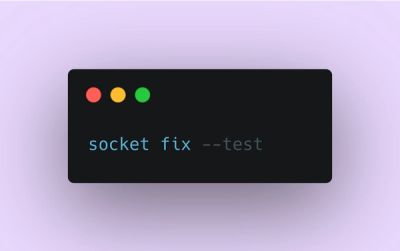
Product
Introducing Socket Fix for Safe, Automated Dependency Upgrades
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
@hfour/nestjs-json-rpc
Advanced tools
Docs | Contributing | Wiki | MIT Licensed
A JSON-RPC microservice strategy implementation for NestJS.
Currently uses HTTP as the transport layer, with plans to add other options as the need arises.
yarn add @hfour/nestjs-json-rpc
Initialize similar to a regular microservice, but pass a JSONRPCService
as the strategy option:
const app = await NestFactory.createMicroservice(ApplicationModule, {
strategy: new JSONRPCServer({
path: "/rpc/v1",
port: 8080
})
});
Decorate your controllers with @JSONRPCService
:
@JSONRpcService({
namespace: "test"
})
export class TestService implements ITestService {
public async myMethod(params: any) {
console.log("The method called was test.myMethod");
return params;
}
}
All the methods of the service will automatically be added with the name <namespace>.<method>
.
Use any standard microservice decorators you would like to use:
@UsePipes(TestPipe)
@UseInterceptors(TestInterceptor)
@UseGuards(TestGuard)
public async myMethod(params: any) {
//...
}
The standard way to use these decorators would be:
nestjs-json-rpc
also comes with a way to create clients!
Simply initialize the client in any other service, then ask it to create a proxy for TestService
for the given namespace:
@injectable()
class MyServiceClient {
private client = new JSONRPCClient("http://localhost:8080/rpc/v1");
private service = this.client.getService<ITestService>("test");
public doSomething() {
// Invoke it just as if its a local service!
return this.service.myMethod(paramsHere);
}
}
nestjs-json-rpc
comes with CodedRpcException
, which as per JSONRPC
spec allows you to include an error code and additional data to the error. The error is reconstructed on the client and you can access and check the code and the data there as well.
Feel free to define your own code constants and declare a union of the types in order to be able to discriminate the codes:
function isValidationError(e: CodedRpcException)
try {
await this.service.myMethod(paramsHere);
} catch (e: CodedRpcException) {
if (e.code === Codes.ValidationError) {
// access validation errors here:
e.data.validationErrors
}
}
You may also implement your own error hierarchy by means of subclassing, but you will not be able to use the instanceof
checks on the client as errors are not reconstructed. Instead we recommend the following approach:
const ValidationErrorCode = 400;
type ValidationErrorCode = 400;
type ValidationError = CodedRpcException & {
code: ValidationErrorCode;
data: {
validationErrors: Array<{ path: string; problem: string }>;
};
};
function isValidationError(e: any): e is ValidationError {
return e instanceof CodedRpcException && e.code === ValidationErrorCode;
}
FAQs
JSON-RPC module for NestJS framework
The npm package @hfour/nestjs-json-rpc receives a total of 2 weekly downloads. As such, @hfour/nestjs-json-rpc popularity was classified as not popular.
We found that @hfour/nestjs-json-rpc demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 9 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Automatically fix and test dependency updates with socket fix—a new CLI tool that turns CVE alerts into safe, automated upgrades.
Security News
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.
Product
We’re excited to announce a powerful new capability in Socket: historical data and enhanced analytics.