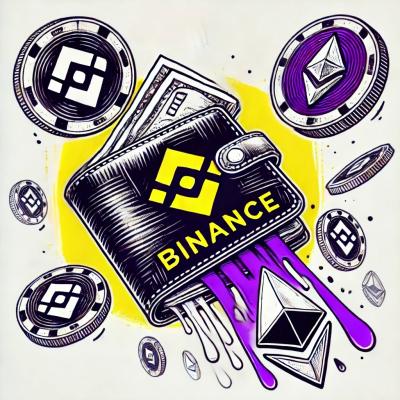
Research
Security News
Malicious npm Packages Target BSC and Ethereum to Drain Crypto Wallets
Socket uncovered four malicious npm packages that exfiltrate up to 85% of a victim’s Ethereum or BSC wallet using obfuscated JavaScript.
@nestjs/terminus
Advanced tools
Terminus integration provides readiness/liveness health checks for NestJS.
@nestjs/terminus is a health check module for NestJS applications. It provides a way to monitor the health of your application by exposing various health indicators and endpoints. This can be useful for ensuring that your application is running smoothly and for integrating with monitoring tools.
Basic Health Check
This code sets up a basic health check endpoint in a NestJS application. The `HealthCheckService` is used to perform the health check, and the `@HealthCheck` decorator is used to mark the endpoint.
```typescript
import { Module } from '@nestjs/common';
import { TerminusModule } from '@nestjs/terminus';
import { HealthController } from './health.controller';
@Module({
imports: [TerminusModule],
controllers: [HealthController],
})
export class AppModule {}
import { Controller, Get } from '@nestjs/common';
import { HealthCheck, HealthCheckService } from '@nestjs/terminus';
@Controller('health')
export class HealthController {
constructor(private health: HealthCheckService) {}
@Get()
@HealthCheck()
check() {
return this.health.check([]);
}
}
```
Database Health Check
This code adds a database health check to the health endpoint. The `TypeOrmHealthIndicator` is used to check the health of the database connection.
```typescript
import { TypeOrmHealthIndicator, HealthCheckService, HealthCheck } from '@nestjs/terminus';
import { Controller, Get } from '@nestjs/common';
@Controller('health')
export class HealthController {
constructor(
private health: HealthCheckService,
private db: TypeOrmHealthIndicator,
) {}
@Get()
@HealthCheck()
check() {
return this.health.check([
async () => this.db.pingCheck('database'),
]);
}
}
```
Custom Health Indicator
This code demonstrates how to create a custom health indicator. The `CustomHealthIndicator` class extends `HealthIndicator` and implements the `isHealthy` method. This custom indicator is then used in the health check endpoint.
```typescript
import { HealthIndicator, HealthIndicatorResult, HealthCheckError } from '@nestjs/terminus';
import { Injectable } from '@nestjs/common';
@Injectable()
export class CustomHealthIndicator extends HealthIndicator {
async isHealthy(key: string): Promise<HealthIndicatorResult> {
const isHealthy = true; // Custom logic to determine health
const result = this.getStatus(key, isHealthy);
if (isHealthy) {
return result;
}
throw new HealthCheckError('Custom health check failed', result);
}
}
import { Controller, Get } from '@nestjs/common';
import { HealthCheck, HealthCheckService } from '@nestjs/terminus';
@Controller('health')
export class HealthController {
constructor(
private health: HealthCheckService,
private customHealthIndicator: CustomHealthIndicator,
) {}
@Get()
@HealthCheck()
check() {
return this.health.check([
async () => this.customHealthIndicator.isHealthy('custom'),
]);
}
}
```
express-healthcheck is a middleware for Express.js applications that provides a simple health check endpoint. It is less feature-rich compared to @nestjs/terminus but can be a good choice for simpler use cases.
node-health-check is a lightweight health check library for Node.js applications. It provides basic health check functionality and can be easily integrated into any Node.js application. It is not as tightly integrated with a specific framework like @nestjs/terminus is with NestJS.
healthcheck-middleware is another middleware option for adding health checks to Node.js applications. It is framework-agnostic and provides a simple way to add health check endpoints. It lacks the advanced features and integrations of @nestjs/terminus.
A progressive Node.js framework for building efficient and scalable server-side applications, heavily inspired by Angular.
This module contains integrated healthchecks for Nest.
@nestjs/terminus
integrates with a lot of cool technologies, such as typeorm
, grpc
, mongodb
, and many more!
In case you have missed a dependency, @nestjs/terminus
will throw an error and prompt you to install the required dependency.
So you will only install what is actually required!
npm install --save @nestjs/terminus
TypeOrmModule
), in case you want to do Database Health Checks.// app.module.ts
@Module({
controllers: [HealthController],
imports:[
// Make sure TypeOrmModule is available in the module context
TypeOrmModule.forRoot({ ... }),
TerminusModule
],
})
export class HealthModule { }
HealthController
which executes your Health Check.// health.controller.ts
@Controller('health')
export class HealthController {
constructor(
private health: HealthCheckService,
private db: TypeOrmHealthIndicator,
) {}
@Get()
@HealthCheck()
readiness() {
return this.health.check([
async () => this.db.pingCheck('database', { timeout: 300 }),
]);
}
}
If everything is set up correctly, you can access the healthcheck on http://localhost:3000/health
.
{
"status": "ok",
"info": {
"database": {
"status": "up"
}
},
"details": {
"database": {
"status": "up"
}
}
}
For more information, see docs. You can find more samples in the samples/ folder of this repository.
In order to get started, first read through our Contributing guidelines.
Setup the development environment by following these instructions:
pnpm i
pnpm dev
In order to test the library against a sample, simply go to a sample and run
pnpm start:dev
cd sample/000-dogs-app
pnpm start:dev
[!NOTE] Once the library is rebuilt, the
pnpm start:dev
within a sample needs to be restarted in order to pick up the changes.
For unit testing run the following command:
pnpm test
For e2e testing, a Docker Compose stack is required. Make sure Docker is installed on your machine and run the following command.
docker compose up -d
pnpm test:e2e
Nest is an MIT-licensed open source project. It can grow thanks to the sponsors and support by the amazing backers. If you'd like to join them, please read more here.
Nest is MIT licensed.
11.0.0-beta.1 (2025-01-25)
FAQs
Terminus integration provides readiness/liveness health checks for NestJS.
The npm package @nestjs/terminus receives a total of 796,428 weekly downloads. As such, @nestjs/terminus popularity was classified as popular.
We found that @nestjs/terminus demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket uncovered four malicious npm packages that exfiltrate up to 85% of a victim’s Ethereum or BSC wallet using obfuscated JavaScript.
Security News
TC39 advances 9 JavaScript proposals, including Array.fromAsync, Error.isError, and Explicit Resource Management, which are now headed into the ECMAScript spec.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.