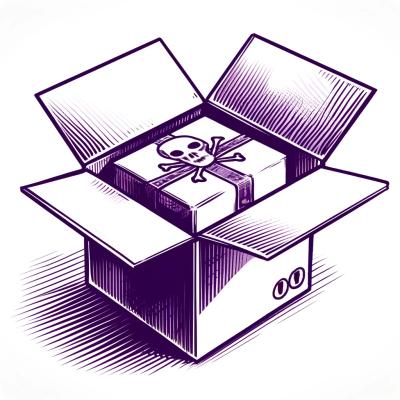
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@octokit/auth-oauth-device
Advanced tools
GitHub OAuth Device authentication strategy for JavaScript
@octokit/auth-oauth-device is an npm package that provides OAuth device flow authentication for GitHub. It allows users to authenticate with GitHub using a device code, which is particularly useful for applications running on devices with limited input capabilities.
Device Code Authentication
This feature allows you to authenticate a user via the OAuth device flow. The code sample demonstrates how to create an OAuth device authentication instance and use it to authenticate a user.
const { createOAuthDeviceAuth } = require('@octokit/auth-oauth-device');
const auth = createOAuthDeviceAuth({
clientType: 'oauth-app',
clientId: 'your-client-id',
clientSecret: 'your-client-secret'
});
(async () => {
const { authentication } = await auth({ type: 'oauth' });
console.log(authentication);
})();
Generate Device Code
This feature generates a device code and provides the user with a URL and code to authenticate. The code sample shows how to generate the device code and display the verification URL and user code.
const { createOAuthDeviceAuth } = require('@octokit/auth-oauth-device');
const auth = createOAuthDeviceAuth({
clientType: 'oauth-app',
clientId: 'your-client-id',
clientSecret: 'your-client-secret'
});
(async () => {
const { device_code, user_code, verification_uri } = await auth({ type: 'oauth', onVerification: ({ device_code, user_code, verification_uri }) => {
console.log(`Open ${verification_uri} and enter code: ${user_code}`);
}});
})();
passport-oauth2 is a strategy for the Passport authentication middleware that supports OAuth 2.0. It provides a comprehensive set of features for OAuth 2.0 authentication but does not specifically focus on device flow like @octokit/auth-oauth-device.
simple-oauth2 is a library that provides a simple and consistent way to handle OAuth 2.0 authentication. It supports various OAuth 2.0 flows, including the device flow, but is more general-purpose compared to the GitHub-specific @octokit/auth-oauth-device.
client-oauth2 is a library for creating OAuth 2.0 clients in JavaScript. It supports multiple OAuth 2.0 flows, including the device flow, and is designed to be flexible and easy to use. However, it is not specifically tailored for GitHub authentication.
GitHub OAuth Device authentication strategy for JavaScript
@octokit/auth-oauth-device
is implementing one of GitHub’s OAuth Device Flow.
createOAuthDeviceAuth(options)
auth(options)
auth.hook(request, route, parameters)
or auth.hook(request, options)
Browsers |
Load
|
---|---|
Node |
Install with
|
[!IMPORTANT] As we use conditional exports, you will need to adapt your
tsconfig.json
by setting"moduleResolution": "node16", "module": "node16"
.See the TypeScript docs on package.json "exports".
See this helpful guide on transitioning to ESM from @sindresorhus
const auth = createOAuthDeviceAuth({
clientType: "oauth-app",
clientId: "1234567890abcdef1234",
scopes: ["public_repo"],
onVerification(verification) {
// verification example
// {
// device_code: "3584d83530557fdd1f46af8289938c8ef79f9dc5",
// user_code: "WDJB-MJHT",
// verification_uri: "https://github.com/login/device",
// expires_in: 900,
// interval: 5,
// };
console.log("Open %s", verification.verification_uri);
console.log("Enter code: %s", verification.user_code);
},
});
const tokenAuthentication = await auth({
type: "oauth",
});
// resolves with
// {
// type: "token",
// tokenType: "oauth",
// clientType: "oauth-app",
// clientId: "1234567890abcdef1234",
// token: "...", /* the created oauth token */
// scopes: [] /* depend on request scopes by OAuth app */
// }
GitHub Apps do not support scopes
. Client IDs of GitHub Apps have a lv1.
prefix. If the GitHub App has expiring user tokens enabled, the resulting authentication
object has extra properties related to expiration and refreshing the token.
const auth = createOAuthDeviceAuth({
clientType: "github-app",
clientId: "lv1.1234567890abcdef",
onVerification(verification) {
// verification example
// {
// device_code: "3584d83530557fdd1f46af8289938c8ef79f9dc5",
// user_code: "WDJB-MJHT",
// verification_uri: "https://github.com/login/device",
// expires_in: 900,
// interval: 5,
// };
console.log("Open %s", verification.verification_uri);
console.log("Enter code: %s", verification.user_code);
},
});
const tokenAuthentication = await auth({
type: "oauth",
});
// resolves with
// {
// type: "token",
// tokenType: "oauth",
// clientType: "github-app",
// clientId: "lv1.1234567890abcdef",
// token: "...", /* the created oauth token */
// }
// or if expiring user tokens are enabled
// {
// type: "token",
// tokenType: "oauth",
// clientType: "github-app",
// clientId: "lv1.1234567890abcdef",
// token: "...", /* the created oauth token */
// refreshToken: "...",
// expiresAt: "2022-01-01T08:00:0.000Z",
// refreshTokenExpiresAt: "2021-07-01T00:00:0.000Z",
// }
createOAuthDeviceAuth(options)
The createOAuthDeviceAuth
method accepts a single options
parameter
name | type | description |
---|---|---|
clientId
|
string
|
Required. Find your OAuth app’s Client ID in your account’s developer settings.
|
onVerification
|
function
|
Required. A function that is called once the device and user codes were retrieved
The
|
clientType
|
string
|
Must be either |
request
|
function
|
You can pass in your own @octokit/request instance. For usage with enterprise, set baseUrl to the API root endpoint. Example:
|
scopes
|
array of strings
|
Only relevant if Array of scope names enabled for the token. Defaults to |
auth(options)
The async auth()
method returned by createOAuthDeviceAuth(options)
accepts the following options
name | type | description |
---|---|---|
type
|
string
|
Required. Must be set to "oauth"
|
scopes
|
array of strings
|
Only relevant if the Array of scope names enabled for the token. Defaults to what was set in the strategy options. See available scopes |
refresh
|
boolean
|
Defaults to |
The async auth(options)
method resolves to one of three possible objects
The differences are
scopes
is only present for OAuth AppsrefreshToken
, expiresAt
, refreshTokenExpiresAt
are only present for GitHub Apps, and only if token expiration is enabledname | type | description |
---|---|---|
type
|
string
|
"token"
|
tokenType
|
string
|
"oauth"
|
clientType
|
string
|
"github-app"
|
clientId
|
string
|
The app's Client ID
|
token
|
string
| The personal access token |
scopes
|
array of strings
| array of scope names enabled for the token |
name | type | description |
---|---|---|
type
|
string
|
"token"
|
tokenType
|
string
|
"oauth"
|
clientType
|
string
|
"github-app"
|
clientId
|
string
|
The app's Client ID
|
token
|
string
| The personal access token |
name | type | description |
---|---|---|
type
|
string
|
"token"
|
tokenType
|
string
|
"oauth"
|
clientType
|
string
|
"github-app"
|
clientId
|
string
|
The app's Client ID
|
token
|
string
| The user access token |
refreshToken
|
string
| The refresh token |
expiresAt
|
string
|
Date timestamp in ISO 8601 standard. Example: 2022-01-01T08:00:0.000Z
|
refreshTokenExpiresAt
|
string
|
Date timestamp in ISO 8601 standard. Example: 2021-07-01T00:00:0.000Z
|
auth.hook(request, route, parameters)
or auth.hook(request, options)
auth.hook()
hooks directly into the request life cycle. It amends the request to authenticate correctly based on the request URL.
The request
option is an instance of @octokit/request
. The route
/options
parameters are the same as for the request()
method.
auth.hook()
can be called directly to send an authenticated request
const { data: user } = await auth.hook(request, "GET /user");
Or it can be passed as option to request()
.
const requestWithAuth = request.defaults({
request: {
hook: auth.hook,
},
});
const { data: user } = await requestWithAuth("GET /user");
import {
OAuthAppStrategyOptions,
OAuthAppAuthOptions,
OAuthAppAuthentication,
GitHubAppStrategyOptions,
GitHubAppAuthOptions,
GitHubAppAuthentication,
GitHubAppAuthenticationWithExpiration,
} from "@octokit/auth-oauth-device";
GitHub's OAuth Device flow is different from the web flow in two ways
The flow has 3 parts (see GitHub documentation)
@octokit/auth-oauth-device
requests a device and user code@octokit/auth-oauth-device
is sending requests in the background to retrieve the OAuth access token. Once the user completed step 2, the request will succeed and the token will be returnedSee CONTRIBUTING.md
FAQs
GitHub OAuth Device authentication strategy for JavaScript
We found that @octokit/auth-oauth-device demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.