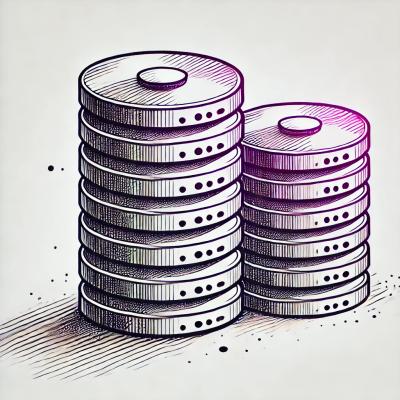
Security News
MCP Community Begins Work on Official MCP Metaregistry
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
@peacechen/react-image-file-resizer
Advanced tools
React module that rescales local images. You can change image's width, height, format, rotation and quality. It returns resized image's new base64 URI, Blob, or File. The URI can be used as the source of an component.
@peacechen/react-image-file-resizer
is a react module that resizes images in the browser.
<Image>
component.imageFileResizer
accepts an options object argument instead of multiple individual arguments.This fork has been published as @peacechen/react-image-file-resizer
pending merge into the parent project.
Install the package:
npm i @peacechen/react-image-file-resizer
or
yarn add @peacechen/react-image-file-resizer
import { imageFileResizer } from "@peacechen/react-image-file-resizer";
const newImage = await imageFileResizer({
compressFormat, // the compression format of the resized image.
file, // the file of the image to resize.
maxHeight, // the maxHeight of the resized image.
maxWidth, // the maxWidth of the resized image.
minHeight // the minHeight of the resized image.
minWidth, // the minWidth of the resized image.
outputType, // the output type of the resized image.
quality, // the quality of the resized image.
rotation, // the degree of clockwise rotation to apply to uploaded image.
});
import React, { useState } from "react";
import { imageFileResizer } from "@peacechen/react-image-file-resizer";
export function App() {
const [newImage, setNewImage] = useState();
async function fileChangedHandler(event) {
let fileInput = event.target.files[0];
if (fileInput) {
try {
const uri = await imageFileResizer({
compressFormat: "jpeg",
file: fileInput,
maxHeight: 300,
maxWidth: 300,
minHeight: 200,
minWidth: 200,
outputType: "base64"
quality: 100,
rotation: 0,
});
console.log(uri);
setNewImage(uri);
} catch (err) {
console.log(err);
}
}
}
render() {
return (
<div className="App">
<input type="file" onChange={fileChangedHandler} />
<img src={newImage} alt="" />
</div>
);
}
}
export default App;
Options object | Description | Type | Default | Required |
---|---|---|---|---|
compressFormat | Image format: jpeg, png or webp. | string | "jpeg" | No |
file | Image File | File | Yes | |
maxHeight | New image max height (ratio is preserved) | number | Yes | |
maxWidth | New image max width (ratio is preserved) | number | Yes | |
minHeight | New image min height (ratio is preserved unless minHeight === maxHeight) | number | No | |
minWidth | New image min width (ratio is preserved unless minWidth === maxWidth) | number | No | |
outputType | Output type: base64, blob or file. | string | "base64" | No |
quality | A number between 0 and 100. Used for the JPEG compression. (100 = no compression) | number | 100 | No |
rotation | Degree of clockwise rotation to apply to the image. Rotation is limited to 0, 90, 180, 270. (0 = no rotation) | number | 0 | No |
imageFileResizer
returns a promise that resolves to type string | File | Blob
depending on the outputType
option.
npm run build
npm publish
Thanks goes to these wonderful people (emoji key):
Ahmad Maleki 💻 🚧 | Martin Vyšňovský 💻 🚧 | Nadun Chamikara 💻 🚧 | Shubham Zanwar 📖 | Onur Önder 💻 🚧 | Yunus Emre 💻 🚧 | Juan 💻 🚧 |
Sreang Rathanak 💻 🚧 | Andres Rivera 💻 🚧 | mmmulani 💻 🚧 | Alex-1701 🚧 |
This project follows the all-contributors specification. Contributions of any kind welcome!
FAQs
React module that rescales local images. You can change image's width, height, format, rotation and quality. It returns resized image's new base64 URI, Blob, or File. The URI can be used as the source of an component.
The npm package @peacechen/react-image-file-resizer receives a total of 393 weekly downloads. As such, @peacechen/react-image-file-resizer popularity was classified as not popular.
We found that @peacechen/react-image-file-resizer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.
Research
Security News
Malicious npm packages posing as developer tools target macOS Cursor IDE users, stealing credentials and modifying files to gain persistent backdoor access.