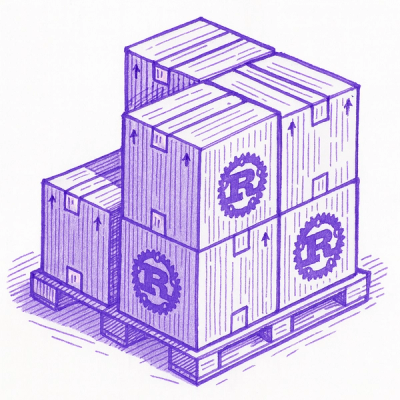
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
@moyal/js-test
Advanced tools
A lightweight, dependency-free JavaScript testing utility. This project is framework-agnostic and usable in both browser and Node.js environments.
A lightweight, dependency-free JavaScript testing utility. This project is framework-agnostic and usable in both browser and Node.js environments.
npm install @moyal/js-test
import { Test } from "@moyal/js-test";
const { Test } = require("@moyal/js-test");
<!-- From jsDelivr CDN (minified version) -->
<script type="module">
import "https://cdn.jsdelivr.net/npm/@moyal/js-test@2.1.8/dist/moyal.test.umd.min.js";
</script>
<!-- From jsDelivr CDN (non minified version with documentation) -->
<script type="module">
import "https://cdn.jsdelivr.net/npm/@moyal/js-test@2.1.8/dist/moyal.test.umd.js";
</script>
Or using unpkg:
<script type="module">
import "https://unpkg.com/@moyal/js-test@2.1.8/dist/moyal.test.umd.min.js";
</script>
console
console
with indentation and ANSI coloringSee also quick-start folder for the source code of the examples.
import {MultiLevelAutoNumbering, TestGroup} from '@moyal/js-test';
new TestGroup("MLA Numbered Tests")
.areNotEqual("Validate inequality", 1, 2)
.areEqual("Test strings", "foo", "foo")
.groupStart("Nested test group")
.isFalse("This is lie", () => 1 == 2)
.areEqual("Test B2", "Hello World!", () => "Hello World!")
.groupClose();
.areEqual("Test booleans", true, true)
.areEqual("Test C", 123, 123)
.run(true, new MultiLevelAutoNumbering());
In this quick start example:
More examples can be found in examples and test/units.
Override console output with your custom logger:
import {Test, LoggerBase} from '@moyal/js-test';
class MyLogger extends LoggerBase {
/* implement logger methods */
log(message, color, ...args) { /* ... */}
info(message, color, ...args) { /* ... */ }
warn(message, color, ...args) { /* ... */ }
error(message, color, ...args) { /* ... */ }
group(label, color) { /* ... */ }
groupCollapsed(label, color) { /* ... */ }
groupEnd() { /* ... */ }
}
Test.logger = new MyLogger();
Note The logger methods are chainable.
Test
- Contains static method for testing.TestBase
- Derive your class from TestBase to create custom test.Assert
- Base class for assertions.IsDefined
- Asserts that the specified evaluates to defined value.IsUndefined
- Asserts that the specified evaluates to undefined value.IsFalse
- Asserts that the specified evaluates to false
.IsTrue
- Asserts that the specified evaluates to false
.IsNull
- Asserts that the specified evaluates to null
.IsNotNull
- Asserts that the specified evaluates to non null
value.AreEqual
- Asserts that the specified values evaluations are equal.AreNotEqual
- Asserts that the specified values evaluations are not equal.ThrowsBase
- Base class to test error throwing.Throws
- Asserts that the specified throws error.NoThrows
- Asserts that the specified does not throw error.SequencesAreEqual
- Asserts that the specified sequences are equal.TestGroup
- Groups and enables chaining of multiple tests.SequentialText
- Utility class to generate sequential text.AutoNumbering
- Utility class to generate automatic incremented number.MultiLevelAutoNumbering
- Utility class to generate automatic incremented number.LoggerBase
- Base class for logger.SimpleLogger
- Simple logger for unknown environments.BrowserLogger
- Console logger for browser.NodeLogger
- Console logger for NodeJS.The namespace MoyalTest
is also exported which wrapping all these types.
Access the library version directly:
import * as myLib from "@moyal/js-test";
myLib.Version // → e.g., "2.1.8"
Example files can be found under examples
folder and/or test/units
folder
(You can treat these test files as examples)
MIT License - free to use, modify, and distribute.
Ilan Moyal Website: https://www.moyal.es
GitHub: Ilan Moyal
LinkedIn: Ilan Moyal
FAQs
A lightweight, dependency-free JavaScript testing utility. This project is framework-agnostic and usable in both browser and Node.js environments.
We found that @moyal/js-test demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.