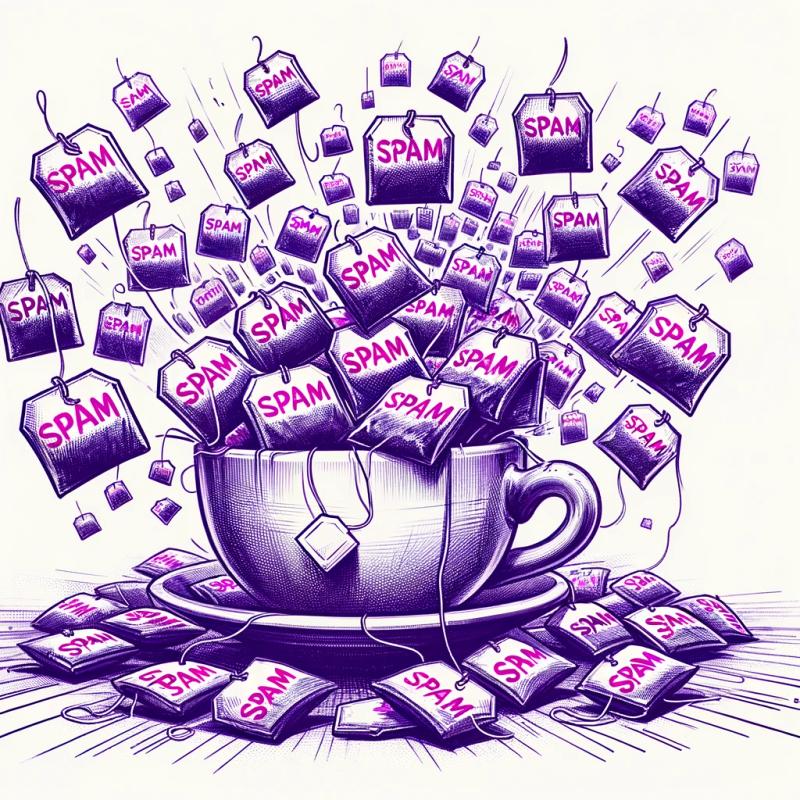
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@mariolazzari/nasa-api
Advanced tools
Readme
This package is a TypeScript based wrapper around the public NASA REST APIs.
Prerequisites
In order to use this package, you need an api key: You can read more on how to obtain the API key on this page.
This package requires NodeJS (version 18 or later) and a node package manager (Npm, Yarn, Pnpm or Bun).
To make sure you have them available on your machine, try running the following command.
$ npm -v && node -v
v10.1.0
v18.18.0
These instructions will get you a copy of the project up and running on your local machine for development and testing purposes. See deployment for notes on how to deploy the project on a live system.
BEFORE YOU INSTALL: please read the prerequisites.
Start with cloning this repo on your local machine:
$ git clone https://github.com/mariolazzari/nasa-api.git
$ cd nasa
To install and set up the library, run:
npm install @mariolazzari/nasa-api
Import package
import { Nasa } from "@mariolazzari/nasa-api"
Watch mode
npm test
Unit testing
npm test
Bulding new version
npm build
This task will create a distribution version of the project inside your local dist/ folder
Nasa class content handles all the requests and the responses to the three main Rijks museum REST APIs.
In order to initialize Nasa client:
const nasa = new Nasa(NASA_API_KEY)
Constructor parameters
Parameter | Type | Required | Default |
---|---|---|---|
apiKey | string | Yes |
Nasa client includes the following three methods:
Description
This asynchronous method handles GET /planetary/apod
REST API, in order to return the astronomical picture of the day for selected day (current day by default).
Prototype
async apodDate(date: Date = new Date()): Promise<Result<Apod>>
Sample code
const date = new Date(2023, 2, 28);
const apod: Result<Apod> = await nasa.apodDate(date);
Description
This asynchronous method handles GET /planetary/apod
REST API, in order to return the astronomical pictures of the day for selected date range.
Prototype
async apodDates(from: Date = new Date(), to: Date = new Date()): Promise<Result<Apod[]>>
Sample code
const from = new Date(2023, 2, 21);
const to = new Date(2023, 2, 8);
const apods: Result<Apod[]> = await nasa.apodDates(from, to);
Description
This asynchronous method handles GET /planetary/apod
REST API, in order to return n random astronomical pictures of the day (10 pictures by default).
Prototype
async apodRandom(n:number = 10): Promise<Result<Apod[]>>
Sample code
const apods: Result<Apod[]> = await nasa.apodRandom(10);
Description
This asynchronous method handles GET /neo/rest/v1/feed
REST API, in order to return the near Earth objects for selected dates range.
Prototype
async neoFeed(from: Date, to: Date): Promise<Result<NeoResponse>>
Sample code
const from = new Date(2023, 2, 21);
const to = new Date(2023, 2, 8);
const neos: Result<NeoResponse> = await nasa.neoFeed(from, to);
Description
This asynchronous method handles GET /neo/rest/v1/neo/:id
REST API, in order to return the near Earth objects for selected asteroid ID, including its orbital data.
Prototype
async neolookup(asteroidId:number): Promise<Result<Neo & Link>>
Sample code
const asteroidId = 3542519
const neo: Result<Neo & Link> = await nasa.neoLookup(asteroidId);
Description
This asynchronous method handles GET /DONKI/CME
REST API, in order to return the coronal mass ejection (CME) for selected dates range.
Prototype
async donkiCme(from: Date, to: Date): Promise<Result<CoronalMassEjection[]>>
Method parameters
Parameter | Type | Required | Default |
---|---|---|---|
from | Date | No | 30 days before |
to | Date | No | today |
Sample code
const from = new Date(2023, 2, 21);
const to = new Date(2023, 2, 8);
const cme: Result<CoronalMassEjection[]> = await nasa.donkiCme(from, to);
Description
This asynchronous method handles GET /DONKI/CMEAnalysis
REST API, in order to return the coronal mass ejection (CME) analysis for selected dates range.
Prototype
async donkiCme(from: Date, to: Date, mostAccurateOnly: boolean, completeEntryOnly: boolean, speed:number ): Promise<Result<CoronalMassEjectionAnalysis[]>>
Method parameters
Parameter | Type | Required | Default |
---|---|---|---|
from | Date | No | 30 days before |
to | Date | No | today |
mostAccurateOnly | boolean | No | true |
completeEntryOnly | boolean | No | true |
speed | number | No | 0 |
Sample code
const from = new Date(2023, 2, 21);
const to = new Date(2023, 2, 8);
const cmeAnalysis: Result<CoronalMassEjectionAnalysis>[] = await nasa.donkiCmeAnalysis(from, to);
Description
This asynchronous method handles GET /DONKI/GST
REST API, in order to return the Geomagnetic Storm (GST) for selected dates range.
Prototype
async donkiGst(from: Date, to: Date ): Promise<Result<GeomagneticStorm[]>>
Method parameters
Parameter | Type | Required | Default |
---|---|---|---|
from | Date | No | 30 days before |
to | Date | No | today |
Sample code
const from = new Date(2023, 2, 21);
const to = new Date(2023, 2, 8);
const gst: Result<GeomagneticStorm[]> = await nasa.donkiCme(from, to);
Description
This asynchronous method handles GET /DONKI/IPS
REST API, in order to return the Interplanetary Shock (IPS) for selected dates range.
Prototype
async donkiIps(from: Date, to: Date ): Promise<Result<InterplanetaryShock[]>>
Method parameters
Parameter | Type | Required | Default |
---|---|---|---|
from | Date | No | 30 days before |
to | Date | No | today |
Sample code
const from = new Date(2023, 2, 21);
const to = new Date(2023, 2, 8);
const ips: Result<InterplanetaryShock[]> = await nasa.donkiIps(from, to);
Description
This asynchronous method handles GET /DONKI/FLR
REST API, in order to return the Solar Flare (FLR) for selected dates range.
Prototype
async donkiFlr(from: Date, to: Date ): Promise<Result<SolarFlare[]>>
Method parameters
Parameter | Type | Required | Default |
---|---|---|---|
from | Date | No | 30 days before |
to | Date | No | today |
Sample code
const from = new Date(2023, 2, 21);
const to = new Date(2023, 2, 8);
const ips: Result<SolarFlare[]> = await nasa.donkiIps(from, to);
Description
This asynchronous method handles GET /DONKI/SEP
REST API, in order to return the Solar Energetic Particle (SEP) for selected dates range.
Prototype
async donkiSep(from: Date, to: Date ): Promise<Result<SolarEnergeticParticle[]>>
Method parameters
Parameter | Type | Required | Default |
---|---|---|---|
from | Date | No | 30 days before |
to | Date | No | today |
Sample code
const from = new Date(2023, 2, 21);
const to = new Date(2023, 2, 8);
const sep: Result<SolarEnergeticParticle[]> = await nasa.donkiSep(from, to);
Description
This asynchronous method handles GET /DONKI/MPC
REST API, in order to return the Magnetopause Crossing (MPC) for selected dates range.
Prototype
async donkiMpc(from: Date, to: Date ): Promise<Result<MagnetopauseCrossing[]>>
Method parameters
Parameter | Type | Required | Default |
---|---|---|---|
from | Date | No | 30 days before |
to | Date | No | today |
Sample code
const from = new Date(2023, 2, 21);
const to = new Date(2023, 2, 8);
const sep: Result<MagnetopauseCrossing[]> = await nasa.donkiMpc(from, to);
Description
This asynchronous method handles GET /DONKI/RBE
REST API, in order to return the Radiation Belt Enhancement (RBE) for selected dates range.
Prototype
async donkiRbe(from: Date, to: Date ): Promise<Result<RadiationBeltEnhancement[]>>
Method parameters
Parameter | Type | Required | Default |
---|---|---|---|
from | Date | No | 30 days before |
to | Date | No | today |
Sample code
const from = new Date(2023, 2, 21);
const to = new Date(2023, 2, 8);
const sep: Result<RadiationBeltEnhancement> = await nasa.donkiRbe(from, to);
Description
This asynchronous method handles GET /DONKI/HSS
REST API, in order to return the Hight Speed Stream (HSS) for selected dates range.
Prototype
async donkiRbe(from: Date, to: Date ): Promise<Result<HightSpeedStream[]>>
Method parameters
Parameter | Type | Required | Default |
---|---|---|---|
from | Date | No | 30 days before |
to | Date | No | today |
Sample code
const from = new Date(2023, 2, 21);
const to = new Date(2023, 2, 8);
const sep: Result<HightSpeedStream[]> = await nasa.donkiHss(from, to);
Description
This asynchronous method handles GET /DONKI/WSAEnlilSimulations
REST API, in order to return the WSA+EnlilSimulation for selected dates range.
Prototype
async donkiWsa(from: Date, to: Date ): Promise<Result<WsaEnlilSimulation[]>>
Method parameters
Parameter | Type | Required | Default |
---|---|---|---|
from | Date | No | 30 days before |
to | Date | No | today |
Sample code
const from = new Date(2023, 2, 21);
const to = new Date(2023, 2, 8);
const sep: Result<WsaEnlilSimulation[]> = await nasa.donkiWsa(from, to);
Description
This asynchronous method handles GET /DONKI/notifications
REST API, in order to return the Notifications for selected dates range.
Prototype
async donkiNotifications(from: Date, to: Date ): Promise<Result<Notification[]>>
Method parameters
Parameter | Type | Required | Default |
---|---|---|---|
from | Date | No | 30 days before |
to | Date | No | today |
type | NotificationType | no | all |
Sample code
const from = new Date(2023, 2, 21);
const to = new Date(2023, 2, 8);
const sep: Result<Notification[]> = await nasa.donkiNotifications(from, to);
FAQs
NASA public REST API client
The npm package @mariolazzari/nasa-api receives a total of 0 weekly downloads. As such, @mariolazzari/nasa-api popularity was classified as not popular.
We found that @mariolazzari/nasa-api demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.