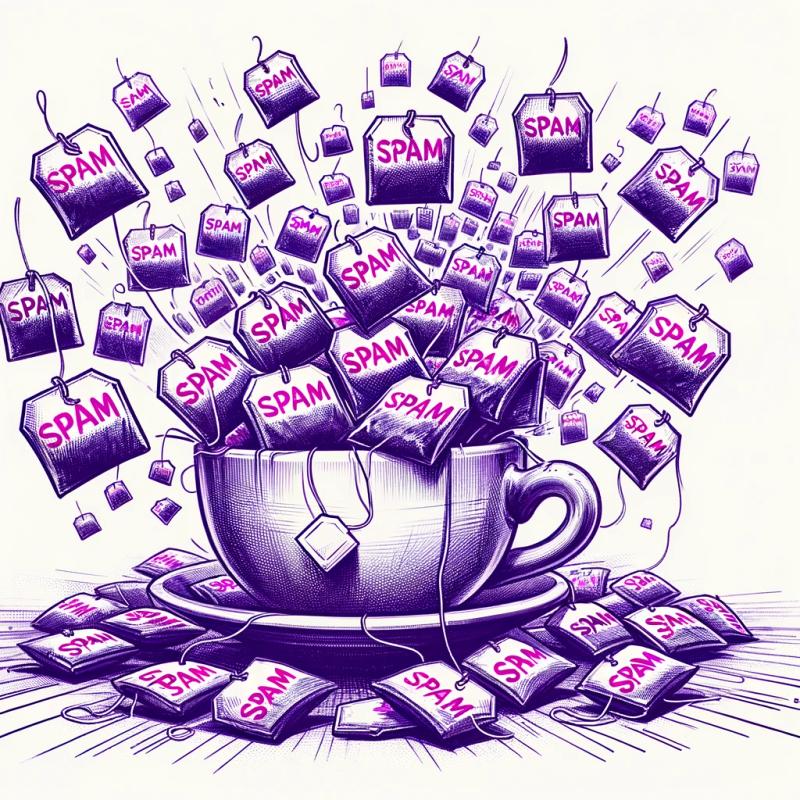
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@ionnet/orm
Advanced tools
Readme
Ionnet ORM is a TypeScript ORM for NodeJS. It is designed to be simple and easy to use, using decorators to define models and relationships, and a simple API to query the database.
This is a work in progress, and is not ready for production use. Not all features are implemented, and the API is subject to change.
npm install @ionnet/orm
Decorators need to be enabled in your tsconfig.json
file.
{
"compilerOptions": {
"experimentalDecorators": true,
}
}
import { DBModel, Field, SQLite } from "@ionnet/orm";
const sql = new SQLite(":memory:"); // SQLite("database.db") to use a file instead of memory.
class Human extends DBModel {
@Field.PrimaryKey({ auto: true })
@Field.INT()
id: number;
@Field.VARCHAR(255)
firstName: string;
@Field.VARCHAR(255)
lastName: string;
@Field.INT()
age: number;
@Field.DATE()
birthday: Date;
}
Human.initialize({
sql: sql
});
// Example of what this might look like
import { DBModel, Field, Authenticator } from "@ionnet/orm";
class User extends Authenticator.Model { // Use the Authenticator.Model class instead of DBMModel to enable authentication.
@Field.PrimaryKey({ auto: true })
@Field.INT()
id: number;
@Authenticator.Username()
@Field.INT()
username: string;
@Authenticator.Password() // This will automatically be varchar(255) and will be stored hashed.
password: string;
@Authenticator.Email()
@Field.VARCHAR(255)
email: string;
@Field.VARCHAR(255)
firstName: string;
@Field.VARCHAR(255)
lastName: string;
@Field.INT()
age: number;
@Field.DATE()
birthday: Date;
}
// Create a new user
const user = await User.register(
"username", // This will be assigned to be what the @Authenticator.Username() decorator is on.
"plaintext_password", // This will be assigned to be what the @Authenticator.Password() decorator is on.
); // Returns a new User object.
// Login
const user = await User.login(
"username",
"plaintext_password",
); // Returns a new User object. If the username or password is incorrect, it will throw an error.
FAQs
A simple TypeScript ORM for SQLite (and other databases in the future)
The npm package @ionnet/orm receives a total of 0 weekly downloads. As such, @ionnet/orm popularity was classified as not popular.
We found that @ionnet/orm demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.