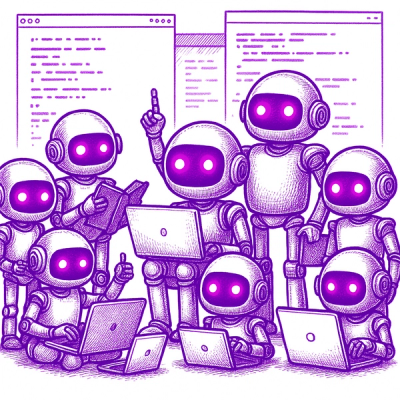
Security News
Open Source CAI Framework Handles Pen Testing Tasks up to 3,600× Faster Than Humans
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
@mapbox/vector-tile
Advanced tools
@mapbox/vector-tile is a library for working with vector tiles, which are a way to deliver geographic data in small chunks. This package allows you to parse and work with vector tiles, which are commonly used in mapping applications to render map data efficiently.
Parsing Vector Tiles
This code demonstrates how to parse a vector tile file and convert its features to GeoJSON format. It reads a vector tile from the file system, parses it using the VectorTile class, and iterates over its layers and features.
const fs = require('fs');
const { VectorTile } = require('@mapbox/vector-tile');
const Protobuf = require('pbf');
const data = fs.readFileSync('path/to/vector-tile.mvt');
const tile = new VectorTile(new Protobuf(data));
for (const layerName in tile.layers) {
const layer = tile.layers[layerName];
console.log(`Layer: ${layerName}`);
for (let i = 0; i < layer.length; i++) {
const feature = layer.feature(i);
console.log(feature.toGeoJSON(0, 0, 0));
}
}
Accessing Feature Properties
This code shows how to access the properties of a specific feature within a vector tile. It reads the vector tile, parses it, and then retrieves the properties of the first feature in a specified layer.
const fs = require('fs');
const { VectorTile } = require('@mapbox/vector-tile');
const Protobuf = require('pbf');
const data = fs.readFileSync('path/to/vector-tile.mvt');
const tile = new VectorTile(new Protobuf(data));
const layer = tile.layers['layer-name'];
const feature = layer.feature(0);
console.log(feature.properties);
Converting Features to GeoJSON
This code demonstrates how to convert a feature from a vector tile to GeoJSON format. It reads and parses the vector tile, retrieves a feature from a specified layer, and converts it to GeoJSON.
const fs = require('fs');
const { VectorTile } = require('@mapbox/vector-tile');
const Protobuf = require('pbf');
const data = fs.readFileSync('path/to/vector-tile.mvt');
const tile = new VectorTile(new Protobuf(data));
const layer = tile.layers['layer-name'];
const feature = layer.feature(0);
const geojson = feature.toGeoJSON(0, 0, 0);
console.log(JSON.stringify(geojson, null, 2));
geojson-vt is a library for slicing GeoJSON data into vector tiles on the fly in the browser or on the server. While it focuses more on creating vector tiles from GeoJSON data rather than parsing existing vector tiles, it can be used in conjunction with @mapbox/vector-tile for a complete solution for working with vector tiles.
tilelive is a library for working with tiles, including vector tiles, in Node.js. It provides a range of tools for reading, writing, and transforming tiles. While it offers broader functionality than @mapbox/vector-tile, it can be more complex to use for simple vector tile parsing tasks.
This library reads Mapbox Vector Tiles and allows access to the layers and features.
import {VectorTile} from '@mapbox/vector-tile';
import Protobuf from 'pbf';
const tile = new VectorTile(new Protobuf(data));
// Contains a map of all layers
tile.layers;
const landuse = tile.layers.landuse;
// Amount of features in this layer
landuse.length;
// Returns the first feature
landuse.feature(0);
Vector tiles contained in serialtiles-spec are gzip-encoded, so a complete example of parsing them with the native zlib module would be:
import {VectorTile} from '@mapbox/vector-tile';
import Protobuf from 'pbf';
import {gunzipSync} from 'zlib';
const buffer = gunzipSync(data);
const tile = new VectorTile(new Protobuf(buffer));
To install:
npm install @mapbox/vector-tile
An object that parses vector tile data and makes it readable.
layers
property. Optionally accepts end index.{<name>: <layer>, ...}
,
where each layer is a VectorTileLayer
object.An object that contains the data for a single vector tile layer.
Number
, default: 1
)String
) — layer nameNumber
, default: 4096
) — tile extent sizeNumber
) — number of features in the layerVectorTileFeature
) by the given index from the layer.An object that contains the data for a single feature.
Number
) — type of the feature (also see VectorTileFeature.types
)Number
) — feature extent sizeNumber
) — feature identifier, if presentObject
) — object literal with feature propertiesx
and y
properties)[x1, y1, x2, y2]
x
, y
, and z
refer to the containing tile's index.)FAQs
Parses vector tiles
The npm package @mapbox/vector-tile receives a total of 1,419,742 weekly downloads. As such, @mapbox/vector-tile popularity was classified as popular.
We found that @mapbox/vector-tile demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 28 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.