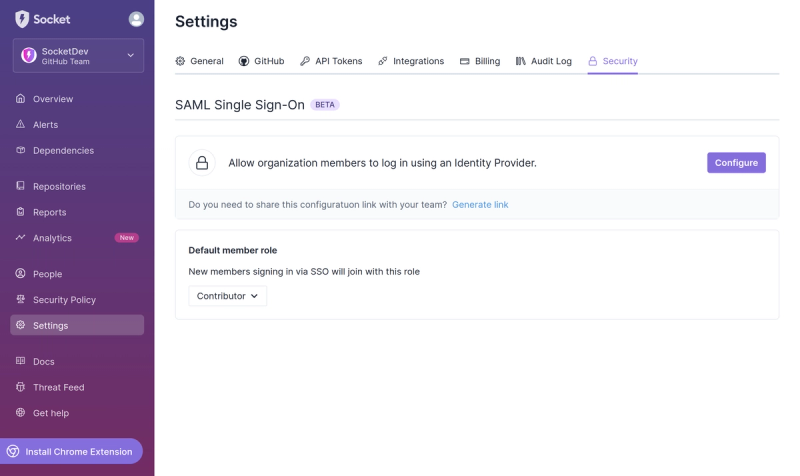
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
@notwoods/default-map
Advanced tools
Readme
A
Map
subclass that automatically adds missing values
import { DefaultMap } from "@notwoods/default-map";
const map = new DefaultMap(() => []);
map.get("key").push("array-item");
Includes TypeScript support and default arguments in just 281 bytes!
Inspired by Python's defaultdict
.
npm install @notwoods/default-map
Using arrays with the defaultFactory
, it is easy to group a sequence of key-value pairs into a map of arrays:
import { DefaultMap } from "@notwoods/default-map";
const pairs = [
{ key: "yellow", value: 1 },
{ key: "blue", value: 2 },
{ key: "yellow", value: 3 },
{ key: "blue", value: 4 },
{ key: "red", value: 1 },
];
const map = new DefaultMap(() => []);
for (const { key, value } of pairs) {
map.get(key).push(value);
}
Object.fromEntries(map);
// { blue: [2, 4], red: [1], yellow: [1, 3] }
When each key is encountered for the first time, it is not already in the mapping; so an entry is automatically created using the defaultFactory
function which returns an empty array. The array.push()
operation then attaches the value to the new array. When keys are encountered again, the look-up proceeds normally (returning the array for that key) and the array.push()
operation adds another value to the array. This technique is simpler than an equivalent technique using a Map
:
const map = new Map();
for (const { key, value } of pairs) {
if (map.has(key)) {
const array = map.get(key);
array.push(value);
} else {
map.set(key, [value]);
}
}
Setting the defaultFactory
to return an int makes the DefaultMap
useful for counting (like a bag or multiset in other languages):
import { DefaultMap } from "@notwoods/default-map";
const string = "mississippi";
const map = new DefaultMap(() => 0);
for (const char of string) {
map.get(char) += 1;
}
Object.fromEntries(map);
// { m: 1, i: 4, p: 2, s: 4 }
When a letter is first encountered, it is missing from the mapping, so the defaultFactory
function supplies a default count of zero. The increment operation then builds up the count for each letter.
Setting the defaultFactory
to return a Set
makes the DefaultMap
useful for building a dictionary of sets:
import { DefaultMap } from "@notwoods/default-map";
const pairs = [
{ key: "red", value: 1 },
{ key: "blue", value: 2 },
{ key: "red", value: 3 },
{ key: "blue", value: 4 },
{ key: "red", value: 1 },
{ key: "blue", value: 4 },
];
const map = new DefaultMap(() => new Set());
for (const { key, value } of pairs) {
map.get(key).add(value);
}
Object.fromEntries(map);
// { blue: Set([2, 4]), red: Set([1, 3]) }
Most functions are the same as a regular Map
.
Creates a new DefaultMap
. Both arguments are optional.
defaultFactory
: a function that returns the default value for an entry. It takes no arguments.entries
: an array or iterable of key-value pairs to initialize the map with.Returns the value
corresponding to key
, or adds a new default value to the map using the defaultFactory
function.
This attribute is initialized from the first argument to the constructor, if present, or to undefined
, if absent. .get(key)
will throw an error if .defaultFactory
is undefined
.
FAQs
A Map subclass that automatically adds missing values
The npm package @notwoods/default-map receives a total of 1 weekly downloads. As such, @notwoods/default-map popularity was classified as not popular.
We found that @notwoods/default-map demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.