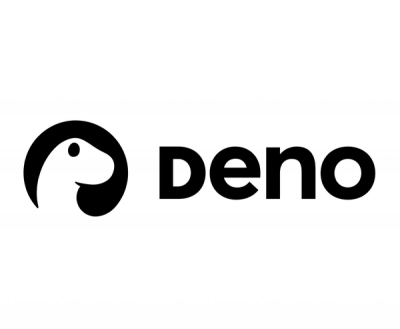
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@livechat/livechat-visitor-sdk
Advanced tools
Chat Widget Visitor SDK to perform a chat as a visitor
Chat Widget Visitor SDK allows you to perform a chat via LiveChat as a visitor using JavaScript.
You can use this to create your own chat widget.
If you need to customize the LiveChat widget, using Chat Widget Visitor SDK is one of the options to do this.
Keep in mind, however, that interacting with this API requires significant development skills.
If you only want to modify the look and feel of the chat widget, check our powerful yet easy to use chat widget configurator.
If you want to integrate the chat widget with your application, you can use the the existing JS API.
However, if you need a fully custom solution and you feel brave, dive into Chat Widget Visitor SDK: we provide methods and callbacks for deep integration with the LiveChat environment.
Chat Widget Visitor SDK is promise-based; all asynchronous methods return a promise. To get a promise fulfillment result, subscribe your handler to the promise's then
method. Check out this article to learn more about promises.
Important! Some methods and callbacks are not implemented yet.
You can use Chat Widget Visitor SDK in two different ways:
npm install --save @livechat/livechat-visitor-sdk
Now, you can import SDK in your code:
import * as LivechatVisitorSDK from "@livechat/livechat-visitor-sdk";
<script src="https://unpkg.com/@livechat/livechat-visitor-sdk@0.36.0/dist/livechat-visitor-sdk.min.js"></script>
If you just want to look around and play with the SDK, check out our sample chat widget implementation
Now run the init function with configuration, replacing LICENSE_NUMBER
with
your LiveChat license number. The function will return the visitorSDK interface:
const visitorSDK = LivechatVisitorSDK.init({
license: LICENSE_NUMBER,
})
With visitorSDK
, you can attach callbacks or execute
methods.
visitorSDK.on('new_message', newMessage => {
console.log(newMessage)
})
visitorSDK
.sendMessage({
text: 'Hello',
customId: 123423215,
})
.then(response => {
console.log(response)
})
.catch(error => {
console.log(error)
})
Initializes the chat window.
import LivechatVisitorSDK from '@livechat/livechat-visitor-sdk'
const visitorSDK = LivechatVisitorSDK.init({
license: 123,
group: 0,
})
param | type | description |
---|---|---|
license | number | LiveChat license number |
group | number | Group number (default: 0) |
Sends a message.
visitorSDK
.sendMessage({
text: 'Hello',
customId: '123423215',
})
.then(response => {
console.log(response)
})
.catch(error => {
console.log(error)
})
param | type | description |
---|---|---|
text | string | Visitor message text |
customId | string | Message custom id |
type | reason |
---|---|
connection | "Request failed" |
missing argument | "Missing text or customId parameter" |
state | "Chat is offline" |
Closes the chat.
visitorSDK
.closeChat()
.then(() => {
console.log('Chat is closed')
})
.catch(error => {
console.log(error)
})
This method has no parameters.
param | type | description |
---|---|---|
success | boolean | Request's response status |
type | reason |
---|---|
"state" | There is no chat to close |
"connection" | Request failed |
Enables file sharing through the chat window.
visitorSDK
.sendFile({
file: FileObject,
customId: '123423215',
})
.then(response => {
console.log(response.status)
})
.catch(error => {
console.log(error)
})
param | type | description |
---|---|---|
file | blob | File to upload |
onProgress | function | Callback function. It will receive a progress value (a number between 0 and 1) |
type | reason |
---|---|
connection | "Request failed" |
missing argument | "Missing file" |
wrong argument | "Cannot upload a file over 10MB" |
param | type | description |
---|---|---|
id | string | File id |
timestamp | string | File timestamp |
url | string | File URL address |
contentType | string | File content type |
size | number | File size in bytes |
width | number | Image width (for image content types) - optional |
height | number | Image height (for image content types) - optional |
Enables chat ratings.
visitorSDK
.rateChat({
rate: 'good',
comment: 'Agent helped me a lot!',
})
.then(response => {
console.log(response)
})
.catch(error => {
console.log(error)
})
param | type | description |
---|---|---|
rate | "good" \ "bad" \ "none" | Rate type |
comment | string | Rate comment text (optional) |
param | type | description |
---|---|---|
success | boolean | Request's response status |
type | reason |
---|---|
"missing argument" | Missing rate parameter |
"wrong argument" | Rate argument should be equal "good", "bad" or "none" |
"connection" | Request failed |
"connection" | Rate Comment request failed |
Marks a message as Seen.
Learn more about LiveChat delivery statuses here.
visitorSDK.markMessageAsSeen('123123123')
param | type | description |
---|---|---|
messageId | string | Id of message to be marked as seen |
Enables sneak peeks to see what the visitor is typing in before they actually send the message.
visitorSDK.setSneakPeek({
text: 'Hello, I woul',
})
param | type | description |
---|---|---|
text | string | Current message input text |
Note: Sneak peek won't be sent every time you call a function. It will be throttled (i.e. sent not earlier than 300ms after the last sneak peek request).
Sends chat transcripts to the specified email address when the chat is ended.
visitorSDK
.forwardChatTranscript({
email: 'test@livechatinc.com',
})
.then(response => {
console.log(response)
})
.catch(error => {
console.log(error)
})
param | type | description |
---|---|---|
string | Email that will automatically receive a transcript when a chat is finished |
param | type | description |
---|---|---|
success | boolean | Request's response status |
type | reason |
---|---|
"state" | There is no chat to forward transcript |
"missing argument" | Missing email parameter |
"connection" | Request failed |
Get post-chat survey form fields configured in chat window settings section in agent app.
visitorSDK
.getPostchatForm()
.then(data => {
console.log('Post-Chat form data', data)
})
.catch(error => {
console.log('error')
})
This method has no parameters.
param | type | description |
---|---|---|
fields | formField[] | Array with form fields details - see field's description below |
param | type | description |
---|---|---|
type | "text" / "select" / "checkbox" / "radio" / "information" | Type of the field |
required | boolean | Is field required? |
name | string | field's name |
label | string | Field's label |
value | string | Optional - field's value |
options | fieldOption[] | Array with fields options - only for fields of type: radio, checkbox, select, group_select. see option's description below |
param | type | description |
---|---|---|
label | string | input's label |
checked | boolean | input's checked flag |
value | string | input's value |
type | reason |
---|---|
"connection" | Request failed |
"state" | Post-chat survey is turned off |
Get pre-chat survey form fields configured in chat window settings section in agent app.
visitorSDK
.getPrechatForm()
.then(data => {
console.log('Pre-Chat form data', data)
})
.catch(error => {
console.log('error')
})
This method has no parameters.
param | type | description |
---|---|---|
fields | formField[] | Array with form fields details - see field's description below |
param | type | description |
---|---|---|
type | "name" / "text" / "email" / "select" / "checkbox" / "radio" / "group_select" / "information" | Type of the field |
required | boolean | Is field required? |
name | string | field's name |
label | string | Field's label |
value | string | Optional - field's value |
options | fieldOption[] | Array with fields options - only for fields of type: radio, checkbox, select, group_select. see option's description below |
param | type | description |
---|---|---|
label | string | input's label |
checked | boolean | input's checked flag |
value | string | input's value |
type | reason |
---|---|
"connection" | Request failed |
"state" | Pre-chat survey is turned off |
Collects the pre-chat survey form information (it will be visible during the chat and in the archives). Pre-chat form should be rendered using fields fetched by getPrechatForm method.
const formAnswers = {
'151913066848701614': 'Sidney Bechet', // "151913066848701614" is field's name, and "Sidney Bechet" is value provided by the visitor
'151913066848701615': 's.brechet@example.org',
'15191306684870388': ['1', '2'], // Fields with "checkbox" type have multiple values.
}
visitorSDK
.sendPrechatForm(formAnswers)
.then(() => {
console.log('Pre-chat sent')
})
.catch(error => {
console.log('error')
})
param | type | description |
---|---|---|
formAnswers | object | Pre-chat forms answers object - field.name: field.value |
param | type | description |
---|---|---|
success | boolean | Request's response status |
type | reason | fields |
---|---|---|
connection | "Request failed" | |
state | "You can't send prechat when chat is running" | |
wrong arguments | fieldError[] |
wrong arguments
error object contains additional array "fields" with detailed validations errors.
param | type | description |
---|---|---|
reason | string | Error reason, e.g. "Required field", "Wrong type" |
description | boolean | Optional. Detailed error description, e.g. "Pease fill in required field" |
field | string | Field's name |
Collects the post-chat form information (it will be visible in the archives).
const formAnswers = {
'151913066848701614': 'Good support!', // "151913066848701614" is field's name, and "Good support!" is value provided by the visitor
'15191306684870388': ['1', '2'], // Fields with "checkbox" type have multiple values.
}
visitorSDK
.sendPostchatForm(formAnswers)
.then(() => {
console.log('Pre-chat sent')
})
.catch(error => {
console.log('error')
})
param | type | description |
---|---|---|
formAnswers | object | Post-chat forms answers object - field.name: field.value |
param | type | description |
---|---|---|
success | boolean | Request's response status |
| type | reason | fields | | --------------- | -------------------------=-------------------- | ------------ | | connection | "Request failed" | | | state | "You can't send postchat when chat is running" | | | wrong arguments | | fieldError[] |
Collects the visitor information.
const visitorData = visitorSDK.getVisitorData().then(visitorData => {
console.log(visitorData)
})
param | type | description |
---|---|---|
name | string | Visitor's name |
string | Visitor's email address | |
pageUrl | string | Visitor's currently visiting website URL |
pageTitle | string | Visitor's currently visiting website title |
customProperties | object | Visitor's additional data object (custom properties) |
Set the visitor information.
visitorSDK.setVisitorData({
name: 'Wynton Marsalis',
email: 'test@livechatinc.com',
pageUrl: 'http://example.org/pricing',
pageTitle: 'Pricing',
customProperties: {
login: 'wyntonmarsalis',
customerId: '18260556127834',
},
})
param | type | description |
---|---|---|
name | string | Visitor's name |
string | Visitor's email address | |
pageUrl | string | Visitor's currently visiting website URL |
pageTitle | string | Visitor's currently visiting website title |
customProperties | object | Visitor's additional data object (custom properties) |
type | reason |
---|---|
missing argument | "Missing name, email, url or customProperties" |
connection | "Request failed" |
Get ticket form fields configured in chat window settings section in agent app.
visitorSDK
.getTicketForm()
.then(data => {
console.log('Ticket form data', data)
})
.catch(error => {
console.log('error')
})
This method has no parameters.
param | type | description |
---|---|---|
fields | formField[] | Array with form fields details - see field's description below |
param | type | description |
---|---|---|
type | "name" / "subject" / "email" / "header" | Type of the field |
required | boolean | Is field required? |
label | string | Field's label |
value | string | Optional - field's value |
Send ticket form filled in by visitor. Ticket form should be rendered using fields fetched by getTicketForm method.
visitorSDK
.sendTicketForm({
name: 'John',
email: 'john@example.org',
subject: 'App support',
message: 'I have a problem with your app',
})
.then(() => {
console.log('Ticket sent')
})
.catch(error => {
console.log('error')
})
param | type | description |
---|---|---|
name | string | Vistior's name |
string | Visitor's email address | |
subject | string | Ticket subject |
message | string | Visitor's message |
param | type | description |
---|---|---|
success | boolean | Request's response status |
type | reason |
---|---|
connection | "Request failed" |
missing argument | "Missing email" |
missing argument | "Missing message" |
Disconnect Visitor SDK. A visitor won't be tracked, and you won't be notified about agent's availability status. You will be automatically connected again after using sendMessage or setVisitorData methods.
visitorSDK.disconnect()
type | reason |
---|---|
state | "You can't disconnect during the chat" |
Disconnect Visitor SDK and unsubscribe from all callbacks.
visitorSDK.destroy()
Get translations for current group.
visitorSDK.getTranslations().then(translations => {
console.log('Translations', translations)
})
type | reason |
---|---|
connection | "Request failed" |
Get agent details without starting a chat
param | type | description |
---|---|---|
name | string | Agent's name |
id | string | Agent's ID |
avatarUrl | string | Agent's avatar - path to the image on Amazon s3 |
jobTitle | string | Agent's job title |
type | reason |
---|---|
connection | "Request failed" |
Get chat widget configuration
param | type | description |
---|---|---|
visitorData | object | Visitor's data |
features | object | Feature toggles and settings |
buttons | array | Buttons configuration |
theme | object | Theme settings |
domainWhitelist | array | Whitelisted domains |
widgetType | "embedded" / "popup" | Chat widget type |
integrations | object | Installed chat widget integrations |
language | string | Chat widget language |
groups | object | Groups details |
type | reason |
---|---|
connection | "Request failed" |
Start the chat
visitorSDK.startChat().then(data => {
console.log('data', data)
})
param | type | description |
---|---|---|
chatId | string | New Chat ID |
type | reason |
---|---|
connection | "Request failed" |
state | "You can't start new chat during chat" |
Callbacks let you bind a custom JavaScript function to an event. For example, your function can be executed every time agent's message has been received.
Callback function executed when server returns visitor's data.
visitorSDK.on('visitor_data', visitorData => {
console.log(visitorData)
})
param | type | description |
---|---|---|
id | string | Visitor ID |
Callback function executed when a new message arrives.
visitorSDK.on('new_message', newMessage => {
console.log(newMessage)
})
param | type | description |
---|---|---|
id | string | Message ID |
authorId | string | Message author ID |
timestamp | number | Timestamp added by server |
text | string | Message text |
chatId | string | Message chat ID |
customId | string | Message custom ID (for visitor's messages only) |
Callback function executed when a visitor is banned.
visitorSDK.on('visitor_banned', data => {
console.log(data)
})
Callback function executed when a chat is started.
visitorSDK.on('chat_started', chatData => {
console.log(chatData)
})
param | type | description |
---|---|---|
chatId | string | New chat ID |
Callback function executed when a chat is ended. This callback is called without any additional data.
visitorSDK.on('chat_ended', () => {
console.log('Chat is closed')
})
Callback function executed when the chat status is changed.
visitorSDK.on('status_changed', statusData => {
console.log(statusData)
})
param | type | description |
---|---|---|
status | "online" | "offline" | Current chat availability status |
Callback function executed when a visitor is queued.
visitorSDK.on('visitor_queued', queueData => {
console.log(queueData)
})
param | type | description |
---|---|---|
numberInQueue | number | Visitor's order number in queue |
waitingTime | number | Estimated waiting time in seconds |
Callback function executed when the connection status changes.
visitorSDK.on('connection_status_changed', statusData => {
console.log(statusData)
})
param | type | description |
---|---|---|
status | "connected" | "disconnected" | Current connection status |
Callback function executed when a file is shared.
visitorSDK.on('new_file', newFile => {
console.log(newFile)
})
param | type | description |
---|---|---|
id | string | File ID |
authorId | string | File author ID |
timestamp | number | Timestamp added by server |
url | string | File url |
contentType | string | File content type (i.e. 'text/plain') |
size | number | File size |
Callback function executed when an agent takes over the chat.
visitorSDK.on('agent_changed', newAgent => {
console.log(newAgent)
})
param | type | description |
---|---|---|
name | string | Agent's name |
id | string | Agent's ID |
avatarUrl | string | Agent's avatar - path to the image on Amazon s3 |
jobTitle | string | Agent's job title |
Callback function executed when the typing indicator appears.
visitorSDK.on('typing_indicator', typingData => {
console.log(typingData)
})
param | type | description |
---|---|---|
authorId | string | Author ID of the writer |
isTyping | boolean | Author is typing / stopped typing |
Callback function executed when a message is marked as seen.
Learn more about LiveChat delivery statuses here.
visitorSDK.on('message_seen', data => {
console.log(data)
})
param | type | description |
---|---|---|
id | string | Seen message id |
customId | string | Seen message custom id |
type | "agent" / "visitor" | Original message author type |
Callback function executed when the chat is rated or commented by visitor.
visitorSDK.on('chat_rated', data => {
console.log(messageData)
})
param | type | description |
---|---|---|
rate | "good" \ "bad" \ "none" | Rate type |
comment | string | Rate comment text |
Callback function executed when an invitation was sent to visitor.
visitorSDK.on('new_invitation', invitationData => {
console.log(invitationData)
})
param | type | description |
---|---|---|
id | string | Message ID |
authorId | string | Message author ID |
text | string | Message text |
receivedFirstTime | boolean | Was invitation received for the first time |
Callback executed when SDK finished initialization and all chat history was fetched. This callback is called without any additional data.
visitorSDK.on('ready', () => {
console.log('Visitor SDK is ready')
})
Subscribe to LiveChat Developers Newsletter to be notified about changes in Visitor SDK.
First public Release
FAQs
Chat Widget Visitor SDK to perform a chat as a visitor
The npm package @livechat/livechat-visitor-sdk receives a total of 517 weekly downloads. As such, @livechat/livechat-visitor-sdk popularity was classified as not popular.
We found that @livechat/livechat-visitor-sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.