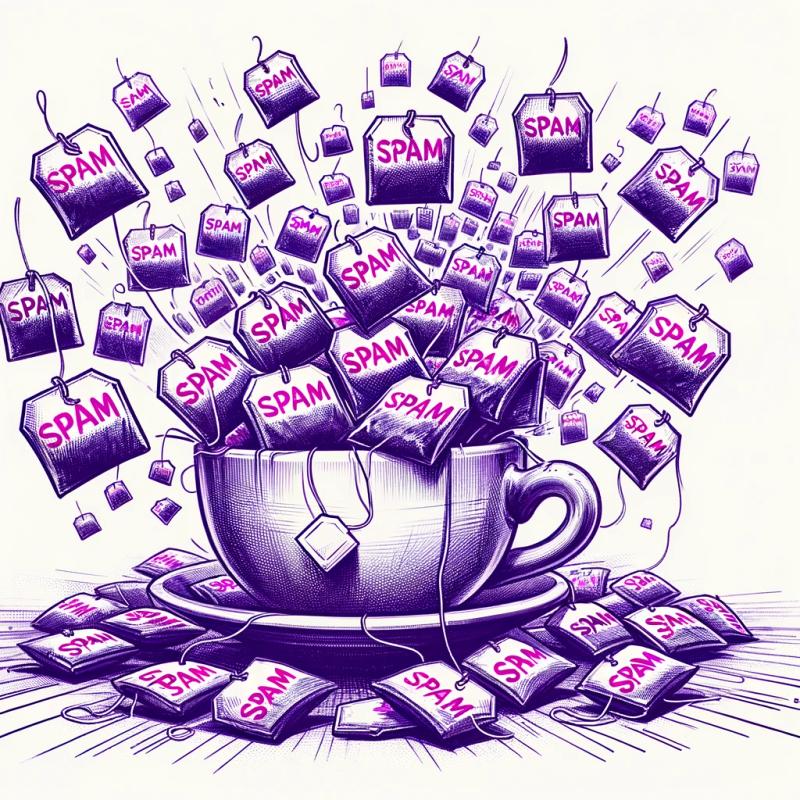
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@muehle/xml-decorators
Advanced tools
Readme
Decorators for xml serialization. Uses js2xmlparser under the hood.
import {XMLElement, XMLAttribute, XMLChild, xml} from 'xml-decorators';
const HOBBY_NS = 'h';
class Hobby {
@XMLAttribute({namespace: HOBBY_NS})
private name: string;
@XMLAttribute({namespace: HOBBY_NS})
private description: string;
}
const PERSON_ROOT = 'person';
const PERSON_NS = 'ps';
@XMLElement({root: PERSON_ROOT}) // optional
class Person {
@XMLAttribute({namespace: PERSON_NS})
private firstname: string;
private lastname: string;
@XMLAttribute({namespace: PERSON_NS})
get fullname(): string {
return this.firstname + ' ' + this.lastname;
}
@XMLAttribute({namespace: PERSON_NS})
private age: number;
@XMLChild({
namespace: PERSON_NS,
name: 'hobby'
})
private hobbies: Hobby[];
@XMLChild({
namespace: PERSON_NS,
stripPluralS: true
})
private friends: Person[];
@XMLChild({
name: 'pet',
implicitStructure: 'pets.$'
})
private pets: string[];
}
const hobbies = [
new Hobby('reading', 'loves to read books, magazines and web articles'),
new Hobby('listening to Music', 'loves to listen to rock music'),
new Hobby('travelling', 'loves to travel around the world'),
];
const pets = ['dog', 'cat'];
const bob = new Person('Bob', 'Mad', 29, hobbies, pets);
const bobXml = xml.serialize(bob);
Or if you want to override the root tag name or did not used the @XMLElement
annotation.
const bob2Xml = xml.serialize('great-person', bob);
<?xml version='1.0'?>
<great-person ps:firstname='Bob' ps:fullname='Bob Mad' ps:age='29'>
<ps:hobby h:name='reading' h:description='loves to read books, magazines and web articles'/>
<ps:hobby h:name='listening to Music' h:description='loves to listen to rock music'/>
<ps:hobby h:name='travelling' h:description='loves to travel around the world'/>
<pets>
<pet>dog</pet>
<pet>cat</pet>
</pets>
</great-person>
xml
.serializeAsync(bob)
.then(bobXml => console.log(bobXml))
;
If you want to retrieve the "js2xmlparser" schema instead:
xml.getSchema(bob);
xml.getSchemaAsync(bob).then(/* */);
FAQs
Decorators for xml serialization
The npm package @muehle/xml-decorators receives a total of 144 weekly downloads. As such, @muehle/xml-decorators popularity was classified as not popular.
We found that @muehle/xml-decorators demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.