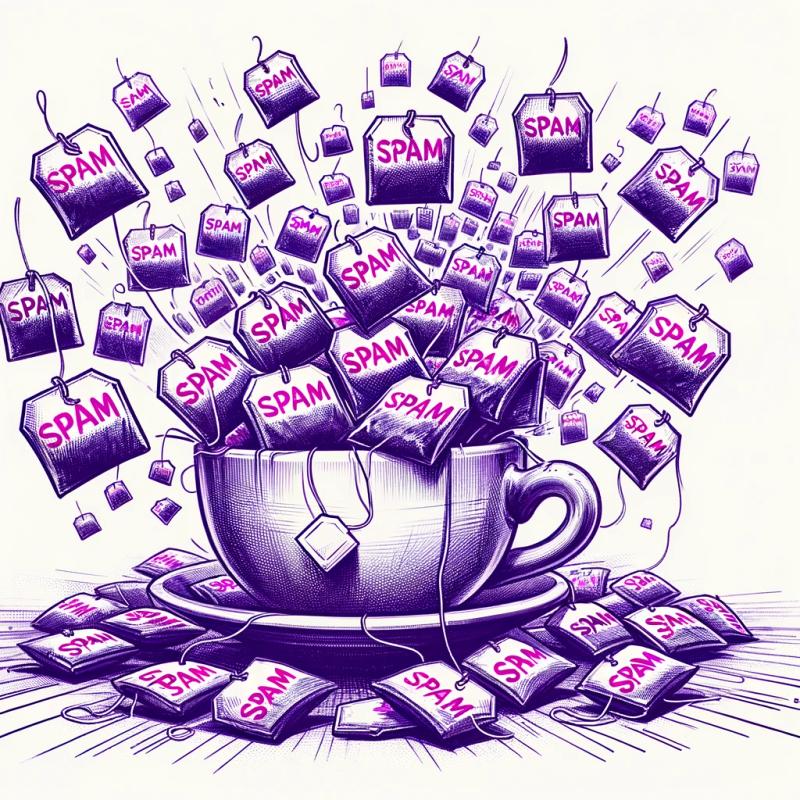
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@laserfiche/lf-oauth-api-client
Advanced tools
This package is a Typescript/Javascript OAuth 2.0 client for authorizing to the Laserfiche API using the client credentials flow. You will need a properly configured Service app as well as at least one **JSON Web Key (JWK)** access key from the Laserfich
Readme
This package is a Typescript/Javascript OAuth 2.0 client for authorizing to the Laserfiche API using the client credentials flow. You will need a properly configured Service app as well as at least one JSON Web Key (JWK) access key from the Laserfiche Developer Console. You can download a .txt file of the access key from the Developer Console.
Name TBD
npm install laserfiche-service-app-oauth-client
Ensure that the Service app is properly configured with a valid Service Principal and at least one access key.
A ClientCredentialsOptions object is a plain Javascript object with three required properties:
{
// The client ID for the application. You can find the client ID
// on the Laserfiche Developer Console config page for your application
clientId: string;
// The service principal key for the associated service principal user
// for the application. You can configure service principals in
// the Laserfiche Account Administration page under
// "Service Principals"
servicePrincipalKey: string;
// A valid JWK access key taken from the Laserfiche Developer Console
// config page for your application.
signingKey: JWK;
}
The signingKey
must be a JWK in the following format:
{
kty: "EC";
use: "sig";
crv: "P-256";
d: string;
x: string;
y: string;
kid: string;
}
Note that a key downloaded or copied directly from the Laserfiche Developer Console will inherently be in this format and thus usable.
let client: ClientCredentialsGrantHandler = new ClientCredentialsGrantHandler(testConfig);
let accessToken: AccessToken = await client.getAccessToken("https://signin.laserfiche.com/OAuth/Token");
Note that the specific endpoint will depend on your region.
An access token is an object of the following format:
{
access_token: string;
expire_in: number;
token_type: string;
}
If the request was successful, you can now make authorized requests to the Laserfiche API using the access token you receive.
We will create a new ClientCredentialsOptions
and ClientCredentialsGrantHandler
as outlined above to retrieve an access token from the Laserfiche OAuth Server. You can then use Axios, fetch, or your HTTP client of choice to create a new Laserfiche API session and make an API call to retrieve the list of entries from a repository.
First, create a ClientCredentialsObject:
import { AccessToken, ClientCredentialsGrantHandler, ClientCredentialsOptions, JWK } from 'laserfiche-service-app-oauth-client';
// Setup our ClientCredentialsOptions object
let signingKey: JWK = {
"kty": "EC",
"crv": "P-256",
"use": "sig",
"kid": "atOfVBe38QD-9Msk1wL0qt5D9YQIM0zWulFqSdTbYGs",
"x": "r7YyDvPUEstFVTuAih-SyR2Xy626ry44hIOzMkgCA7M",
"y": "DJOYCafNLXqpTGzxI_9fGNW6czmC_biWxau8VDHUU0o",
"d": "Yolx3mlDkfIOpVBORzZz2h3ySFsFRibkOELdSxqRDzU"
};
let clientId: string = "rTXtFqwwatWX0qlTdJnUtOi1";
let servicePrincipalKey: string = "KkeSGwKcqoOKH5_8g1RR";
let testConfig: ClientCredentialsOptions = {
clientId: clientId,
servicePrincipalKey: servicePrincipalKey,
signingKey: signingKey
}
Create a ClientCredentialsGrantHandler to request a new access token:
// Get an access token using the client credentials flow
console.log("Retrieving access token...");
let inst: ClientCredentialsGrantHandler = new ClientCredentialsGrantHandler(testConfig);
let accessToken: AccessToken = await inst.getAccessToken("https://signin.a.clouddev.laserfiche.com/oauth/Token");
if (accessToken) {
console.log("Access token retrieved: ", accessToken?.access_token);
}
Once you have your access token, use it to create a new session with the Laserfiche API server:
import axios, { AxiosRequestConfig } from 'axios';
// Our Laserfiche repository ID
let repoId: string = "r-23456789";
// Set the access token as the bearer token for authorization
axios.defaults.headers.common['Authorization'] = `Bearer ${accessToken?.access_token}`;
// Create a new Laserfiche API session
console.log("Creating new session...");
let createServerSessionReq: AxiosRequestConfig = {
method: 'POST',
url: `https://api.a.clouddev.laserfiche.com/repository/v1/Repositories/${repoId}/ServerSession/Create`,
};
try {
// No need to store the response
await axios.request(createServerSessionReq);
} catch (error) {
console.error(error);
}
import fetch from 'isomorphic-fetch';
// Our Laserfiche repository ID
let repoId: string = "r-23456789";
// Create a new Laserfiche API session
console.log("Creating new session...");
let createServerSessionEndpoint = `https://api.a.clouddev.laserfiche.com/repository/v1/Repositories/${repoId}/ServerSession/Create`;
// Set the access token as the bearer token for authorization
let createServerSessionReq: RequestInit = {
method: 'POST',
headers: new Headers({
'content-type': 'application/json',
'withCredentials': 'true',
'credentials': 'include',
'Authorization': `Bearer ${accessToken?.access_token}`
}),
body: 'grant_type=client_credentials'
};
try {
// No need to store the response
await fetch(createServerSessionEndpoint, createServerSessionReq);
} catch (error) {
console.error(error);
}
After creating a new session with the Laserfiche API server, use your access token to make further requests to the Laserfiche API:
// Retrieve entries from repository
console.log("Retrieving repository entries...");
let entryId: number = 1;
let getEntriesReq: AxiosRequestConfig = {
method: 'GET',
url: `https://api.a.clouddev.laserfiche.com/repository/v1/Repositories/${repoId}/Entries/${entryId}/Laserfiche.Repository.Folder/children`,
};
try {
const { data } = await axios.request(getEntriesReq);
// Log the list of entries
console.log(data);
} catch (error) {
console.error(error);
}
// Retrieve entries from repository
console.log("Retrieving repository entries...");
let entryId: number = 1;
let getEntriesEndpoint = `https://api.a.clouddev.laserfiche.com/repository/v1/Repositories/${repoId}/Entries/${entryId}/Laserfiche.Repository.Folder/children`;
// Set the access token as the bearer token for authorization
let getEntriesReq: RequestInit = {
method: 'GET',
headers: new Headers({
'content-type': 'application/json',
'withCredentials': 'true',
'credentials': 'include',
'Authorization': `Bearer ${accessToken?.access_token}`
}),
};
try {
let response = await fetch(getEntriesEndpoint, getEntriesReq);
let data = await response.json();
console.log(data);
} catch (error) {
console.error(error);
}
You can also run this example yourself by running npm run example
and checking out the /example
folder.
Run the Jest test suite with npm test
.
FAQs
This package is a Typescript/Javascript OAuth 2.0 client for authorizing to the Laserfiche API using the client credentials flow. You will need a properly configured Service app as well as at least one **JSON Web Key (JWK)** access key from the Laserfich
We found that @laserfiche/lf-oauth-api-client demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.