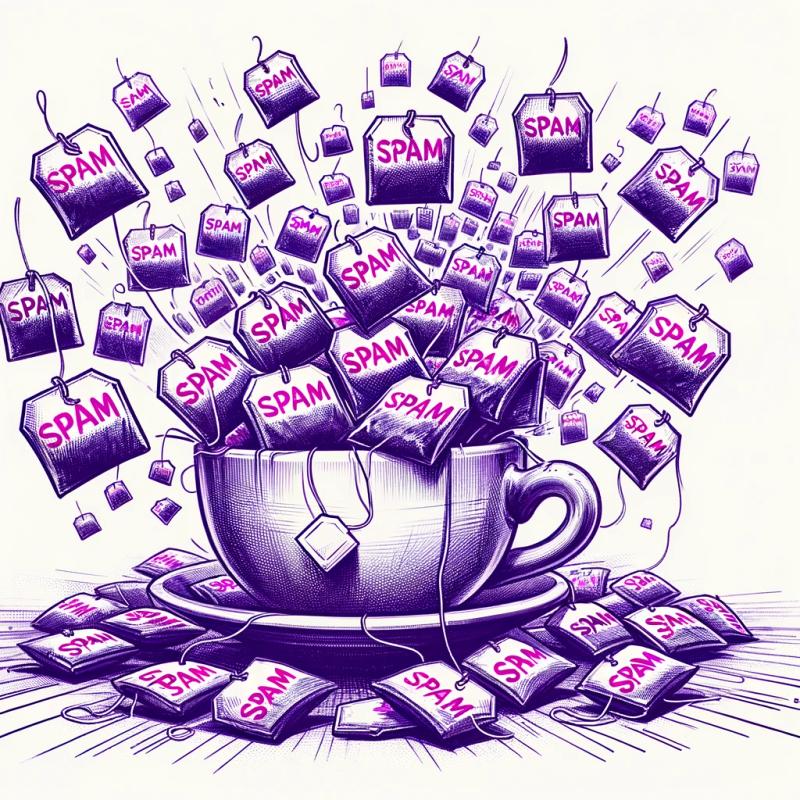
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@henningm/mp4-stream
Advanced tools
Readme
Streaming mp4 encoder and decoder
npm install mp4-stream
var mp4 = require('mp4-stream')
var fs = require('fs')
var decode = mp4.decode()
fs.createReadStream('video.mp4')
.pipe(decode)
.on('box', function (headers) {
console.log('found box (' + headers.type + ') (' + headers.length + ')')
if (headers.type === 'mdat') {
// you can get the contents as a stream
console.log('box has stream data (consume stream to continue)')
decode.stream().resume()
} else if (headers.type === 'moof') {
// you can ignore some boxes
decode.ignore()
} else {
// or you can fully decode them
decode.decode(function (box) {
console.log('box contents:', box)
})
}
}
})
All boxes have a type thats a 4 char string with a type name.
var stream = mp4.decode()
Create a new decoder.
The decoder is a writable stream you should write a mp4 file to. It emits the following additional events:
on('box', headers)
- emitted when a new box is found.Each time the box
event fires, you must call one of these three functions:
stream.ignore()
- ignore the entire box and continue parsing after its endstream.stream()
- get a readable stream of the box contentsstream.decode(callback)
- decode the box, including all childeren in the case of containers, and pass
the resulting box object to the callbackvar fs = require('fs')
var stream = mp4.decode()
stream.on('box', function (headers) {
console.log('found new box:', headers)
})
fs.createReadStream('my-video.mp4').pipe(stream)
var stream = mp4.encode()
Create a new encoder.
The encoder is a readable stream you can use to generate a mp4 file. It has the following API:
stream.box(box, [callback])
- adds a new mp4 box to the stream.var ws = stream.mediaData(size)
- helper that adds an mdat
box. write the media content to this stream.stream.finalize()
- finalizes the mp4 stream. call this when you're done.var fs = require('fs')
var stream = mp4.encode()
stream.pipe(fs.createWriteStream('my-new-video.mp4'))
stream.box(anMP4Box, function (err) {
// box flushed
var content = stream.mediaData(lengthOfStream, function () {
// wrote media data
stream.finalize()
})
someContent.pipe(content)
})
To decode and encode an mp4 file with this module do
var encoder = mp4.encode()
var decoder = mp4.decode()
decoder.on('box', function (headers) {
decoder.decode(function (box) {
encoder.box(box, next)
})
})
fs.createReadStream('my-movie.mp4').pipe(decoder)
encoder.pipe(fs.createWriteStream('my-movie-copy.mp4'))
Mp4 supports a wide range of boxes, implemented in mp4-box-encoding.
MIT
FAQs
Streaming mp4 encoder and decoder
The npm package @henningm/mp4-stream receives a total of 1 weekly downloads. As such, @henningm/mp4-stream popularity was classified as not popular.
We found that @henningm/mp4-stream demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.