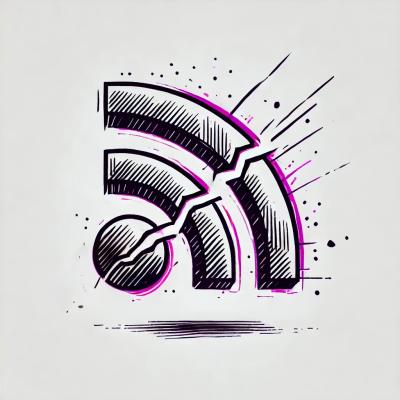
Security News
CISA Kills Off RSS Feeds for KEVs and Cyber Alerts
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
@panelist/react-hls-player
Advanced tools
A simple and easy to use react component for playing an hls live stream
react-hls-player
is a simple HLS live stream player.
It uses hls.js to play your hls live stream if your browser supports html 5 video
and MediaSource Extension
.
import React from 'react';
import ReactDOM from 'react-dom';
import ReactHlsPlayer from 'react-hls-player';
ReactDOM.render(
<ReactHlsPlayer
src="https://bitdash-a.akamaihd.net/content/sintel/hls/playlist.m3u8"
autoPlay={false}
controls={true}
width="100%"
height="auto"
/>,
document.getElementById('app')
);
All available config properties can be found on the Fine Tuning section of the Hls.js API.md
import React from 'react';
import ReactDOM from 'react-dom';
import ReactHlsPlayer from 'react-hls-player';
ReactDOM.render(
<ReactHlsPlayer
src="https://bitdash-a.akamaihd.net/content/sintel/hls/playlist.m3u8"
hlsConfig={{
maxLoadingDelay: 4,
minAutoBitrate: 0,
lowLatencyMode: true,
}}
/>,
document.getElementById('app')
);
The playerRef
returns a ref to the underlying video component, and as such will give you access to all video component properties and methods.
import React from 'react';
import ReactHlsPlayer from 'react-hls-player';
function MyCustomComponent() {
const playerRef = React.useRef();
function playVideo() {
playerRef.current.play();
}
function pauseVideo() {
playerRef.current.pause();
}
function toggleControls() {
playerRef.current.controls = !playerRef.current.controls;
}
return (
<ReactHlsPlayer
playerRef={playerRef}
src="https://bitdash-a.akamaihd.net/content/sintel/hls/playlist.m3u8"
/>
);
}
ReactDOM.render(<MyCustomComponent />, document.getElementById('app'));
You can also listen to events of the video
import React from 'react';
import ReactHlsPlayer from 'react-hls-player';
function MyCustomComponent() {
const playerRef = React.useRef();
React.useEffect(() => {
function fireOnVideoStart() {
// Do some stuff when the video starts/resumes playing
}
playerRef.current.addEventListener('play', fireOnVideoStart);
return playerRef.current.removeEventListener('play', fireOnVideoStart);
}, []);
React.useEffect(() => {
function fireOnVideoEnd() {
// Do some stuff when the video ends
}
playerRef.current.addEventListener('ended', fireOnVideoEnd);
return playerRef.current.removeEventListener('ended', fireOnVideoEnd);
}, []);
return (
<ReactHlsPlayer
playerRef={playerRef}
src="https://bitdash-a.akamaihd.net/content/sintel/hls/playlist.m3u8"
/>
);
}
ReactDOM.render(<MyCustomComponent />, document.getElementById('app'));
All video properties are supported and passed down to the underlying video component
Prop | Description |
---|---|
src String , required | The hls url that you want to play |
autoPlay Boolean | Autoplay when component is ready. Defaults to false |
controls Boolean | Whether or not to show the playback controls. Defaults to false |
width Number | Video width. Defaults to 100% |
height Number | Video height. Defaults to auto |
hlsConfig Object | hls.js config, you can see all config here |
playerRef React Ref | Pass in your own ref to interact with the video player directly. This will override the default ref. |
By default, the HLS config will have enableWorker
set to false
. There have been issues with the HLS.js library that breaks some React apps, so I've disabled it to prevent people from running in to this issue. If you want to enable it and see if it works with your React app, you can simply pass in enableWorker: true
to the hlsConfig
prop object. See this issue for more information
Thanks goes to these wonderful people (emoji key):
Marco Chavez 💻 | Chris Short 💻 📆 |
This project follows the all-contributors specification. Contributions of any kind welcome!
FAQs
A simple and easy to use react component for playing an hls live stream
The npm package @panelist/react-hls-player receives a total of 37 weekly downloads. As such, @panelist/react-hls-player popularity was classified as not popular.
We found that @panelist/react-hls-player demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 9 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.