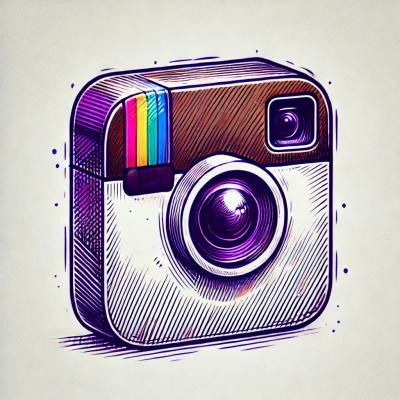
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
var Liara = require('@liara/sdk');
var liaraClient = new Liara.Storage.Client({
accessKey: 'Q3AM3UQ867SPQQA43P2F',
secretKey: 'zuf+tfteSlswRu7BJ86wekitnifILbZam1KYY3TG',
endPoint: '2aoassadfu234.storage.liara.ir',
});
new Liara.Storage.Client ({endPoint, accessKey, secretKey}) |
Initializes a new client object. |
Parameters
Param | Type | Description |
---|---|---|
endPoint | string | endPoint is a host name or an IP address. |
accessKey | string | accessKey is like user-id that uniquely identifies your account. |
secretKey | string | secretKey is the password to your account. |
Example
var Liara = require('@liara/sdk');
var liaraClient = new Liara.Storage.Client({
accessKey: 'Q3AM3UQ867SPQQA43P2F',
secretKey: 'zuf+tfteSlswRu7BJ86wekitnifILbZam1KYY3TG',
endPoint: '2aoassadfu234.storage.liara.ir',
});
Creates a new bucket.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
callback(err) | function | Callback function with err as the error argument. err is null if the bucket is successfully created. If no callback is passed, a Promise is returned. |
Example
liaraClient.makeBucket('mybucket', function(err) {
if (err) return console.log('Error creating bucket.', err)
console.log('Bucket created successfully.')
})
Lists all buckets.
Parameters
Param | Type | Description |
---|---|---|
callback(err, bucketStream) | function | Callback function with error as the first argument. bucketStream is the stream emitting bucket information. If no callback is passed, a Promise is returned. |
bucketStream emits Object with the format:-
Param | Type | Description |
---|---|---|
bucket.name | string | bucket name |
bucket.creationDate | Date | date when bucket was created. |
Example
liaraClient.listBuckets(function(err, buckets) {
if (err) return console.log(err)
console.log('buckets :', buckets)
})
Checks if a bucket exists.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
callback(err, exists) | function | exists is a boolean which indicates whether bucketName exists or not. err is set when an error occurs during the operation. |
Example
liaraClient.bucketExists('mybucket', function(err, exists) {
if (err) {
return console.log(err)
}
if (exists) {
return console.log('Bucket exists.')
}
})
Removes a bucket.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
callback(err) | function | err is null if the bucket is removed successfully. If no callback is passed, a Promise is returned. |
Example
liaraClient.removeBucket('mybucket', function(err) {
if (err) return console.log('unable to remove bucket.')
console.log('Bucket removed successfully.')
})
Lists all objects in a bucket.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
prefix | string | The prefix of the objects that should be listed (optional, default '' ). |
recursive | bool | true indicates recursive style listing and false indicates directory style listing delimited by '/'. (optional, default false ). |
Return Value
Param | Type | Description |
---|---|---|
stream | Stream | Stream emitting the objects in the bucket. |
The object is of the format:
Param | Type | Description |
---|---|---|
obj.name | string | name of the object. |
obj.prefix | string | name of the object prefix. |
obj.size | number | size of the object. |
obj.etag | string | etag of the object. |
obj.lastModified | Date | modified time stamp. |
Example
var stream = liaraClient.listObjects('mybucket','', true)
stream.on('data', function(obj) { console.log(obj) } )
stream.on('error', function(err) { console.log(err) } )
Lists all objects in a bucket using S3 listing objects V2 API
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
prefix | string | The prefix of the objects that should be listed (optional, default '' ). |
recursive | bool | true indicates recursive style listing and false indicates directory style listing delimited by '/'. (optional, default false ). |
startAfter | string | Specifies the object name to start after when listing objects in a bucket. (optional, default '' ). |
Return Value
Param | Type | Description |
---|---|---|
stream | Stream | Stream emitting the objects in the bucket. |
The object is of the format:
Param | Type | Description |
---|---|---|
obj.name | string | name of the object. |
obj.prefix | string | name of the object prefix. |
obj.size | number | size of the object. |
obj.etag | string | etag of the object. |
obj.lastModified | Date | modified time stamp. |
Example
var stream = liaraClient.listObjectsV2('mybucket','', true,'')
stream.on('data', function(obj) { console.log(obj) } )
stream.on('error', function(err) { console.log(err) } )
Lists partially uploaded objects in a bucket.
Parameters
Param | Type | Description |
---|---|---|
bucketname | string | Name of the bucket. |
prefix | string | Prefix of the object names that are partially uploaded. (optional, default '' ) |
recursive | bool | true indicates recursive style listing and false indicates directory style listing delimited by '/'. (optional, default false ). |
Return Value
Param | Type | Description |
---|---|---|
stream | Stream | Emits objects of the format listed below: |
Param | Type | Description |
---|---|---|
part.key | string | name of the object. |
part.uploadId | string | upload ID of the object. |
part.size | Integer | size of the partially uploaded object. |
Example
var Stream = liaraClient.listIncompleteUploads('mybucket', '', true)
Stream.on('data', function(obj) {
console.log(obj)
})
Stream.on('end', function() {
console.log('End')
})
Stream.on('error', function(err) {
console.log(err)
})
Downloads an object as a stream.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
objectName | string | Name of the object. |
callback(err, stream) | function | Callback is called with err in case of error. stream is the object content stream. If no callback is passed, a Promise is returned. |
Example
var size = 0
liaraClient.getObject('mybucket', 'photo.jpg', function(err, dataStream) {
if (err) {
return console.log(err)
}
dataStream.on('data', function(chunk) {
size += chunk.length
})
dataStream.on('end', function() {
console.log('End. Total size = ' + size)
})
dataStream.on('error', function(err) {
console.log(err)
})
})
Downloads the specified range bytes of an object as a stream.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
objectName | string | Name of the object. |
offset | number | offset of the object from where the stream will start. |
length | number | length of the object that will be read in the stream (optional, if not specified we read the rest of the file from the offset). |
callback(err, stream) | function | Callback is called with err in case of error. stream is the object content stream. If no callback is passed, a Promise is returned. |
Example
var size = 0
// reads 30 bytes from the offset 10.
liaraClient.getPartialObject('mybucket', 'photo.jpg', 10, 30, function(err, dataStream) {
if (err) {
return console.log(err)
}
dataStream.on('data', function(chunk) {
size += chunk.length
})
dataStream.on('end', function() {
console.log('End. Total size = ' + size)
})
dataStream.on('error', function(err) {
console.log(err)
})
})
Downloads and saves the object as a file in the local filesystem.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
objectName | string | Name of the object. |
filePath | string | Path on the local filesystem to which the object data will be written. |
callback(err) | function | Callback is called with err in case of error. If no callback is passed, a Promise is returned. |
Example
var size = 0
liaraClient.fGetObject('mybucket', 'photo.jpg', '/tmp/photo.jpg', function(err) {
if (err) {
return console.log(err)
}
console.log('success')
})
Uploads an object from a stream/Buffer.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
objectName | string | Name of the object. |
stream | Stream | Readable stream. |
size | number | Size of the object (optional). |
metaData | Javascript Object | metaData of the object (optional). |
callback(err, etag) | function | Non-null err indicates error, etag string is the etag of the object uploaded. If no callback is passed, a Promise is returned. |
Example
The maximum size of a single object is limited to 5TB. putObject transparently uploads objects larger than 5MiB in multiple parts. This allows failed uploads to resume safely by only uploading the missing parts. Uploaded data is carefully verified using MD5SUM signatures.
var Fs = require('fs')
var file = '/tmp/40mbfile'
var fileStream = Fs.createReadStream(file)
var fileStat = Fs.stat(file, function(err, stats) {
if (err) {
return console.log(err)
}
liaraClient.putObject('mybucket', '40mbfile', fileStream, stats.size, function(err, etag) {
return console.log(err, etag) // err should be null
})
})
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
objectName | string | Name of the object. |
string or Buffer | Stream or Buffer | Readable stream. |
metaData | Javascript Object | metaData of the object (optional). |
callback(err, etag) | function | Non-null err indicates error, etag string is the etag of the object uploaded. |
Example
var buffer = 'Hello World'
liaraClient.putObject('mybucket', 'hello-file', buffer, function(err, etag) {
return console.log(err, etag) // err should be null
})
Uploads contents from a file to objectName.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
objectName | string | Name of the object. |
filePath | string | Path of the file to be uploaded. |
metaData | Javascript Object | Metadata of the object. |
callback(err, etag) | function | Non-null err indicates error, etag string is the etag of the object uploaded. If no callback is passed, a Promise is returned. |
Example
The maximum size of a single object is limited to 5TB. fPutObject transparently uploads objects larger than 5MiB in multiple parts. This allows failed uploads to resume safely by only uploading the missing parts. Uploaded data is carefully verified using MD5SUM signatures.
var file = '/tmp/40mbfile'
var metaData = {
'Content-Type': 'text/html',
'Content-Language': 123,
'X-Amz-Meta-Testing': 1234,
'example': 5678
}
liaraClient.fPutObject('mybucket', '40mbfile', file, metaData, function(err, etag) {
return console.log(err, etag) // err should be null
})
Copy a source object into a new object in the specified bucket.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
objectName | string | Name of the object. |
sourceObject | string | Path of the file to be copied. |
conditions | CopyConditions | Conditions to be satisfied before allowing object copy. |
callback(err, {etag, lastModified}) | function | Non-null err indicates error, etag string and lastModified Date are the etag and the last modified date of the object newly copied. If no callback is passed, a Promise is returned. |
Example
var conds = new Minio.CopyConditions()
conds.setMatchETag('bd891862ea3e22c93ed53a098218791d')
liaraClient.copyObject('mybucket', 'newobject', '/mybucket/srcobject', conds, function(e, data) {
if (e) {
return console.log(e)
}
console.log("Successfully copied the object:")
console.log("etag = " + data.etag + ", lastModified = " + data.lastModified)
})
Gets metadata of an object.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
objectName | string | Name of the object. |
callback(err, stat) | function | err is not null in case of error, stat contains the object information listed below. If no callback is passed, a Promise is returned. |
Param | Type | Description |
---|---|---|
stat.size | number | size of the object. |
stat.etag | string | etag of the object. |
stat.metaData | Javascript Object | metadata of the object. |
stat.lastModified | Date | Last Modified time stamp. |
Example
liaraClient.statObject('mybucket', 'photo.jpg', function(err, stat) {
if (err) {
return console.log(err)
}
console.log(stat)
})
Removes an object.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
objectName | string | Name of the object. |
callback(err) | function | Callback function is called with non null value in case of error. If no callback is passed, a Promise is returned. |
Example
liaraClient.removeObject('mybucket', 'photo.jpg', function(err) {
if (err) {
return console.log('Unable to remove object', err)
}
console.log('Removed the object')
})
Remove all objects in the objectsList.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
objectsList | object | list of objects in the bucket to be removed. |
callback(err) | function | Callback function is called with non null value in case of error. |
Example
var objectsList = []
// List all object paths in bucket my-bucketname.
var objectsStream = s3Client.listObjects('my-bucketname', 'my-prefixname', true)
objectsStream.on('data', function(obj) {
objectsList.push(obj.name);
})
objectsStream.on('error', function(e) {
console.log(e);
})
objectsStream.on('end', function() {
s3Client.removeObjects('my-bucketname',objectsList, function(e) {
if (e) {
return console.log('Unable to remove Objects ',e)
}
console.log('Removed the objects successfully')
})
})
Removes a partially uploaded object.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
objectName | string | Name of the object. |
callback(err) | function | Callback function is called with non null value in case of error. If no callback is passed, a Promise is returned. |
Example
liaraClient.removeIncompleteUpload('mybucket', 'photo.jpg', function(err) {
if (err) {
return console.log('Unable to remove incomplete object', err)
}
console.log('Incomplete object removed successfully.')
})
Presigned URLs are generated for temporary download/upload access to private objects.
Generates a presigned URL for the provided HTTP method, 'httpMethod'. Browsers/Mobile clients may point to this URL to directly download objects even if the bucket is private. This presigned URL can have an associated expiration time in seconds after which the URL is no longer valid. The default value is 7 days.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
objectName | string | Name of the object. |
expiry | number | Expiry time in seconds. Default value is 7 days. (optional) |
reqParams | object | request parameters. (optional) |
requestDate | Date | A date object, the url will be issued at. Default value is now. (optional) |
callback(err, presignedUrl) | function | Callback function is called with non null err value in case of error. presignedUrl will be the URL using which the object can be downloaded using GET request. If no callback is passed, a Promise is returned. |
Example 1
// presigned url for 'getObject' method.
// expires in a day.
liaraClient.presignedUrl('GET', 'mybucket', 'hello.txt', 24*60*60, function(err, presignedUrl) {
if (err) return console.log(err)
console.log(presignedUrl)
})
Example 2
// presigned url for 'listObject' method.
// Lists objects in 'myBucket' with prefix 'data'.
// Lists max 1000 of them.
liaraClient.presignedUrl('GET', 'mybucket', '', 1000, {'prefix': 'data', 'max-keys': 1000}, function(err, presignedUrl) {
if (err) return console.log(err)
console.log(presignedUrl)
})
Generates a presigned URL for HTTP GET operations. Browsers/Mobile clients may point to this URL to directly download objects even if the bucket is private. This presigned URL can have an associated expiration time in seconds after which the URL is no longer valid. The default value is 7 days.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
objectName | string | Name of the object. |
expiry | number | Expiry time in seconds. Default value is 7 days. (optional) |
respHeaders | object | response headers to override (optional) |
requestDate | Date | A date object, the url will be issued at. Default value is now. (optional) |
callback(err, presignedUrl) | function | Callback function is called with non null err value in case of error. presignedUrl will be the URL using which the object can be downloaded using GET request. If no callback is passed, a Promise is returned. |
Example
// expires in a day.
liaraClient.presignedGetObject('mybucket', 'hello.txt', 24*60*60, function(err, presignedUrl) {
if (err) return console.log(err)
console.log(presignedUrl)
})
Generates a presigned URL for HTTP PUT operations. Browsers/Mobile clients may point to this URL to upload objects directly to a bucket even if it is private. This presigned URL can have an associated expiration time in seconds after which the URL is no longer valid. The default value is 7 days.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
objectName | string | Name of the object. |
expiry | number | Expiry time in seconds. Default value is 7 days. |
callback(err, presignedUrl) | function | Callback function is called with non null err value in case of error. presignedUrl will be the URL using which the object can be uploaded using PUT request. If no callback is passed, a Promise is returned. |
Example
// expires in a day.
liaraClient.presignedPutObject('mybucket', 'hello.txt', 24*60*60, function(err, presignedUrl) {
if (err) return console.log(err)
console.log(presignedUrl)
})
Allows setting policy conditions to a presigned URL for POST operations. Policies such as bucket name to receive object uploads, key name prefixes, expiry policy may be set.
Parameters
Param | Type | Description |
---|---|---|
policy | object | Policy object created by liaraClient.newPostPolicy() |
callback(err, {postURL, formData}) | function | Callback function is called with non null err value in case of error. postURL will be the URL using which the object can be uploaded using POST request. formData is the object having key/value pairs for the Form data of POST body. If no callback is passed, a Promise is returned. |
Create policy:
var policy = liaraClient.newPostPolicy()
Apply upload policy restrictions:
// Policy restricted only for bucket 'mybucket'.
policy.setBucket('mybucket')
// Policy restricted only for hello.txt object.
policy.setKey('hello.txt')
or
// Policy restricted for incoming objects with keyPrefix.
policy.setKeyStartsWith('keyPrefix')
var expires = new Date
expires.setSeconds(24 * 60 * 60 * 10)
// Policy expires in 10 days.
policy.setExpires(expires)
// Only allow 'text'.
policy.setContentType('text/plain')
// Only allow content size in range 1KB to 1MB.
policy.setContentLengthRange(1024, 1024*1024)
POST your content from the browser using superagent
:
liaraClient.presignedPostPolicy(policy, function(err, data) {
if (err) return console.log(err)
var req = superagent.post(data.postURL)
_.each(data.formData, function(value, key) {
req.field(key, value)
})
// file contents.
req.attach('file', '/path/to/hello.txt', 'hello.txt')
req.end(function(err, res) {
if (err) {
return console.log(err.toString())
}
console.log('Upload successful.')
})
})
Buckets are configured to trigger notifications on specified types of events and paths filters.
Fetch the notification configuration stored in the S3 provider and that belongs to the specified bucket name.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
callback(err, bucketNotificationConfig) | function | Callback function is called with non null err value in case of error. bucketNotificationConfig will be the object that carries all notification configurations associated to bucketName. If no callback is passed, a Promise is returned. |
Example
liaraClient.getBucketNotification('mybucket', function(err, bucketNotificationConfig) {
if (err) return console.log(err)
console.log(bucketNotificationConfig)
})
Upload a user-created notification configuration and associate it to the specified bucket name.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
bucketNotificationConfig | BucketNotification | Javascript object that carries the notification configuration. |
callback(err) | function | Callback function is called with non null err value in case of error. If no callback is passed, a Promise is returned. |
Example
// Create a new notification object
var bucketNotification = new Minio.NotificationConfig();
// Setup a new topic configuration
var arn = Minio.buildARN('aws', 'sns', 'us-west-2', '408065449417', 'TestTopic')
var topic = new Minio.TopicConfig(arn)
topic.addFilterSuffix('.jpg')
topic.addFilterPrefix('myphotos/')
topic.addEvent(Minio.ObjectReducedRedundancyLostObject)
topic.addEvent(Minio.ObjectCreatedAll)
// Add the topic to the overall notification object
bucketNotification.add(topic)
liaraClient.setBucketNotification('mybucket', bucketNotification, function(err) {
if (err) return console.log(err)
console.log('Success')
})
Remove the bucket notification configuration associated to the specified bucket.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket |
callback(err) | function | Callback function is called with non null err value in case of error. If no callback is passed, a Promise is returned. |
liaraClient.removeAllBucketNotification('my-bucketname', function(e) {
if (e) {
return console.log(e)
}
console.log("True")
})
Listen for notifications on a bucket. Additionally one can provider filters for prefix, suffix and events. There is no prior set bucket notification needed to use this API. This is an Minio extension API where unique identifiers are regitered and unregistered by the server automatically based on incoming requests.
Returns an EventEmitter
, which will emit a notification
event carrying the record.
To stop listening, call .stop()
on the returned EventEmitter
.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket |
prefix | string | Object key prefix to filter notifications for. |
suffix | string | Object key suffix to filter notifications for. |
events | Array | Enables notifications for specific event types. |
See here for a full example.
var listener = liaraClient.listenBucketNotification('my-bucketname', 'photos/', '.jpg', ['s3:ObjectCreated:*'])
listener.on('notification', function(record) {
// For example: 's3:ObjectCreated:Put event occurred (2016-08-23T18:26:07.214Z)'
console.log('%s event occurred (%s)', record.eventName, record.eventTime)
listener.stop()
})
Get the bucket policy associated with the specified bucket. If objectPrefix
is not empty, the bucket policy will be filtered based on object permissions
as well.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket |
callback(err, policy) | function | Callback function is called with non null err value in case of error. policy is the bucket policy. If no callback is passed, a Promise is returned. |
// Retrieve bucket policy of 'my-bucketname'
liaraClient.getBucketPolicy('my-bucketname', function(err, policy) {
if (err) throw err
console.log(`Bucket policy file: ${policy}`)
})
Set the bucket policy on the specified bucket. bucketPolicy is detailed here.
Parameters
Param | Type | Description |
---|---|---|
bucketName | string | Name of the bucket. |
bucketPolicy | string | bucket policy. |
callback(err) | function | Callback function is called with non null err value in case of error. If no callback is passed, a Promise is returned. |
// Set the bucket policy of `my-bucketname`
liaraClient.setBucketPolicy('my-bucketname', JSON.stringify(policy), function(err) {
if (err) throw err
console.log('Bucket policy set')
})
Set the HTTP/HTTPS request options. Supported options are agent
(http.Agent()) and tls related options ('agent', 'ca', 'cert', 'ciphers', 'clientCertEngine', 'crl', 'dhparam', 'ecdhCurve', 'honorCipherOrder', 'key', 'passphrase', 'pfx', 'rejectUnauthorized', 'secureOptions', 'secureProtocol', 'servername', 'sessionIdContext') documented here
// Do not reject self signed certificates.
liaraClient.setRequestOptions({rejectUnauthorized: false})
MIT © Liara
FAQs
Liara SDK for NodeJS
The npm package @liara/sdk receives a total of 3 weekly downloads. As such, @liara/sdk popularity was classified as not popular.
We found that @liara/sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.