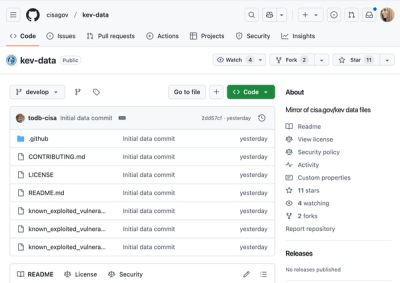
Security News
CISA Brings KEV Data to GitHub
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.
@opentelemetry/sdk-trace-base
Advanced tools
The @opentelemetry/sdk-trace-base package is part of the OpenTelemetry JavaScript SDK, which provides the core functionality for tracing. It allows developers to collect and export trace data to various backends for analysis and visualization. The package includes base classes and interfaces for implementing custom trace exporters, span processors, and configuration.
Basic Tracer Provider Setup
This code sets up a basic tracer provider with a simple span processor that exports spans to the console. It's the starting point for setting up OpenTelemetry tracing in an application.
const { BasicTracerProvider, SimpleSpanProcessor } = require('@opentelemetry/sdk-trace-base');
const { ConsoleSpanExporter } = require('@opentelemetry/tracing');
const provider = new BasicTracerProvider();
const consoleExporter = new ConsoleSpanExporter();
provider.addSpanProcessor(new SimpleSpanProcessor(consoleExporter));
provider.register();
Custom Span Processor
This code demonstrates how to create a custom span processor by extending the SpanProcessor class from the @opentelemetry/sdk-trace-base package. Developers can add custom logic for handling spans on start, on end, and during shutdown.
const { SpanProcessor } = require('@opentelemetry/sdk-trace-base');
class MySpanProcessor extends SpanProcessor {
onStart(span) {
// Custom logic for when a span starts.
}
onEnd(span) {
// Custom logic for when a span ends.
}
shutdown() {
// Custom logic for shutdown.
}
}
Configuring a Tracer Provider
This code snippet shows how to configure a tracer provider with a specific span processor and exporter. It's a common pattern for initializing tracing in an application with OpenTelemetry.
const { BasicTracerProvider, ConsoleSpanExporter, SimpleSpanProcessor } = require('@opentelemetry/sdk-trace-base');
const provider = new BasicTracerProvider();
const exporter = new ConsoleSpanExporter();
const processor = new SimpleSpanProcessor(exporter);
provider.addSpanProcessor(processor);
provider.register();
The jaeger-client package is a Node.js client for Jaeger, a distributed tracing system. It provides similar functionality to @opentelemetry/sdk-trace-base, allowing developers to send trace data to a Jaeger backend. However, it is specific to Jaeger, whereas OpenTelemetry provides a more vendor-neutral approach.
This package is an OpenTracing-compatible tracer implementation for Zipkin in JavaScript. Like @opentelemetry/sdk-trace-base, it enables tracing and exporting trace data but is tailored for integration with Zipkin, a different distributed tracing system.
Lightstep's tracer library for JavaScript is designed to monitor and trace application performance. It is similar to @opentelemetry/sdk-trace-base in that it collects trace data, but it is specifically built to work with Lightstep's performance monitoring platform.
The tracing
module contains the foundation for all tracing SDKs of opentelemetry-js.
Used standalone, this module provides methods for manual instrumentation of code, offering full control over span creation for client-side JavaScript (browser) and Node.js.
It does not provide automated instrumentation of known libraries, context propagation for asynchronous invocations or distributed-context out-of-the-box.
For automated instrumentation for Node.js, please see @opentelemetry/sdk-trace-node.
npm install --save @opentelemetry/api
npm install --save @opentelemetry/sdk-trace-base
const opentelemetry = require('@opentelemetry/api');
const { BasicTracerProvider } = require('@opentelemetry/sdk-trace-base');
// To start a trace, you first need to initialize the Tracer provider.
// NOTE: The default OpenTelemetry tracer provider does not record any tracing information.
// Registering a working tracer provider allows the API methods to record traces.
new BasicTracerProvider().register();
// To create a span in a trace, we used the global singleton tracer to start a new span.
const span = opentelemetry.trace.getTracer('default').startSpan('foo');
// Set a span attribute
span.setAttribute('key', 'value');
// We must end the spans so they become available for exporting.
span.end();
Tracing configuration is a merge of user supplied configuration with both the default
configuration as specified in config.ts and an
environmentally configurable sampling (via OTEL_TRACES_SAMPLER
and OTEL_TRACES_SAMPLER_ARG
).
Sampler is used to make decisions on Span
sampling.
Samples every trace regardless of upstream sampling decisions.
This is used as a default Sampler
const {
AlwaysOnSampler,
BasicTracerProvider,
} = require("@opentelemetry/sdk-trace-base");
const tracerProvider = new BasicTracerProvider({
sampler: new AlwaysOnSampler()
});
Doesn't sample any trace, regardless of upstream sampling decisions.
const {
AlwaysOffSampler,
BasicTracerProvider,
} = require("@opentelemetry/sdk-trace-base");
const tracerProvider = new BasicTracerProvider({
sampler: new AlwaysOffSampler()
});
Samples some percentage of traces, calculated deterministically using the trace ID. Any trace that would be sampled at a given percentage will also be sampled at any higher percentage.
The TraceIDRatioSampler
may be used with the ParentBasedSampler
to respect the sampled flag of an incoming trace.
const {
BasicTracerProvider,
TraceIdRatioBasedSampler,
} = require("@opentelemetry/sdk-trace-base");
const tracerProvider = new BasicTracerProvider({
// See details of ParentBasedSampler below
sampler: new ParentBasedSampler({
// Trace ID Ratio Sampler accepts a positional argument
// which represents the percentage of traces which should
// be sampled.
root: new TraceIdRatioBasedSampler(0.5)
});
});
ParentBased
helps distinguished between the
following cases:
sampled
flag true
sampled
flag false
sampled
flag true
sampled
flag false
Required parameters:
root(Sampler)
- Sampler called for spans with no parent (root spans)Optional parameters:
remoteParentSampled(Sampler)
(default: AlwaysOn
)remoteParentNotSampled(Sampler)
(default: AlwaysOff
)localParentSampled(Sampler)
(default: AlwaysOn
)localParentNotSampled(Sampler)
(default: AlwaysOff
)Parent | parent.isRemote() | parent.isSampled() | Invoke sampler |
---|---|---|---|
absent | n/a | n/a | root() |
present | true | true | remoteParentSampled() |
present | true | false | remoteParentNotSampled() |
present | false | true | localParentSampled() |
present | false | false | localParentNotSampled() |
const {
AlwaysOffSampler,
BasicTracerProvider,
ParentBasedSampler,
TraceIdRatioBasedSampler,
} = require("@opentelemetry/sdk-trace-base");
const tracerProvider = new BasicTracerProvider({
sampler: new ParentBasedSampler({
// By default, the ParentBasedSampler will respect the parent span's sampling
// decision. This is configurable by providing a different sampler to use
// based on the situation. See configuration details above.
//
// This will delegate the sampling decision of all root traces (no parent)
// to the TraceIdRatioBasedSampler.
// See details of TraceIdRatioBasedSampler above.
root: new TraceIdRatioBasedSampler(0.5)
})
});
See examples/basic-tracer-node for an end-to-end example, including exporting created spans.
Apache 2.0 - See LICENSE for more information.
FAQs
OpenTelemetry Tracing
The npm package @opentelemetry/sdk-trace-base receives a total of 8,039,050 weekly downloads. As such, @opentelemetry/sdk-trace-base popularity was classified as popular.
We found that @opentelemetry/sdk-trace-base demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.