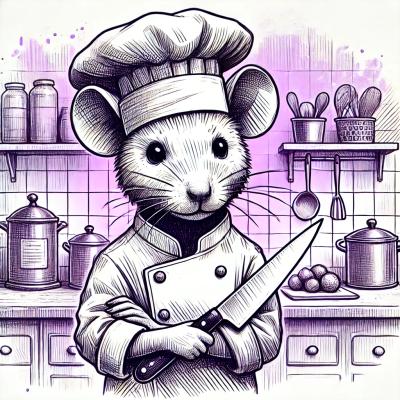
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@maplibre/maplibre-gl-compare
Advanced tools
Swipe and sync between two MapLibre maps. This plugin was originally developed for Mapbox (https://github.com/mapbox/mapbox-gl-compare) and was migrated to MapLibre after Mapbox changed its license.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>Swipe between maps</title>
<meta
name="viewport"
content="initial-scale=1,maximum-scale=1,user-scalable=no"
/>
<script src="../.env"></script>
<script src="https://unpkg.com/maplibre-gl@1.14.0-rc.1/dist/maplibre-gl.js"></script>
<link
href="https://unpkg.com/maplibre-gl@1.14.0-rc.1/dist/maplibre-gl.css"
rel="stylesheet"
/>
<style>
body {
margin: 0;
padding: 0;
}
#map {
position: absolute;
top: 0;
bottom: 0;
width: 100%;
}
</style>
</head>
<body>
<style>
body {
overflow: hidden;
}
body * {
-webkit-touch-callout: none;
-webkit-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
.map {
position: absolute;
top: 0;
bottom: 0;
width: 100%;
}
</style>
<script src="maplibre-gl-compare.js"></script>
<link rel="stylesheet" href="maplibre-gl-compare.css" type="text/css" />
<div id="comparison-container">
<div id="before" class="map"></div>
<div id="after" class="map"></div>
</div>
<script>
var beforeMap = new maplibregl.Map({
container: "before",
style: "https://demotiles.maplibre.org/style.json",
center: [7.221275, 50.326111],
zoom: 5,
});
var afterMap = new maplibregl.Map({
container: "after",
style:
"https://vectortiles.geo.admin.ch/styles/ch.swisstopo.leichte-basiskarte.vt/style.json",
center: [7.221275, 50.326111],
zoom: 5,
});
// A selector or reference to HTML element
var container = "#comparison-container";
var map = new maplibregl.Compare(beforeMap, afterMap, container, {
// Set this to enable comparing two maps by mouse movement:
// m ousemove: true
});
</script>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title>Swipe between maps</title>
<meta
name="viewport"
content="initial-scale=1,maximum-scale=1,user-scalable=no"
/>
<script src="../.env"></script>
<script src="https://unpkg.com/maplibre-gl@1.14.0-rc.1/dist/maplibre-gl.js"></script>
<link
href="https://unpkg.com/maplibre-gl@1.14.0-rc.1/dist/maplibre-gl.css"
rel="stylesheet"
/>
<style>
body {
margin: 0;
padding: 0;
}
#map {
position: absolute;
top: 0;
bottom: 0;
width: 100%;
}
</style>
</head>
<body>
<style>
body {
overflow: hidden;
}
body * {
-webkit-touch-callout: none;
-webkit-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
}
.map {
position: absolute;
top: 0;
bottom: 0;
width: 100%;
}
</style>
<script src="maplibre-gl-compare.js"></script>
<link rel="stylesheet" href="maplibre-gl-compare.css" type="text/css" />
<div id="comparison-container">
<div id="before" class="map"></div>
<div id="after" class="map"></div>
</div>
<script>
var beforeMap = new maplibregl.Map({
container: "before",
style:
"https://api.maptiler.com/maps/hybrid/style.json?key=get_your_own_OpIi9ZULNHzrESv6T2vL",
center: [7.221275, 50.326111],
zoom: 15,
});
var afterMap = new maplibregl.Map({
container: "after",
style:
"https://api.maptiler.com/maps/streets/style.json?key=get_your_own_OpIi9ZULNHzrESv6T2vL",
center: [7.221275, 50.326111],
zoom: 15,
});
// A selector or reference to HTML element
var container = "#comparison-container";
var map = new maplibregl.Compare(beforeMap, afterMap, container, {
// Set this to enable comparing two maps by mouse movement:
// m ousemove: true
});
</script>
</body>
</html>
var beforeMap = new maplibregl.Map({
container: "before",
style: "https://demotiles.maplibre.org/style.json",
center: [7.221275, 50.326111],
zoom: 5,
});
var afterMap = new maplibregl.Map({
container: "after",
style:
"https://vectortiles.geo.admin.ch/styles/ch.swisstopo.leichte-basiskarte.vt/style.json",
center: [7.221275, 50.326111],
zoom: 5,
});
// A selector or reference to HTML element
var container = '#comparison-container';
var map = new maplibregl.Compare(beforeMap, afterMap, container, {
mousemove: true, // Optional. Set to true to enable swiping during cursor movement.
orientation: 'vertical' // Optional. Sets the orientation of swiper to horizontal or vertical, defaults to vertical
});
compare = new mapboxgl.Compare(beforeMap, afterMap, container, {
mousemove: true, // Optional. Set to true to enable swiping during cursor movement.
orientation: 'vertical' // Optional. Sets the orientation of swiper to horizontal or vertical, defaults to vertical
});
// Get Current position - this will return the slider's current position, in pixels
compare.currentPosition;
// Set Position - this will set the slider at the specified (x) number of pixels from the left-edge or top-edge of viewport based on swiper orientation
compare.setSlider(x);
//Listen to slider movement - and return current position on each slideend
compare.on('slideend', (e) => {
console.log(e.currentPosition);
});
//Remove - this will remove the compare control from the DOM and stop synchronizing the two maps.
compare.remove();
Compare swisstopo with demotiles
Add tags referencing maplibre-gl-compare
after adding maplibre-gl
to your website:
<!-- MapLibre GL -->
<link href="https://unpkg.com/maplibre-gl@1.14.0/dist/maplibre-gl.css" rel='stylesheet' />
<link href="maplibre-gl-compare.css" rel='stylesheet' />
<script src="https://unpkg.com/maplibre-gl@1.14.0/dist/maplibre-gl.js"></script>
<script src="maplibre-gl-compare.js"></script>
This project makes it possible to easily compare two maps.
Please use the issue tracker to report any bugs or file feature requests.
FAQs
Swipe and sync between two MapLibre maps
The npm package @maplibre/maplibre-gl-compare receives a total of 245 weekly downloads. As such, @maplibre/maplibre-gl-compare popularity was classified as not popular.
We found that @maplibre/maplibre-gl-compare demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.