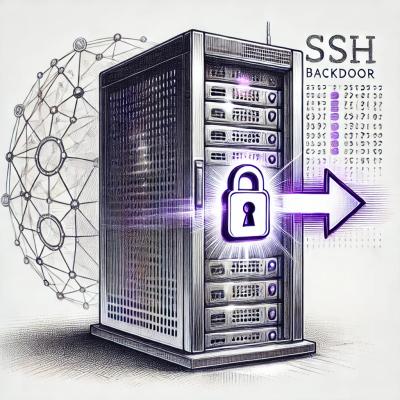
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@mionkit/client
Advanced tools
Fully typed client for mion Apis
@mionkit/client
Modern client for mion Apis:
To be able to use the client the server must register a couple of routes required by the client to request remote methods metadata for validation and serialization. This routes are part of the @mionkit/commons
package.
It is also required to export the type of the registered routes in the server.
// examples/server.routes.ts
import {RpcError} from '@mionkit/core';
import {Routes, registerRoutes} from '@mionkit/router';
import {clientRoutes} from '@mionkit/common';
import {Logger} from 'Logger';
export type User = {id: string; name: string; surname: string};
export type Order = {id: string; date: Date; userId: string; totalUSD: number};
const routes = {
auth: {
headerName: 'authorization',
headerHook: (ctx, token: string): void | RpcError => {
if (!token) return new RpcError({statusCode: 401, message: 'Not Authorized', name: ' Not Authorized'});
},
},
users: {
getById: (ctx, id: string): User | RpcError => ({id, name: 'John', surname: 'Smith'}),
delete: (ctx, id: string): string | RpcError => id,
create: (ctx, user: Omit<User, 'id'>): User | RpcError => ({id: 'USER-123', ...user}),
},
orders: {
getById: (ctx, id: string): Order | RpcError => ({id, date: new Date(), userId: 'USER-123', totalUSD: 120}),
delete: (ctx, id: string): string | RpcError => id,
create: (ctx, order: Omit<Order, 'id'>): Order | RpcError => ({id: 'ORDER-123', ...order}),
},
utils: {
sum: (ctx, a: number, b: number): number => a + b,
sayHello: (ctx, user: User): string => `Hello ${user.name} ${user.surname}`,
},
log: {
forceRunOnError: true,
hook: (ctx): any => {
Logger.log(ctx.path, ctx.request.headers, ctx.request.body);
},
},
} satisfies Routes;
// init server or serverless router
// initHttpRouter(...);
// initAwsLambdaRouter(...);
// register routes and exporting the type of the Api to be used by client
const myApi = registerRoutes(routes);
export type MyApi = typeof myApi;
// register routes required by client, (these routes serve metadata, for validation and serialization)
registerRoutes(clientRoutes);
To use the client we just need to import the type of the registered routes, and initialize the client.
The methods
object returned when initializing the client is a fully typed `RemoteApi`` object that contains all the remote methods with parameter types and return values.
// examples/client.ts
import {initClient} from '@mionkit/client';
// importing type only from server
import type {MyApi} from './server.routes';
import {ParamsValidationResponse} from '@mionkit/reflection';
const port = 8076;
const baseURL = `http://localhost:${port}`;
const {methods, client} = initClient<MyApi>({baseURL});
// prefills the token for any future requests, value is stored in localStorage
await methods.auth('myToken-XYZ').prefill();
// calls sayHello route in the server
const sayHello = await methods.utils.sayHello({id: '123', name: 'John', surname: 'Doe'}).call();
console.log(sayHello); // Hello John Doe
// calls sumTwo route in the server
const sumTwoResp = await methods.utils.sum(5, 2).call();
console.log(sumTwoResp); // 7
// validate parameters locally without calling the server
const validationResp: ParamsValidationResponse = await methods.utils
.sayHello({id: '123', name: 'John', surname: 'Doe'})
.validate();
console.log(validationResp); // {hasErrors: false, totalErrors: 0, errors: []}
When a remote route call fails, it always throws a RpcError
this can be the error from the route or any other error thrown from the route's hooks.
All the methods
operations: call
, validate
, prefill
, removePrefill
are async and throw a RpcError
if something fails including validation and serialization.
As catch blocks are always of type any
, the Type guard isRpcError
can be used to check the correct type of the error.
// examples/handling-errors.ts
import {initClient} from '@mionkit/client';
// importing type only from server
import type {MyApi} from './server.routes';
import {isRpcError, RpcError} from '@mionkit/core';
const port = 8076;
const baseURL = `http://localhost:${port}`;
const {methods, client} = initClient<MyApi>({baseURL});
try {
// calls sayHello route in the server
const sayHello = await methods.utils.sayHello({id: '123', name: 'John', surname: 'Doe'}).call();
console.log(sayHello); // Hello John Doe
} catch (error: RpcError | any) {
// in this case the request has failed because the authorization hook is missing
console.log(error); // {statusCode: 400, name: 'Validation Error', message: `Invalid params for Route or Hook 'auth'.`}
if (isRpcError(error)) {
// ... handle the error as required
}
}
try {
// Validation throws an error when validation fails
const sayHello = await methods.utils.sayHello(null as any).validate();
console.log(sayHello); // Hello John Doe
} catch (error: RpcError | any) {
console.log(error); // { statusCode: 400, name: 'Validation Error', message: `Invalid params ...`, errorData : {...}}
}
MIT LICENSE
FAQs
Browser client for mion Apps.
We found that @mionkit/client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.