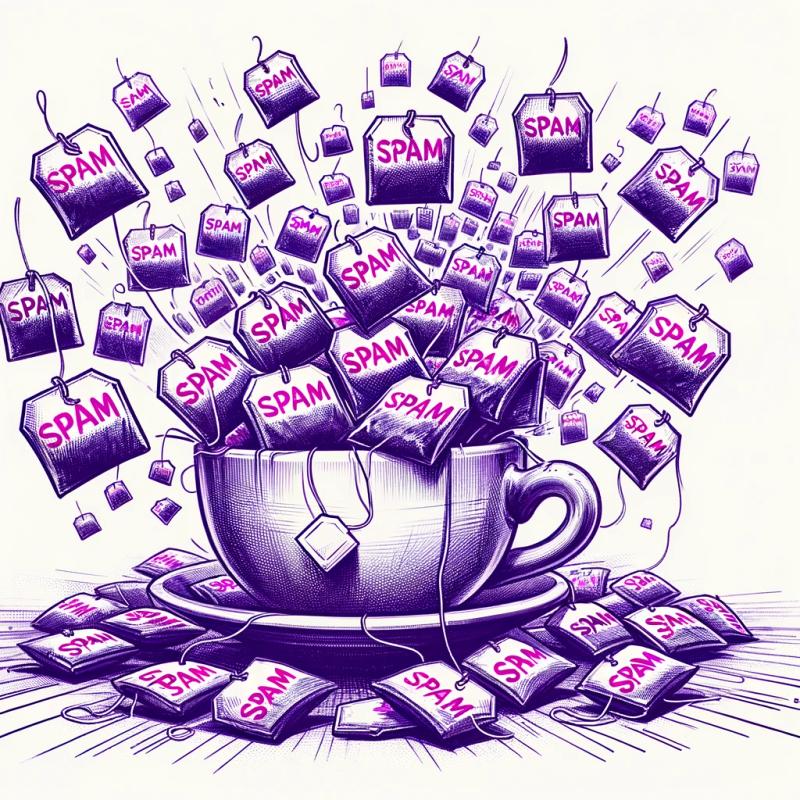
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@janiscommerce/event-listener
Advanced tools
Changelog
[5.0.1] - 2023-09-18
Readme
An API handler to implement event listeners
npm install @janiscommerce/event-listener
This is the class you should extend to code your own Listeners. You can customize them with the following methods and getters:
This optional method should throw an Error in case of validation failure. It's message will be set in the response body. It's return value will be discarded. If you dont implement this method, it will automatically validate your event structure.
IMPORTANT If you implement it, remember to always call super.validate()
before so your event structure get validated for you.
This method is required, and should have the logic of your API. At this point, request should be already validated. If you throw an error here, default http code is set to 500.
The following methods will be inherited from the base API Class:
mustHaveClient (getter).
Indicates whether the events must have the client
property present when validating it. Default is false.
mustHaveId (getter).
Indicates whether the events must have the id
property present when validating it. Default is false.
event (getter). Returns the event object
eventService (getter). Returns the event source service
eventEntity (getter). Returns the event entity
eventName (getter). Returns the event name
eventClient (getter).
Returns the event client if present or undefined
otherwise.
eventId (getter).
Returns the event id if present or undefined
otherwise.
You can also use getters and setters defined in @janiscommerce/api.
This is the class you should use as a handler for your AWS Lambda functions.
This will handle the lambda execution.
EventListener
class.Handled runtime errors of the event listener. You might find more information about the error source in the previousError
property.
It also uses the following error codes:
Name | Value | Description |
---|---|---|
Missing event | 1 | The request body is empty |
Invalid event | 2 | The request body has an invalid event object |
Since 2.0.0
This package implements API Session. In order to associate a request to a session, you must pass a valid authentication data in the authenticationData
property of the Dispatcher constructor.
Session details and customization details can be found in api-session README.
ColorUpdatedListener:
handler: src/listeners/color/updated.handler
description: Color updated listener
events:
- http:
integration: lambda
path: listener/profile/created
method: post
caching:
enabled: ${self:custom.apiGatewayCaching.enabled}
request:
template: ${file(./serverless/functions/subtemplates/lambda-request-with-path.yml)}
response: ${file(./serverless/functions/subtemplates/lambda-response-with-cors.yml)}
# This is for serverless-offline only
responses: ${file(./serverless/functions/subtemplates/lambda-serverless-offline-responses.yml)}
'use strict';
const {
EventListener,
ServerlessHandler
} = require('@janiscommerce/event-listener');
class MyEventListener extends EventListener {
async process() {
this.setBody({
event: this.event
});
}
}
module.exports.handler = (...args) => ServerlessHandler.handle(MyEventListener, ...args);
'use strict';
const {
EventListener,
ServerlessHandler
} = require('@janiscommerce/event-listener');
class MyEventListener extends EventListener {
get mustHaveClient() {
return true;
}
get mustHaveId() {
return true;
}
async process() {
this.setBody({
eventClient: this.eventClient,
eventId: this.eventId
});
}
}
module.exports.handler = (...args) => ServerlessHandler.handle(MyEventListener, ...args);
'use strict';
const {
EventListener,
ServerlessHandler
} = require('@janiscommerce/event-listener');
const MyModel = require('../../models/mine');
class MyEventListener extends EventListener {
get mustHaveClient() {
return true;
}
async process() {
const client = await this.session.client;
const model = this.session.getSessionInstance(MyModel);
return model.update({
someFieldToUpdate: client.status
}, {
entity: this.eventEntity
});
}
}
module.exports.handler = (...args) => ServerlessHandler.handle(MyEventListener, ...args);
FAQs
An API handler to implement event listeners
The npm package @janiscommerce/event-listener receives a total of 536 weekly downloads. As such, @janiscommerce/event-listener popularity was classified as not popular.
We found that @janiscommerce/event-listener demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.