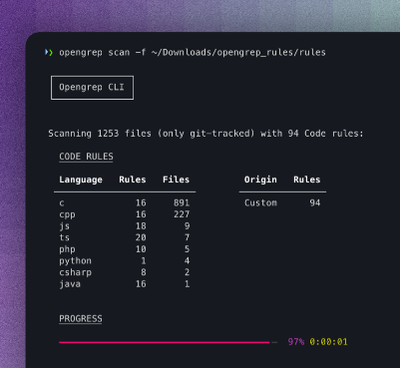
Security News
Opengrep Emerges as Open Source Alternative Amid Semgrep Licensing Controversy
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
@npmcli/fs
Advanced tools
The @npmcli/fs package is a file system utility library that provides a set of asynchronous file system methods. It is designed to offer more convenient and higher-level operations for interacting with the file system in Node.js applications. This package is particularly useful for tasks like reading, writing, and manipulating files and directories in a way that's compatible with the rest of the npm CLI ecosystem.
Reading files
This feature allows you to asynchronously read the contents of a file. The code sample demonstrates how to read a file and handle the promise returned by the readFile method.
const { readFile } = require('@npmcli/fs');
readFile('path/to/file.txt', 'utf8').then(contents => {
console.log(contents);
}).catch(error => {
console.error('Error reading file:', error);
});
Writing files
This feature enables you to write data to a file asynchronously. The code sample shows how to write a string to a file and handle the promise to catch any errors.
const { writeFile } = require('@npmcli/fs');
const content = 'Hello, world!';
writeFile('path/to/file.txt', content, 'utf8').then(() => {
console.log('File written successfully');
}).catch(error => {
console.error('Error writing file:', error);
});
Removing files
This feature provides a way to asynchronously remove a file. The code sample illustrates how to delete a file and handle the promise to catch errors.
const { unlink } = require('@npmcli/fs');
unlink('path/to/file.txt').then(() => {
console.log('File removed successfully');
}).catch(error => {
console.error('Error removing file:', error);
});
fs-extra is a popular npm package that extends the built-in Node.js fs module. It adds file system methods that aren't included in the native fs module, such as copying directories and files, removing directories, and ensuring directories exist. Compared to @npmcli/fs, fs-extra offers a broader set of file system operations and is widely used for its convenience methods.
rimraf is a Node.js package that provides a simple way to remove files and directories, mimicking the UNIX command `rm -rf`. It's specifically focused on deletion operations, making it more specialized compared to @npmcli/fs, which offers a variety of file system operations including reading, writing, and removing files.
polyfills, and extensions, of the core fs
module.
fs.cp
polyfill for node < 16.7.0fs.withTempDir
addedfs.readdirScoped
addedfs.moveFile
addedfs.withTempDir(root, fn, options) -> Promise
root
: the directory in which to create the temporary directoryfn
: a function that will be called with the path to the temporary directoryoptions
tmpPrefix
: a prefix to be used in the generated directory nameThe withTempDir
function creates a temporary directory, runs the provided
function (fn
), then removes the temporary directory and resolves or rejects
based on the result of fn
.
const fs = require('@npmcli/fs')
const os = require('os')
// this function will be called with the full path to the temporary directory
// it is called with `await` behind the scenes, so can be async if desired.
const myFunction = async (tempPath) => {
return 'done!'
}
const main = async () => {
const result = await fs.withTempDir(os.tmpdir(), myFunction)
// result === 'done!'
}
main()
fs.readdirScoped(root) -> Promise
root
: the directory to readLike fs.readdir
but handling @org/module
dirs as if they were
a single entry.
const { readdirScoped } = require('@npmcli/fs')
const entries = await readdirScoped('node_modules')
// entries will be something like: ['a', '@org/foo', '@org/bar']
fs.moveFile(source, dest, options) -> Promise
A fork of move-file with support for Common JS.
source
: File, or directory, you want to move.dest
: Where you want the file or directory moved.options
overwrite
(boolean
, default: true
): Overwrite existing destination file(s).The built-in
fs.rename()
is just a JavaScript wrapper for the C rename(2)
function, which doesn't
support moving files across partitions or devices. This module is what you
would have expected fs.rename()
to be.
const { moveFile } = require('@npmcli/fs');
(async () => {
await moveFile('source/unicorn.png', 'destination/unicorn.png');
console.log('The file has been moved');
})();
4.0.0 (2024-09-11)
@npmcli/fs
now supports node ^18.17.0 || >=20.5.0
1c67142
#123 enable auto publish (@hashtagchris)83f4580
#121 run template-oss-apply (@hashtagchris)65bff4e
#119 bump @npmcli/eslint-config from 4.0.5 to 5.0.0 (@dependabot[bot])6dd91fc
#120 postinstall for dependabot template-oss PR (@hashtagchris)72176f8
#120 bump @npmcli/template-oss from 4.23.1 to 4.23.3 (@dependabot[bot])FAQs
filesystem utilities for the npm cli
The npm package @npmcli/fs receives a total of 14,938,493 weekly downloads. As such, @npmcli/fs popularity was classified as popular.
We found that @npmcli/fs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.