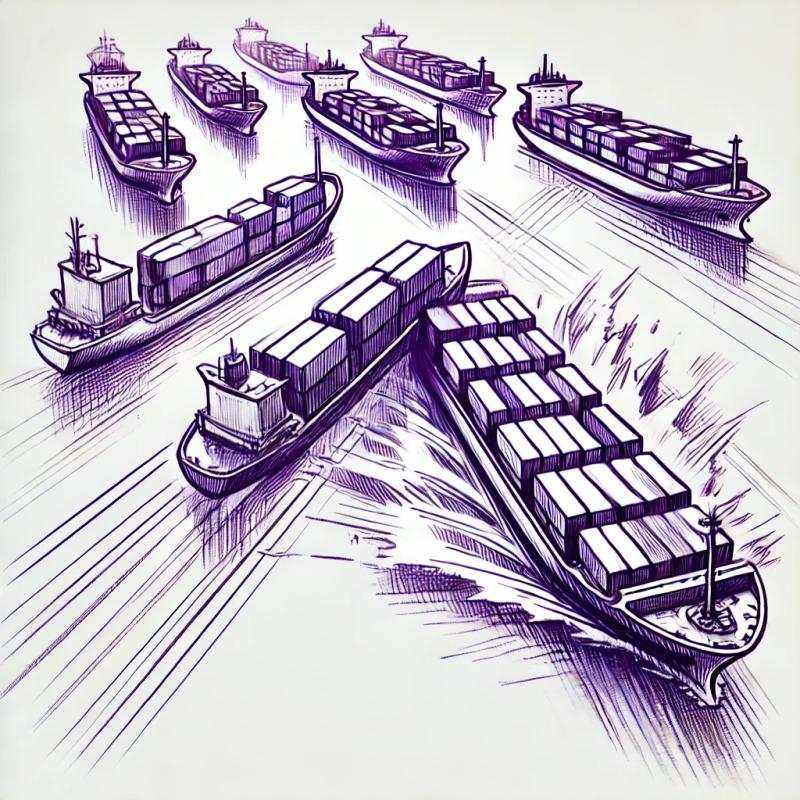
Security News
Namecheap Takes Down Polyfill.io Service Following Supply Chain Attack
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
@iteam/hooks
Advanced tools
Readme
This is a collection of hooks that we can reuse for any project that needs them. It's also a source of use cases of React Hooks and how to test them using react-testing-library.
npm install @iteam/hooks
Uses useState
but returns a setter function that toggles the value.
useToggle(initialValue: boolean): [boolean, () => void]
import React from 'react'
import { useToggle } from '@iteam/hooks'
export const ToggleComponent = () => {
const [isAlive, toggleValue] = useToggle(false)
return <button onClick={toggleValue}>{isAlive ? '🚀' : '😴'}</button>
}
Gets all the query param values
useQueryParams(): { [key: string]: string | string[] | undefined }
// https://awesome.domain/?name=cookie&lastName=monster
import React from 'react'
import { useQueryParams } from '@iteam/hooks'
export const NeedsABunchOfParams = () => {
const params = useQueryParams()
console.log(params)
// { name: 'cookie', lastName: 'monster' }
return null
}
Gets a value from a specified query param, useful if you only require one value
out of a bunch. Uses useQueryParams
under the hood.
useQueryParam(param: string): string | string[]
import React from 'react'
import { useQueryParam } from '@iteam/hooks'
export const NeedsAParam = () => {
const param = useQueryParam('sweetParam')
return (
<div>
{typeof param === 'string'
? `That's a nice query param with the value ${param}`
: `Woah! A bunch of params ${param.join(',')}`}
</div>
)
}
Debounce the updating of a value
useDebounce<ValueType>(value: ValueType, duration: number): ValueType
import React from 'react'
import { useDebounce } from '@iteam/hooks'
export const Debounced = () => {
const [inputValue, setInputValue] = React.useState('')
const debouncedValue = useDebounce(inputValue, 300)
const handleChange = e => {
setInputValue(e.currentTarget.value)
}
return (
<div>
<label htmlFor="test-input">Best field ever</label>
<input id="test-input" onChange={handleChange} value={debouncedValue} />
{debouncedValue}
</div>
)
}
Get and set values in localStorage
. Uses useStorage
under the hood.
useLocalStorage(key: string, initialValue?: any): [string, (newValue: string) => void]
import React from 'react'
import { useLocalStorage } from '@iteam/hooks'
export const Storage = ({ initialValue }) => {
const [value, setValue] = useLocalStorage('storedValue', initialValue)
return (
<div>
{value ? value : 'no value ;('}
<label htmlFor="store">Update store value</label>
<input
id="store"
onChange={e => setValue(e.currentTarget.value)}
type="text"
value={value}
/>
</div>
)
}
Get and set values in any store with a getItem
or setItem
. Defaults to
localStorage
. Useful if you want to add something to for example sessionStorage
.
useStorage(key: string, options?: { initialValue?: any, store?: Storage }): [string, (newValue: string) => void]
import React from 'react'
import { useStorage } from '@iteam/hooks'
export const Storage = ({ initialValue }) => {
const [value, setValue] = useStorage('storedValue', {
initialValue,
store: sessionStorage,
})
return (
<div>
{value ? value : 'no value ;('}
<label htmlFor="store">Update store value</label>
<input
id="store"
onChange={e => setValue(e.currentTarget.value)}
type="text"
value={value}
/>
</div>
)
}
FAQs
A collection of reusable hooks
We found that @iteam/hooks demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 7 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Polyfill.io has been serving malware for months via its CDN, after the project's open source maintainer sold the service to a company based in China.
Security News
OpenSSF is warning open source maintainers to stay vigilant against reputation farming on GitHub, where users artificially inflate their status by manipulating interactions on closed issues and PRs.
Security News
A JavaScript library maintainer is under fire after merging a controversial PR to support legacy versions of Node.js.