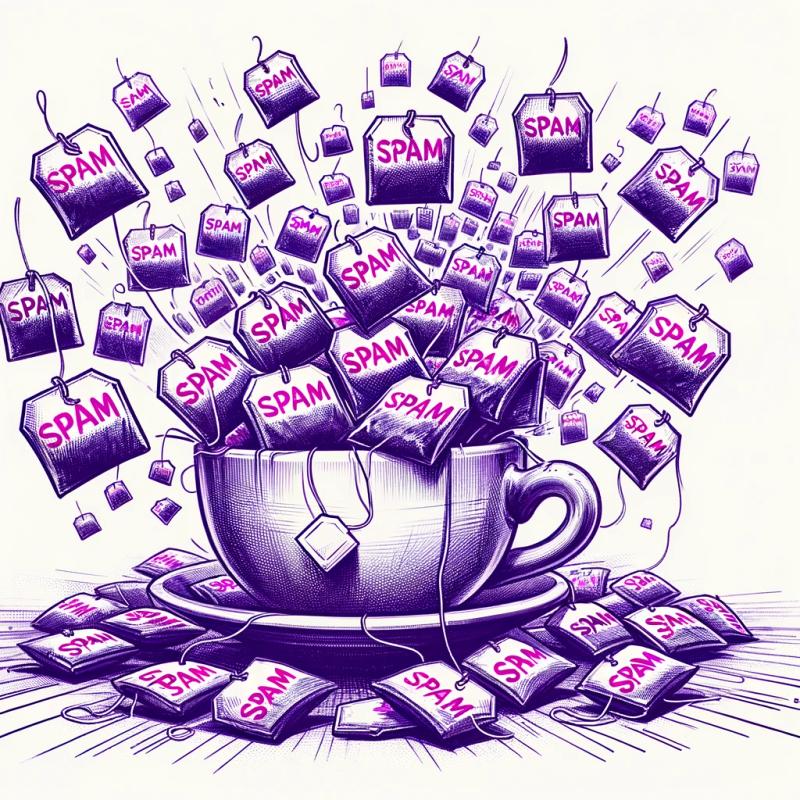
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
@oothkoo/seo-js
Advanced tools
Readme
The most simple, framework agnostic and easy to use JavaScript SEO meta tag management library.
npm install @oothkoo/seo-js
<script src="dist/seo-X.X.X.min.js" type="text/javascript"></script>
name | type | default | description |
---|---|---|---|
debug | Boolean | false | Enable debug mode |
headSelector | String | 'head' | Page CSS selector |
ignoreEmptyTagValue | Boolean | true | Ignore all meta registered with no values provided |
import Seo from '@oothkoo/seo-js'
var seo = new Seo({
debug: true,
headSelector: 'head',
ignoreEmptyTagValue: true
})
Register a meta configuration. You must register all metas before to use the library.
config
- Object - Configuration to use. seo.use({
name: 'name', // meta configuration ame
selector: 'name', // dom selector (.class, #id etc..)
template: '<template>{value}</template>' // html template
})
Generate a tag meta configuration. This configuration produces tags from this html template <{name}>{value}</{name}>
.
name
- String | Array - Tag name(s) // Register 'title' tag and produces <title>{value}</title>
seo.use(seo.tag('title'))
seo.use(seo.tag([
'author',
'section'
]))
Generate a meta[name=""] meta configuration. This configuration produces tags from this html template <meta name="{name}" content="{value}" />
.
name
- String | Array - Tag name(s) // Register 'title' tag and produces <meta name="title" content="{value}" />
seo.use(seo.metaName('title'))
seo.use(seo.metaName([
'keywords',
'description'
]))
Generate a meta[property=""] meta configuration. This configuration produces tags from this html template <meta property="{name}" content="{value}" />
.
name
- String | Array - Tag name(s) // Register 'og:title' tag and produces <meta name="og:title" content="{value}" />
seo.use(seo.metaProperty('og:title'))
seo.use(seo.metaProperty([
'og:site_name',
'og:description'
]))
Generate a link[rel=""] meta configuration. This configuration produces tags from this html template <link rel="{name}" href="{value}" />
.
name
- String | Array - Tag name(s) // Register 'favicon' tag and produces <link rel="favicon" href="{value}" />
seo.use(seo.link('favicon'))
seo.use(seo.link([
'icon',
'robots'
]))
Delete all registered SEO meta tags.
seo.clearMetas()
Delete all terms used for templating.
seo.clearTerms()
Setting a couple of terms to be used for templating.
terms
- Object - Tokens name/value list ({name: value}) // Setting terms for templating
seo.updateTerms({
'title': "Seo.js - A beautiful example",
'description': "Seo example page description",
'website': "https://www.oothkoo.com"
})
Delete all registered meta tags in current html page.
seo.clear()
Update all metas specified in current html page. Theses metas must be registered by Seo.use(config)
before calling this function.
metas
- Object - Meta name/value list ({name: value})only
- Boolean - Meta scope (default: false
)
true
: Update only metas specified in metas
argumentfalse
: Update all metas registered and overwrite if needed // Setting terms for templating
seo.update({
'title': "{title}", // Using terms 'title' for value
'description': "{description}", // Using terms 'description' for value
'website': "https://www.oothkoo.com"
})
// When page content is loaded
window.addEventListener('DOMContentLoaded', function () {
// Initialize Seo library
var seo = new Seo({
debug: true
})
// Register basic page metas
seo.use(seo.tag('title'))
seo.use(seo.metaName([
'description',
'keywords'
]))
seo.use(seo.metaLink('canonical'))
// Register Open Graph page metas
seo.use(seo.metaProperty([
'og:type',
'og:site_name',
'og:title',
'og:url',
'og:description',
'og:image',
'og:image:width',
'og:image:height'
]))
// Register Twitter page metas
seo.use(seo.metaName([
'twitter:card',
'twitter:site',
'twitter:creator',
'twitter:domain',
'twitter:url',
'twitter:image',
'twitter:title',
'twitter:description',
'twitter:label1',
'twitter:data1',
'twitter:label2',
'twitter:data2'
]))
// Register Slack page metas
seo.use(seo.metaName([
'slack-app-id'
]))
// Setting terms for templating
seo.updateTerms({
'name': "SlugBay",
'title': "Seo - A beautiful example",
'description': "SlugBay helps you centralise and better manage all your resources, and discover new interesting material more quickly",
'domain': "slugbay.com",
'website': "https://www.slugbay.com",
'image': "https://www.slugbay.com/pictures/opengraph.png",
'username': "@slugbay"
})
// Update all current registered metas
seo.update({
'title': "{title}",
'description': "{description}",
'keywords': "example keyword seo js",
'canonical': "{website}",
'og:type': "website",
'og:title': "{title}",
'og:site_name': "{name}",
'og:url': "{website}",
'og:description': "{description}",
'og:image': "{image}",
'og:image:width': "640",
'og:image:height': "280",
'twitter:card': "summary_large_image",
'twitter:site': "{username}",
'twitter:creator': "{username}",
'twitter:domain': "slugbay.com",
'twitter:url': "{website}",
'twitter:image': "{image}",
'twitter:title': "{title}",
'twitter:description': "{description}",
'slack-app-id': "A2UTWA5PT"
})
})
:heart: Donations are always welcome :heart:.
Coins | Symbols | Addresses |
---|---|---|
BTC | 3B52fbzNFQTaKZxWf5GrCUsASD2UP8na4A | |
ETH | 0x1C389f1f85Cdb3C2996b83fAc87E496A80698B7C |
FAQs
A simple vanilla JavaScript SEO library for SPAs (Single Page Applications).
The npm package @oothkoo/seo-js receives a total of 9 weekly downloads. As such, @oothkoo/seo-js popularity was classified as not popular.
We found that @oothkoo/seo-js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.