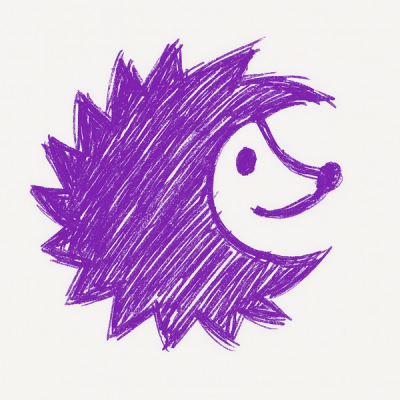
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
@nerd-coder/react-notifications
Advanced tools
Just another notification system for React.
The style is stolen heavily inspired by react-toast-notifications by Joss Mackison
npm i @nerd-coder/react-notifications
Wrap your app in the NotificationProvider
, which provides context for the useNotification
descendants.
import React, { useCallback } from 'react'
import { render } from 'react-dom'
import { useNotification, NotificationProvider } from '@nerd-coder/react-notifications'
const HelloComponent = ({ name }) => {
const { notify } = useNotification()
return <button onClick={() => notify(`Hello ${name}!`)}>Click me</button>
}
const App = () => (
<NotificationProvider>
<HelloComponent name='World' />
</NotificationProvider>
)
render(<App />, document.getElementById('root'))
Property | Type | Description |
---|---|---|
placement | 'top-left' | 'top-center' | 'top-right' | 'bottom-left' | 'bottom-center' | 'bottom-right' | Notification placement, default is bottom-right |
autoDismiss | boolean? | Auto dismiss the notification after timeout. Default is true |
autoDismissTimeout | number? | Work conjunction with autoDissmiss . Default is 5000 ms (5 seconds) |
animationTimeOut | number? | Timing for enter & exit animation. Default is 300 ms |
Tag | React.ComponentType? | Notification Component to render |
onAdd | (id: string) => void | Event handler, will be passed notification's id as first parameter |
onAdded | (id: string) => void | Event handler, will be passed notification's id as first parameter |
onRemove | (id: string) => void | Event handler, will be passed notification's id as first parameter |
onRemoved | (id: string) => void | Event handler, will be passed notification's id as first parameter |
The notify
and dimiss
methods on useNotification
have two arguments.
Node
.Options
object, which accept the same props as NotificationProvider
, and an additional prop appearance
having value being one of 'info' | 'warning' | 'success' | 'error'
FAQs
React Notifications
We found that @nerd-coder/react-notifications demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.