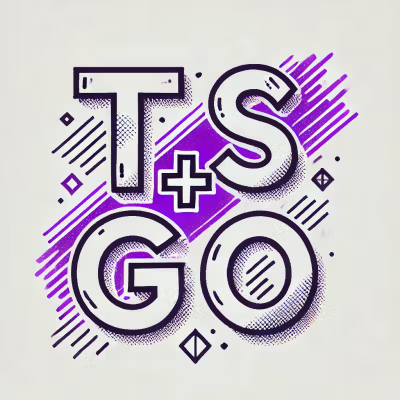
Security News
TypeScript is Porting Its Compiler to Go for 10x Faster Builds
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
@jbroll/nmea-widgets
Advanced tools
Modern React/Preact components for visualizing NMEA GPS data in real-time using Web Serial API
A modern React/Preact component library for visualizing NMEA GPS data in real-time using Web Serial, Web Bluetooth, and Geolocation APIs. Built with TypeScript and Tailwind CSS.
npm install @jbroll/nmea-widgets
This package has peer dependencies that you'll need to install in your project:
npm install preact tailwindcss
tailwind.config.js
:/** @type {import('tailwindcss').Config} */
export default {
content: [
"./index.html",
"./src/**/*.{js,ts,jsx,tsx}",
"./node_modules/@jbroll/nmea-widgets/**/*.{js,ts,jsx,tsx}"
],
theme: {
extend: {},
},
plugins: [],
}
/* styles.css */
@tailwind base;
@tailwind components;
@tailwind utilities;
import { NMEADisplay } from '@jbroll/nmea-widgets';
function App() {
return <NMEADisplay />;
}
For more control over the NMEA processing, you can use the useNMEA
hook and individual components:
import { useNMEA, NMEADetailView, NMEAButton } from '@jbroll/nmea-widgets';
function CustomDisplay() {
const {
serialData,
processedData,
isConnected,
connect,
disconnect,
setFilter,
supportedTypes
} = useNMEA();
return (
<div>
<div className="flex justify-between">
<h1>GPS Data</h1>
<NMEAButton
detailsLabel="View Details"
onDetailsClick={() => {
// Handle details view
}}
/>
</div>
<NMEADetailView processedData={processedData} />
</div>
);
}
The main component that provides a complete UI for NMEA data visualization. Automatically manages connection and data processing.
<NMEADisplay
onDetailsClick={() => {
// Handle details view navigation
}}
/>
A status indicator and connection management button.
<NMEAButton
detailsLabel="View Details" // Optional label for details action
onDetailsClick={() => {}} // Optional callback for details action
className="custom-styles" // Optional class name for styling
/>
Features:
A standalone component for visualizing satellite positions and signal strengths.
import { SatellitePlot } from '@jbroll/nmea-widgets';
<SatellitePlot data={processedData} />
Features:
Displays position information and accuracy metrics.
import { NMEAInfo } from '@jbroll/nmea-widgets';
<NMEAInfo data={processedData} />
Features:
A React hook that handles device communication and NMEA data processing.
const {
serialData, // Raw NMEA sentences
processedData, // Parsed and processed NMEA data
isConnected, // Connection state
isConnecting, // Connection in progress
error, // Error state
connect, // Function to initiate connection
disconnect, // Function to close connection
sendCommand, // Function to send commands to device
setFilter, // Function to filter NMEA sentences
supportedTypes, // Array of supported connection types
connection // Current connection interface
} = useNMEA();
interface ProcessedData {
position: {
latitude: number;
longitude: number;
altitudeMeters: number;
fixType: number;
satellites: number;
} | null;
errorStats: {
latitudeError: number;
longitudeError: number;
altitudeError: number;
} | null;
satellites: {
visible: Satellite[];
inUse: number[];
};
}
interface Satellite {
prnNumber: number;
elevationDegrees: number;
azimuthTrue: number;
SNRdB: number;
constellation: string;
}
The library currently supports parsing these NMEA sentence types:
Supported constellations:
The library supports various UART/Serial over Bluetooth LE services including:
To build the library:
npm install
npm run build
To run the demo application:
cd examples/nmea-demo
npm install
npm run dev
Contributions are welcome! Please feel free to submit a Pull Request.
The project uses:
MIT License - see LICENSE file for details
FAQs
Modern React/Preact components for visualizing NMEA GPS data in real-time using Web Serial API
The npm package @jbroll/nmea-widgets receives a total of 33 weekly downloads. As such, @jbroll/nmea-widgets popularity was classified as not popular.
We found that @jbroll/nmea-widgets demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
TypeScript is porting its compiler to Go, delivering 10x faster builds, lower memory usage, and improved editor performance for a smoother developer experience.
Research
Security News
The Socket Research Team has discovered six new malicious npm packages linked to North Korea’s Lazarus Group, designed to steal credentials and deploy backdoors.
Security News
Socket CEO Feross Aboukhadijeh discusses the open web, open source security, and how Socket tackles software supply chain attacks on The Pair Program podcast.