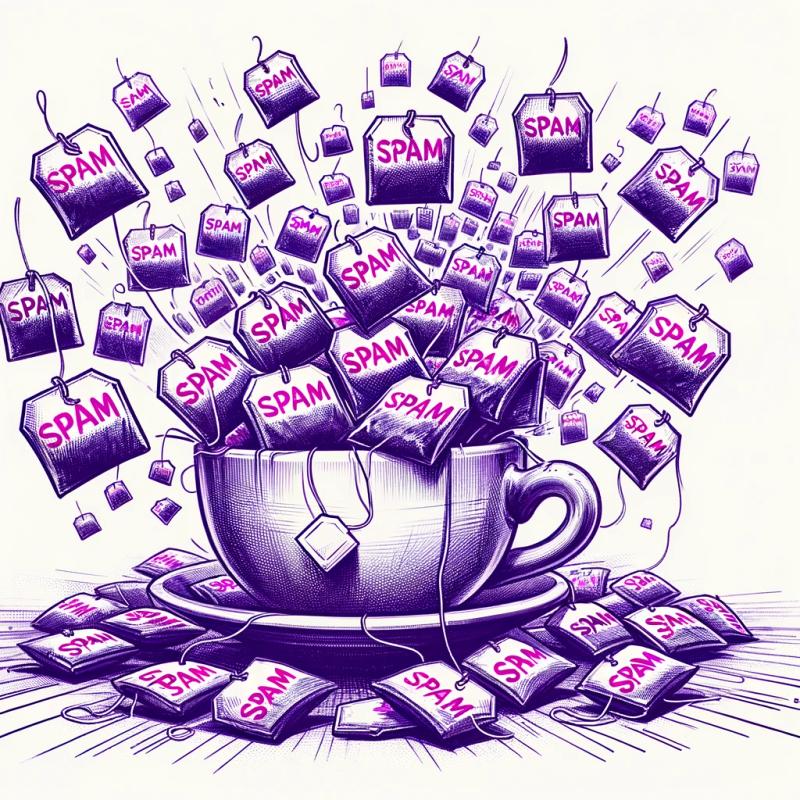
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@interopio/search-api
Advanced tools
Readme
Apps can perform searches and provide results for others' searches.
For example, let's say an app provides Seinfeld quotes, and wants to make those quotes available to any other app. The Seinfeld app could register itself as a search provider with this:
// Registering as a provider
const provider = await glue.search.registerProvider({
name: "Seinfeld-Quotes"
});
// Registering how the provider will respond to queries
provider.onQuery(({search, sendResults, done}) => {
const textOfQuote = doStuffInternally(search);
sendResults({
type: {
name: "tv-quote",
displayName: "Seinfeld Quotes"
},
id: "quote-123",
description: textOfQuote
});
done();
})
Now, let's say an app is interested to see if there are any Seinfeld quotes with the word "architect". It would use the following function:
const query = await glue.search.query({search: "architect"});
query.onResults((result) => {
/*
// Example value for result -
{
provider: {
id: "INTERNAL_ID",
interopId: "INTERNAL_INSTANCE_ID",
name: "Seinfeld-Quotes" // The search provider's name
appName: "seinfeld-app", // The app that registered the search provider
},
result: {
type: {
name: "tv-quote",
displayName: "Seinfeld Quotes"
},
id: "quote-123",
description: "You know I always wanted to pretend I was an architect.
}
}
*/
})
If you're writing a custom search provider, here are things to make sure you implement:
Basics
Other considerations:
done()
once you are done (in case queriers are waiting on onCompleted()
)types
property? You don't want to ignore the restrictions the querier placed on their query.maxResults
property? You don't want to return more that the querier asked for.maxResultsByType
error()
callback if necessary?onQueryCancel
?If you're querying a search providerm here are things to make sure you implement:
const query = await glue.search.query({search: "My search string"})
)onResults
(eg query.onResults(displaySearchResult)
)onError
Other considerations:
cancel()
your query?providerLimits
)?onCompleted
, to see when different search providers have finished? If so, does it also make sense to include a timeout (in case a search provider doesn't indicate when they're done()
?)listTypes()
to see if the type you're looking is being provided? Would it makse sense to limit your search results to a certain type?listProviders()
to see if the provider you're looking is present? Would it makse sense to limit your search results to certain providers?actions
. If that makes sense for your use cases, are you handling incoming actions?glue.search has the following methods:
Config options for .registerProvider()
are:
name
(required)types
(optional but recommended). If you register a custom type, it will appear in the .listTypes
method.const provider = await glue.search.registerProvider({name: "test"});
const provider = await glue.search.registerProvider({
name: "test",
types: [
{name: "application"}
]
});
registerProvider
returns a promise that resolves to a Provider
object. This has the following available methods:
onQuery
- callback when search queries are executedonQueryCancel
- callback when an app cancels its search queryunregister
- an unregistration method.:::tip Search providers are automatically unregistered when windows are closed. Unregistration is a good cleanup action, but may not be required. :::
The onQuery
callback provides a ProviderQuery
object, with the following properties:
query.onResults()
callback)query.onError()
callback)query.done()
callback)When sending a result, you supply a QueryResult
object. The properties of that object are:
SearchType
(eg: {name: "application"}
)You create a query object with the query()
method:
const query = await glue.search.query({search: "My search query"});
The query
method takes the following properties:
search
(required) (string) - what you're searching forproviders
(ProviderData[]
) - providers you want to limit your search totypes
(SearchType[]
) - the types of results you want to limit your search toproviderLimits.maxResults
(number) - the maximum number of results the querier wants to receive from each providerproviderLimits.maxResultsPerType
(number) - the maximum number of results PER TYPE the querier wants to recevie from each providerThe query object you receive has the following methods:
cancel()
- Cancels the query. (See provider's onQueryCancel()
)onResults()
- Callback to receive results (See provider's query handling for sendResult()
)onCompleted()
- Callback when provider is done (See provider's query handling for done()
)onError()
- Callback when provider has an error (See provider's query handling for error()
)await glue.search.listProviders();
/*
Example results: [
{
appName: "fsbl-test-butler",
id: "INTERNAL_ID",
interopId: "INSTANCE_ID",
name: "registered-search-provider-custom-name",
},
{
appName: "tester",
id: "INTERNAL_ID",
interopId: "INSTANCE_ID",
name: "my-tester",
types: [{name: "test-type"}]
}
]
*/
You can see what search types are available. This is especially useful if you are relying on a custom search type from another app, and want to confirm that that app has already been registered.
await glue.search.listTypes();
/*
Example results: [
{name: "customSearchType"},
{name: "application"},
{name: "layout"},
{name: "workspace"},
{name: "swimlane"},
{name: "action"}
]
*/
Default debounce: 0ms
Queries can be debounced. If you plan on querying based on individual keystrokes or mouse movements, you can potentially generate an unnecessarily large message flurry. Debouncing the queries will reduce search query traffic.
Here are examples of getting/setting the debounce time:
// Get the debounce time:
glue.search.getDebounceMS(); // returns 0 by default
// Set the debounce time to 20ms:
glue.search.setDebounceMS(20);
TBD
/* eslint-disable @typescript-eslint/no-explicit-any */
// package name: @interopio/search-api -> adds the Search API
// @interopio/browser-platform -> can use the package to register as a provider or use as a client, by default register as a provider for applications, workspaces, layouts and optionally actions // @interopio/browser -> can use the package to register as a provider or use as a client // GD3 -> by default register as a provider for applications, workspaces, layouts and optionally actions
// Foundation: Interop and Shared Context // lib registers: lazily two methods // lib uses: shared context to providers state management // method used when registered as provider to accept queries
// category = display name for type
FAQs
Glue42 Search API
The npm package @interopio/search-api receives a total of 154 weekly downloads. As such, @interopio/search-api popularity was classified as not popular.
We found that @interopio/search-api demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.