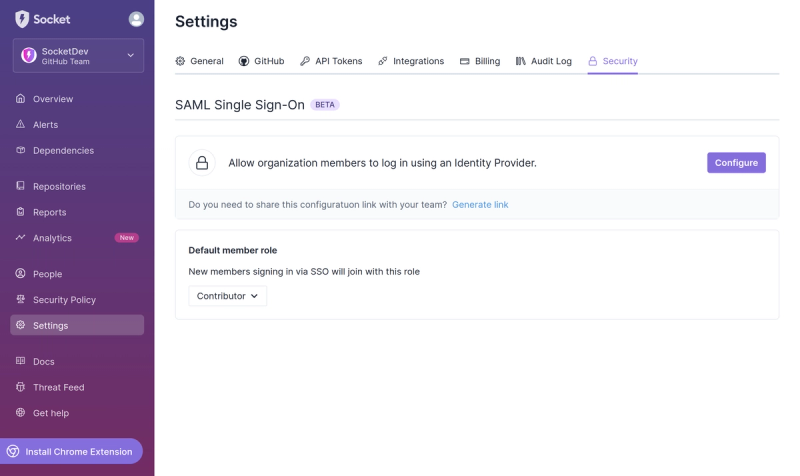
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
@krishnapawar/kp-mysql-models
Advanced tools
The `kp-mysql-models` library simplifies MySQL database interaction, streamlining tasks such as creating, inserting, updating, and deleting records, as well as handling complex queries like joins, pagination, and conditional operations. By offering an int
Readme
The
kp-mysql-models
is a mysql query builder light weight library that simplifies interactions with MySQL databases. It streamlines tasks such as creating, inserting, updating, and deleting records, and handles complex operations like joins, pagination, and conditionals. Its intuitive and efficient approach can greatly expedite development, saving both time and effort.
npm i kp-mysql-models
npm i @krishnapawar/kp-mysql-models
This package provides a set of models for working with MySQL database. It is built on top of the mysql
npm module
Note:-
for connection mysql we must have use library mysql
for example.
var mysql = require("mysql");
var pool = mysql.createPool({
connectionLimit: 10,
host: "localhost",
user: "root",
password: "",
database: "test",
});
using your modele class by extendes BaseModels class for Example
const { BaseModels } = require("kp-mysql-models");
const { pool } =require("./db");
class User extends BaseModels{
constructor(){
super();
this._table="users";
this._connection=pool;
}
}
module.exports= User;
No need to connect table name if class name same as table name but without s. for exmaple we have users table then we make User model class. also we sort hand connet database by using super() method;
const { BaseModels } = require("kp-mysql-models");
const { pool } =require("./db");
class User extends BaseModels{
constructor(){
super();
this._connection=pool;
}
}
module.exports= User;
class User extends BaseModels{
constructor(){
super(pool);
}
}
module.exports= User;
You can access all methods after make User class object for Example
let user = new User;
let data = await user.first();
let data = await user.get();
//deleting data
let data = await user.delele({
where: {
id: 585,
}
});
let data = await user.deleleAll();
let data = await user.destroy({
where: {
id: 585,
}
});
//trucate table
let data = await user.trunCate();
We can use soft delete as well by using BaseModels class for Example
let user = new User;
class User extends BaseModels{
constructor(){
super(pool);
this._softDelete=true;
}
}
// for soft deleteing data
let data = await user.trashed({
where: {
id: 585,
}
});
// for soft deleteing All data
let data = await user.trashedAll();
// for soft deleteing restoring data
let data = await user.restore({
where: {
id: 585,
}
});
//for soft deleteing restoring All data
let data = await user.restoreAll();
//for fetch soft deleted data useing onlyTrashed:true;
let data = await user.first({
onlyTrashed:true,
where: {
id: 585,
}
});
let data = await user.get({ onlyTrashed:true });
or you can use same like abow example.
let data = await user.get({
select: ["id", "firstname", "lastname"],
with: {
doctor: {
table: "appointments",
limit: 2,
select: ["id", "user_id"],
connect: {
user_id: "id",
},
},
clinic: {
table: "appointments",
limit: 2,
select: ["id", "user_id"],
connect: {
doctor_id: "id",
},
},
},
where: {
id: 585,
},
});
first method for geting single data
const data = await first({
table: "users",
select: ["id", "first_name", "last_name"],
limit: 10,
latest: "id",
whereNotIn: {
id: [1, 1221],
},
whereIs: {
last_name: "NULL",
},
where:{
id:1
}
});
get methods
const data = await user.get({
select: ["id", "first_name", "last_name"],
limit: 10,
latest: "id",
whereNotIn: {
id: [1, 1221],
},
whereIs: {
last_name: "NULL",
},
});
we can make dyanamic pagination with key word (pagination:1) 1 is page No. we can set page limit by (limit:10) key word 10 is 10 data per page
const data = await user.get({
limit: 10,
pagination: 1,
});
let page = req.query.page;
const data = await user.get({
limit: 10,
pagination: page,
});
let data = await User.findOne(13);
let data = await User.findOneById(13);
let data = await User.find({
id:12,
name:"test",
date:"12/10/2023"
});
using with() method using with first method to fetch data in specific variable in object
const data = await user.first({
select: [
"id",
"first_name",
"last_name"
],
with: {
doctor: {
table: "appointments",
limit: 2,
select: ["id", "user_id"],
connect: {
user_id: "id",
},
},
clinic: {
table: "appointments",
limit: 2,
select: ["id", "user_id"],
connect: {
doctor_id: "id",
},
},
},
where: {
id: 585,
},
});
Applying the with() method in conjunction with the get method to retrieve data and store it in a specific variable within an object.
const data = await user.get({
select: ["id", "created_by_id", "first_name", "last_name"],
with: {
doctor: {
table: "appointments",
limit: 2,
select: ["id", "user_id"],
connect: {
user_id: "id",
},
},
clinic: {
table: "appointments",
limit: 2,
select: ["id", "user_id"],
connect: {
doctor_id: "id",
},
},
},
where: {
created_by_id: "1",
},
});
hasOne
, belognsTo
, hasMany
, connect
in (with:{}). {
select:['id','first_name','role_id','created_at'],
whereIsNotNull:['last_name'],
with:{
hasOne_appointment:{
select:['id','user_id'],
table:"appointments",
hasOne:{
user_id:'id'
}
},
belongsTo_appointment:{
select:['id','user_id'],
table:"appointments",
belongsTo:{
user_id:'id'
}
},
connect_appointment:{
select:['id','user_id'],
table:"appointments",
connect:{
user_id:'id'
}
},
hasMany_appointment:{
select:['id','user_id'],
table:"appointments",
hasMany:{
user_id:'id'
}
}
}
}
WE can get multi level relational data using with
let data = await User.get({
with:{
appointment:{
select:['id','user_id'],
table:"appointments",
hasOne:{
user_id:'id'
},
with:{
doctor:{
select:['id as doctor_id','email'],
table:"users",
hasOne:{
user_id:'id'
},
with:{
clinic_data:{
table:"clinices",
hasOne:{
id:'doctor_id'
}
}
}
}
}
}
}
});
belongsTo
andhasOne
give single response with single object data and other handhasMany
andconnect
, give array object response with multiple object data `.
const data = await user.dbJoin({
table: "users",
limit: 10,
select: [
"users.id as uId",
"appointments.id",
"users.first_name",
"lab.first_name as lab_name",
],
latest: "appointments.id",
join: [
{
type: "hasOne",
table: "appointments",
on: {
"users.id": "appointments.patient_id",
},
},
{
type: "belongsTo",
table: "users lab",
on: {
"lab.id": "appointments.user_id",
},
},
],
where: {
"users.id": 1122,
},
pagination: page,
});
you can also use for this method to join mutlipal table
const data = await user.dbWith({
table: "users",
limit: 10,
select: [
"users.id as uId",
"appointments.id",
"users.first_name",
"lab.first_name as lab_name",
],
latest: "appointments.id",
with: {
hasOne: [
{
table: "appointments",
on: {
"users.id": "appointments.patient_id",
},
},
{
table: "users clinic",
on: {
"clinic.id": "appointments.clinic_id",
},
},
],
belongsTo: [
{
table: "users lab",
on: {
"lab.id": "appointments.user_id",
},
},
],
},
where: {
"users.id": 1122,
},
pagination: page,
});
Note:- we can use left join
, right join
, join
and inner join
instant of hasOne
, belognsTo
, hasMany
, connect
in dbJoin()
,dbWith()
and also with with
.
import all Helper method Example
const {
setDBConnection,
get,
first,
save,
create,
update,
dbJoin,
dbWith,
} = require("kp-mysql-models");
first you have to setup mysql connection for using helper. we can setup by using setBDConnection() method to connect database or we can directly pass mysql pool or db connection object or params in help method look both example in below.
var mysql = require("mysql");
var pool = mysql.createPool({
connectionLimit: 10,
host: "localhost",
user: "root",
password: "",
database: "test",
});
Example 1 for using setDBConnection method
setDBConnection(pool);
const data = await get({
table: "users",
whereNotIn: {
id: [1, 1221],
}
});
You can also pass the connection object to each method directly Example 2 for directly pass db connection
const data = await get({
table: "users",
whereNotIn: {
id: [1, 1221],
}
},pool);
Available important Helper methods can we use as well
create method using for create data
const data = await create({
table: "users",
elements: {
first_name: "ram",
last_name: "ji",
}
});
update method using for updating data
const dataj = await update({
table: "users",
elements: {
first_name: "ram",
last_name: "ji",
},
where: {
id: 1223,
}
});
save method using for create or updating data
const dataj = await save({
table: "users",
elements: {
first_name: "ram",
last_name: "ji",
},
// where: {
// id: 1223,
// },
});
table:
select:
elements:
latest:
limit:
pagination:
with:
connect:
hasOne:
belongsTo:
hasMany:
join:
dbWith:
where:
whereOr, whereIn, whereNotIn, whereIs, whereIsNull, whereIsNotNull, whereRaw:
on, onOr, onIn, onNotIn, onIs, onRaw:
onlyTrashed:
groupBy:
where
and on
operations with exampleswhere:-
where: {
id: 1223,
}
whereOr:-
whereOr: {
id: 1223,
}
whereIn:-
whereIn: {
id: [1, 1221],
}
whereNotIn:-
whereNotIn: {
id: [1, 1221],
}
whereIs:-
whereIs: {
last_name: "NULL",
}
whereIsNot:-
whereIsNot: {
last_name: "NULL",
}
whereRaw:-
whereRaw:"name='mohan' and age=30 "
on:-
on: {
id: 1223,
}
onOr:-
onOr: {
id: 1223,
}
onIn:-
onIn: {
id: [1, 1221],
}
onNotIn:-
onNotIn: {
id: [1, 1221],
}
onIs:-
onIs: {
last_name: "NULL",
}
onIsNot:-
onIsNot: {
last_name: "NULL",
}
onRaw:-
onRaw:"name='mohan' and age=30 "
FAQs
The `kp-mysql-models` library simplifies MySQL database interaction, streamlining tasks such as creating, inserting, updating, and deleting records, as well as handling complex queries like joins, pagination, and conditional operations. By offering an int
The npm package @krishnapawar/kp-mysql-models receives a total of 4 weekly downloads. As such, @krishnapawar/kp-mysql-models popularity was classified as not popular.
We found that @krishnapawar/kp-mysql-models demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.