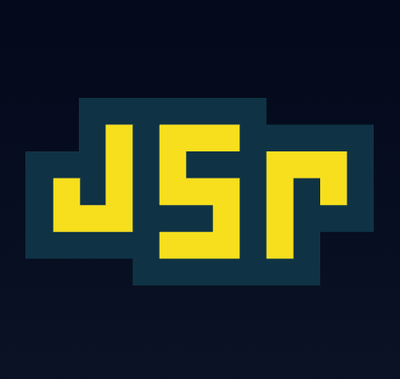
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@lexical/rich-text
Advanced tools
@lexical/rich-text is a package that provides rich text editing capabilities using the Lexical framework. It allows developers to create feature-rich text editors with support for various text formatting options, embedded media, and more.
Basic Text Formatting
This code sets up a basic rich text editor with support for text formatting, undo/redo functionality, and change tracking.
import { LexicalComposer } from '@lexical/react/LexicalComposer';
import { RichTextPlugin } from '@lexical/react/LexicalRichTextPlugin';
import { ContentEditable } from '@lexical/react/LexicalContentEditable';
import { HistoryPlugin } from '@lexical/react/LexicalHistoryPlugin';
import { OnChangePlugin } from '@lexical/react/LexicalOnChangePlugin';
const editorConfig = {
namespace: 'MyEditor',
theme: {},
onError: (error) => {
console.error(error);
},
};
function MyEditor() {
return (
<LexicalComposer initialConfig={editorConfig}>
<RichTextPlugin
contentEditable={<ContentEditable />}
placeholder={<div>Enter some text...</div>}
/>
<HistoryPlugin />
<OnChangePlugin onChange={(editorState) => {
console.log(editorState);
}} />
</LexicalComposer>
);
}
Custom Toolbar
This code demonstrates how to integrate a custom toolbar plugin into the rich text editor, allowing for additional text formatting options and controls.
import { LexicalComposer } from '@lexical/react/LexicalComposer';
import { RichTextPlugin } from '@lexical/react/LexicalRichTextPlugin';
import { ContentEditable } from '@lexical/react/LexicalContentEditable';
import { HistoryPlugin } from '@lexical/react/LexicalHistoryPlugin';
import { OnChangePlugin } from '@lexical/react/LexicalOnChangePlugin';
import { ToolbarPlugin } from './ToolbarPlugin'; // Custom toolbar plugin
const editorConfig = {
namespace: 'MyEditor',
theme: {},
onError: (error) => {
console.error(error);
},
};
function MyEditor() {
return (
<LexicalComposer initialConfig={editorConfig}>
<ToolbarPlugin />
<RichTextPlugin
contentEditable={<ContentEditable />}
placeholder={<div>Enter some text...</div>}
/>
<HistoryPlugin />
<OnChangePlugin onChange={(editorState) => {
console.log(editorState);
}} />
</LexicalComposer>
);
}
Embedding Media
This code shows how to add support for embedding images within the rich text editor using the ImagePlugin.
import { LexicalComposer } from '@lexical/react/LexicalComposer';
import { RichTextPlugin } from '@lexical/react/LexicalRichTextPlugin';
import { ContentEditable } from '@lexical/react/LexicalContentEditable';
import { HistoryPlugin } from '@lexical/react/LexicalHistoryPlugin';
import { OnChangePlugin } from '@lexical/react/LexicalOnChangePlugin';
import { ImagePlugin } from '@lexical/react/LexicalImagePlugin';
const editorConfig = {
namespace: 'MyEditor',
theme: {},
onError: (error) => {
console.error(error);
},
};
function MyEditor() {
return (
<LexicalComposer initialConfig={editorConfig}>
<RichTextPlugin
contentEditable={<ContentEditable />}
placeholder={<div>Enter some text...</div>}
/>
<HistoryPlugin />
<OnChangePlugin onChange={(editorState) => {
console.log(editorState);
}} />
<ImagePlugin />
</LexicalComposer>
);
}
Draft.js is a JavaScript rich text editor framework, built for React. It provides a set of tools for building rich text editors with support for various text formatting options, embedded media, and more. Compared to @lexical/rich-text, Draft.js is more mature and widely used but may have a steeper learning curve.
Slate is a completely customizable framework for building rich text editors. It provides a set of tools for creating complex text editing experiences with support for various text formatting options, embedded media, and more. Slate offers more flexibility and customization options compared to @lexical/rich-text but may require more effort to set up and configure.
Quill is a powerful, free, open-source WYSIWYG editor built for the modern web. It provides a rich API for building text editors with support for various text formatting options, embedded media, and more. Quill is easier to set up and use compared to @lexical/rich-text but may offer less flexibility and customization options.
@lexical/rich-text
This package provides a starting point for Lexical users by registering listeners for a set of basic commands that cover simple text-editing behavior such as entering text, deleting characters, copy + paste, or changing the selection with arrow keys. It also provides default behavior for rich text features, such as headings, formatted, text and blockquotes.
You can use this package as a starting point, and then add additional command listeners to customize the functionality of your editor. If you don't want or need rich text functionality, you may want to consider using @lexical/plain-text instead.
v0.17.1 (2024-08-26)
FAQs
This package contains rich text helpers for Lexical.
We found that @lexical/rich-text demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.