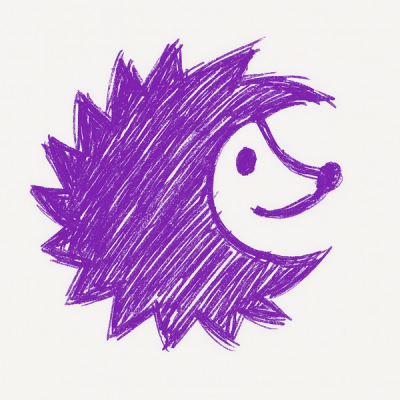
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
@notabene/nodejs
Advanced tools
Notabene NodeJS SDK for sending and receiving transactions through the Notabene Travel Rule gateway.
Documentation • Getting started • Installation • Configuration
npm install @notabene/nodejs
const { Notabene } = require('@notabene/nodejs');
const client = new Notabene({
clientId: '{CLIENT_ID}', // Add your own client ID
clientSecret: '{CLIENT_SECRET}', // Add your own client secret
});
The client ID and client secret required to authenticate against Notabene's APIs must be requested.
For sending transactions to Notabene's test environment, use your test Client ID and Client Secret and
set the baseURL
and audience
to https://api.notabene.dev
.
const { Notabene } = require('@notabene/nodejs');
const client = new Notabene({
baseURL: 'https://api.notabene.dev',
audience: 'https://api.notabene.dev',
clientId: '{CLIENT_ID}', // Add your own client ID
clientSecret: '{CLIENT_SECRET}', // Add your own client secret
});
client.trustFramework.get(...)
get properties of a VASPclient.trustFramework.list(...)
get a list of VASPsclient.trustFramework.update(...)
update properties of your VASPclient.transaction.create(...)
create a new outgoing transaction (see details below)client.transaction.get(...)
get transaction by id (see details below)client.transaction.update(...)
update transaction (see details below)client.transaction.list(did)
retrieve a list of transactionsclient.transaction.approve(id)
approve a transaction by IDclient.transaction.cancel(id)
cancel a transaction by IDclient.transaction.confirm(id)
confirm a transaction by IDclient.transaction.reject(id)
reject a transaction by IDclient.transaction.notReady(id)
mark transaction as Not Ready by IDclient.transaction.accept(id)
accept a transaction by IDclient.transaction.decline(id)
decline a transaction by IDclient.transaction.notify(...)
create an empty incoming transactionclient.transaction.redirect(fromDID, toDID)
redirect transactionclient.transaction.verifyMessage(signature, message, address)
Verify a signature (for beneficiary ownership proof)Examples for ivms
and payload
variables can be found in the Appendix.
const ivms = ...; // (see Appendix)
const payload = ...; // (see Appendix)
const txCreated = await client.transaction.create(payload);
E2E encryption method will encryt PII that such that only you and the beneficiary VASP
const ivms = ...; // (see Appendix)
const payload = ...; // (see Appendix)
const jsonDIDKey = ...; // create or import a jsonDIDKey (see below)
const txCreated = await client.transaction.create(
payload,
jsonDIDKey
);
The hybrid encryption method will also encrypt the PII data to Notabene, using a unique managed Escrow Key for your VASP. This allows us to run sanction screening on the PII data.
const txCreated = await client.transaction.create(
payload,
jsonDIDKey,
true // hybrid
);
For END_2_END
and HYBRID
encryption your VASP needs a dedicated DIDKey, which is a public-private keypair. You can create a new keypair using the @notabene/cli
and then publish it to the Notabene directory under the pii_didkey
field. This allows other VASPs retrieve your public key and encrypt PII data to you.
// get your encryption key
const ikey = ... // or create a new key using the CLI, or: await client.toolset.createKey();
// extract the did:key (public key)
const pii_didkey = JSON.parse(ikey).did;
// upload did:key to your VASP on the Notabene directory
const fields = [
{
fieldName: 'pii_didkey',
values: [
{
value: pii_didkey,
},
],
},
];
await client.trustFramework.update(vaspDID, fields);
Typically you will do this only once, and re-use the same keypair for a long time. If you believe your private key was compromised, you can rotate your keypair (ie. create a new one + publish it again). Data encrypted using a specific public key can only be decrypted with its private key, so don't throw away your old key(s) if you still have data of interest encrypted with those key(s).
To retrieve a transaction simply call:
const txInfo = await client.transaction.get(id);
If the transaction was encrypted with the HOSTED
(default) or HYBRID
strategy, the PII Service will be able to decrypt it for you, the ivms101
property will contain the decrypted data. However, for END_2_END
encrypted data you can pass your jsonDIDKey
argument to decrypt it locally:
const txInfo = await client.transaction.get(id, jsonDIDKey);
To update a transaction simply call the following with the fields you wish to update:
const updatedTx = await client.transaction.update({
id: txCreated.id,
beneficiaryVASPdid: '...',
});
Note, you need specify an encryption method just like in transaction.create
(and your jsonDIDKey
):
const updatedTxEnd2End = await client.transaction.update(
{ id: txCreated.id, beneficiaryVASPdid: '...' },
jsonDIDKey // for END_2_END | HYBRID
// false | true
);
// transaction.create payload:
const payload = {
transactionAsset: 'ETH',
transactionAmount: '1111111000000000000',
originatorVASPdid: 'did:ethr:0xb086499b7f028ab7d3c96c4c2b71d7f24c5a0772',
beneficiaryVASPdid: 'did:ethr:0xa80b54afa45dc22a4ebc0e1a9b638998a7899c33',
transactionBlockchainInfo: {
origin: '0x123',
destination: '0x321',
},
originator: ivms.originator,
beneficiary: ivms.beneficiary,
};
const ivms = {
originator: {
originatorPersons: [
{
naturalPerson: {
name: [
{
nameIdentifier: [
{
primaryIdentifier: 'Frodo',
secondaryIdentifier: 'Baggins',
nameIdentifierType: 'LEGL',
},
],
},
],
nationalIdentification: {
nationalIdentifier: 'AABBCCDDEEFF0011223344',
nationalIdentifierType: 'CCPT',
countryOfIssue: 'NZ',
},
dateAndPlaceOfBirth: {
dateOfBirth: '1900-01-01',
placeOfBirth: 'Planet Earth',
},
geographicAddress: [
{
addressLine: ['Cool Road /-.st'],
country: 'BE',
addressType: 'HOME',
},
],
},
},
],
accountNumber: ['01234567890'],
},
beneficiary: {
beneficiaryPersons: [
{
naturalPerson: {
name: [
{
nameIdentifier: [
{
primaryIdentifier: 'Bilbo',
secondaryIdentifier: 'Bolson',
nameIdentifierType: 'LEGL',
},
],
},
],
},
},
],
accountNumber: ['01234567890'],
},
};
BSD 3-Clause © Notabene Inc.
FAQs
Client for Notabene's API
The npm package @notabene/nodejs receives a total of 0 weekly downloads. As such, @notabene/nodejs popularity was classified as not popular.
We found that @notabene/nodejs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.