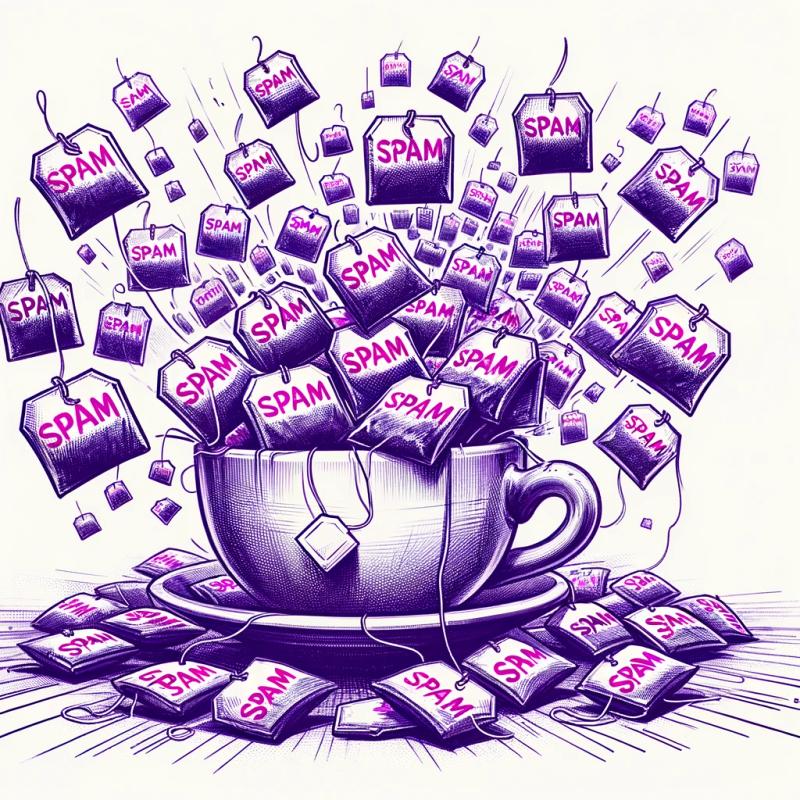
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@mini-apps/navigation-react
Advanced tools
Readme
React implementation of @mini-apps/navigation
yarn add @mini-apps/navigation-react
or
npm i @mini-apps/navigation-react
Firstly, you have to create instance of BrowserNavigator
from
@mini-apps/navigation
:
import {BrowserNavigator as Navigator} from '@mini-apps/navigation';
const navigator = new Navigator();
Then, you should import BrowserNavigator
component from
@mini-apps/navigation-react
and pass created navigator:
import React, {useEffect, useMemo} from 'react';
import {BrowserNavigator} from '@mini-apps/navigation-react';
// App component will be shown later
import {App} from './App';
import {
BrowserNavigator as Navigator,
extractInitOptions,
} from '@mini-apps/navigation';
function Root() {
// Create navigator
const navigator = useMemo(() => new Navigator(), []);
// Initialize it with extracted from browser settings
useEffect(() => {
const settings = extractInitOptions();
navigator.init(settings || undefined);
// We could pass any data we need in init. Moreover, we could just
// call mount() if extracting initial navigator state is not required
}, [navigator]);
return (
<BrowserNavigator navigator={navigator}>
<App/>
</BrowserNavigator>
);
}
To make routing work, we could use native browser a
tags along with
createLink
from @mini-apps/navigation
which creates appropriate links
for navigator with something like this:
import React from 'react';
import {createLink} from '@mini-apps/navigation';
function SomeBanner() {
return (
<div>
<a href={createLink({view: 'promo'})}>Go to promo</a>
<a href={createLink({view: '', modifiers: ['back']})}>Go back</a>
</div>
);
}
When using this way of defining links, make sure you already created and
initialized BrowserNavigator
instance, because otherwise, nothing will
watch for history updates and as a result, nothing happens.
Nevertheless, using default a
tags has a big defect. Clicking these links
will make browser splice all history items after current history element. So,
you will lose part of history, but BrowserNavigator
will keep working fine
and correct.
We recommend more comfortable and stable way of defining links like this:
import React from 'react';
import {Link} from '@mini-apps/navigation-react';
function SomeBanner() {
return (
<div>
<Link state={{view: 'promo'}}>
<a>Go to promo</a>
</Link>
<Link state={{view: 'promo-2'}} replace={true}>
<a>Replace with another promo</a>
</Link>
<Link back={true}>
<a>Go back</a>
</Link>
</div>
);
}
It looks much better and has a good benefit. When back
property is passed,
Go back
link will not make browser cut its history. Internally,
navigator.back()
is called.
Link
component is just passing props href
and onClick
(onClick
is just
extended, original callback is not lost) to child component
import React, {useEffect, useMemo} from 'react';
import {
BrowserNavigator as Navigator,
extractInitOptions,
} from '@mini-apps/navigation';
import {
BrowserNavigator,
Link,
useHistory,
useNavigatorState,
useNavigator,
} from '@mini-apps/navigation-react';
export function App() {
const navigator = useNavigator();
const state = useNavigatorState();
const history = useHistory();
return (
<div>
<p>Current history</p>
<div>
{history.map((s, idx) => {
const stringified = JSON.stringify(s);
let content = s === state
? <b>{stringified}</b>
: stringified;
return <div key={idx}>{content}</div>;
})}
</div>
<button onClick={() => navigator.back()}>Back</button>
<button onClick={() => navigator.forward()}>Forward</button>
<Link state={{view: 'main'}}>
<a>Link to main</a>
</Link>
<Link state={{view: 'main', popup: 'delete-user'}} oneTime={true}>
<a>Show one time popup which prompts for user delete</a>
</Link>
<Link state={{view: 'onboarding'}} replace={true}>
<a>Replace current state with onboarding</a>
</Link>
<Link back={true}>
<a>Link to previous state (Back button alternative)</a>
</Link>
</div>
);
}
function Root() {
// Create navigator
const navigator = useMemo(() => new Navigator(), []);
// Initialize it with extracted from browser settings
useEffect(() => {
const settings = extractInitOptions();
navigator.init(settings || undefined);
// We could pass any data we need in init. Moreover, we could just
// call mount() if extracting initial navigator state is not required
}, [navigator]);
return (
<BrowserNavigator navigator={navigator}>
<App/>
</BrowserNavigator>
);
}
FAQs
React wrapper for @mini-apps/navigation
The npm package @mini-apps/navigation-react receives a total of 6 weekly downloads. As such, @mini-apps/navigation-react popularity was classified as not popular.
We found that @mini-apps/navigation-react demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.