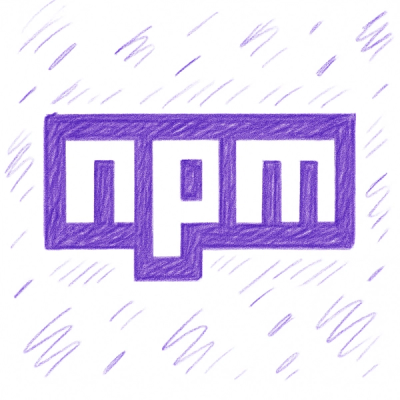
Security News
npm Adopts OIDC for Trusted Publishing in CI/CD Workflows
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
@putout/plugin-nodejs
Advanced tools
πPutout plugin adds ability to transform code to new API of Node.js
Node.js is an open-source, cross-platform, JavaScript runtime environment.
(c) Nodejs.org
πPutout plugin adds ability to transform to new Node.js API and apply best practices.
npm i putout @putout/plugin-nodejs -D
{
"rules": {
"nodejs/convert-commonjs-to-esm": "off",
"nodejs/convert-esm-to-commonjs": "off",
"nodejs/cjs-file": "off",
"nodejs/mjs-file": "off",
"nodejs/rename-file-cjs-to-js": "off",
"nodejs/rename-file-mjs-to-js": "off",
"nodejs/add-node-prefix": "on",
"nodejs/convert-buffer-to-buffer-alloc": "on",
"nodejs/convert-fs-promises": "on",
"nodejs/convert-promisify-to-fs-promises": "on",
"nodejs/convert-dirname-to-url": "on",
"nodejs/convert-exportst-to-module-exports": "on",
"nodejs/convert-url-to-dirname": "on",
"nodejs/convert-top-level-return": "on",
"nodejs/declare": "on",
"nodejs/declare-after-require": "on",
"nodejs/group-require-by-id": "on",
"nodejs/remove-process-exit": "on",
"nodejs/add-missing-strict-mode": "on",
"nodejs/remove-useless-strict-mode": "on",
"nodejs/remove-illegal-strict-mode": "on",
"nodejs/remove-useless-promisify": "on"
}
}
Deno
supports using Node.js built-in modules such asfs
,path
,process
, and many more vianode
: specifiers.(c) deno.land
Check out in πPutout Editor.
import fs from 'fs';
const path = require('path');
await import('path');
import fs from 'node:fs';
const path = require('node:path');
await import('node:path');
Linter | Rule | Fix |
---|---|---|
π Putout | apply-node-prefix | β |
β£ ESLint | prefer-node-protocol | β |
The
Buffer()
function andnew Buffer()
constructor are deprecated due to API usability issues that can lead to accidental security issues.(c) DEP0005
Check out in πPutout Editor.
const n = 100;
const buf = [];
new Buffer(123);
new Buffer(n);
new Buffer('hello');
new Buffer([]);
new Buffer(buf);
const n = 100;
const buf = [];
Buffer.alloc(123);
Buffer.alloc(n);
Buffer.from('hello');
Buffer.from([]);
Buffer.from(buf);
Convert fs.promises into form that will be simpler to use and convert to and from ESM.
const {readFile} = require('fs').promises;
const {readFile} = require('fs/promises');
const fs = require('fs');
const readFile = promisify(fs.readFile);
const {readFile} = require('fs/promises');
Only for ESM.
const {join} = require('path');
const path = require('path');
const file1 = join(__dirname, '../../package.json');
const file2 = path.join(__dirname, '../../package.json');
const file1 = new URL('../../package.json', import.meta.url);
const file2 = new URL('../../package.json', import.meta.url);
Only for CommonJS.
const {readFile} = require('fs/promises');
const file = new URL('../../package.json', import.meta.url);
const {readFile} = require('fs/promises');
const {join} = require('path');
const file = join(__dirname, '../../package.json');
In most cases process.exit()
is called from bin
directory, if not - disable this rule using match
.
-process.exit();
Since exports = 5
wan't make any export, just change value of variable.
Checkout in πPutout Editor.
exports.x = 5;
module.exports.x = 5;
return;
process.exit();
Add declarations to built-in node.js modules:
Based on @putout/operator-declare
.
await readFile('hello.txt', 'utf8');
import {readFile} from 'fs/promises';
await readFile('hello.txt', 'utf8');
When you want to skip some declaration use dismiss
:
{
"rules": {
"nodejs/declare": ["on", {
"dismiss": ["readFile"]
}]
}
}
Node.js follows the CommonJS module system, and the builtin
require
function is the easiest way to include modules that exist in separate files. The basic functionality ofrequire
is that it reads a JavaScript file, executes the file, and then proceeds to return theexports
object.(c) Nodejs.org
Check out in πPutout Editor.For ESM use esm/declare-imports-first
.
const name = 'hello.txt';
const {readFile} = require('fs/promises');
const {readFile} = require('fs/promises');
const name = 'hello.txt';
Convert CommonJS EcmaScript Modules.
EcmaScript module syntax is the standard way to import and export values between files in JavaScript. The
import
statement can be used to reference a value exposed by theexport
statement in another file.(c) parceljs
const {join} = require('path');
const args = require('minimist')({
string: ['a', 'b'],
});
import {join} from 'path';
import minimist from 'minimist';
const args = minimist({
string: ['a', 'b'],
});
module.exports = () => {};
export default () => {};
const {readFile} = require('fs/promises');
await readFile(__filename);
import {readFile} from 'fs/promises';
import {fileURLToPath} from 'url';
const __filename = fileURLToPath(import.meta.url);
await readFile(__filename);
Checkout in πPutout Editor. For ESM use esm/group-imports-by-sources
.
const ss = require('../../bb/ss');
const d = require('../hello');
const react = require('react');
const {lodash} = require('lodash');
const fs = require('node:fs');
const b = require('./ss');
const m = require(x);
const c = 5;
const fs = require('node:fs');
const react = require('react');
const {lodash} = require('lodash');
const ss = require('../../bb/ss');
const d = require('../hello');
const b = require('./ss');
const m = require(x);
const c = 5;
CommonJS is a module system supported in Node, it provides a
require
function, which can be used to access theexports
object exposed by another file.(c) parceljs
Convert EcmaScript Modules to CommonJS.
import hello from 'world';
const hello = require('world');
Run convert-esm-to-commonjs for all *.cjs
files with help of redlint.
Check out in πPutout Editor.
Run convert-commonjs-to-esm for all *.cjs
files with help of redlint.
Check out in πPutout Editor.
Rename *.cjs
files when type === "commonjs"
:
/
|-- package.json
`-- lib/
- `-- hello.cjs
+ `-- hello.js
Check out in πPutout Editor.
Rename *.mjs
files when type === "module"
:
/
|-- package.json
`-- lib/
- `-- hello.mjs
+ `-- hello.js
Check out in πPutout Editor.
Strict mode makes several changes to normal JavaScript semantics:
- Eliminates some JavaScript silent errors by changing them to throw errors.
- Fixes mistakes that make it difficult for JavaScript engines to perform optimizations: strict mode code can sometimes be made to run faster than identical code that's not strict mode.
- Prohibits some syntax likely to be defined in future versions of ECMAScript.
(c) MDN
Add strict mode to CommonJS:
const a = require('b');
'strict mode';
const a = require('b');
Remove 'use strict'
from ESM.
'strict mode';
import a from 'b';
import a from 'b';
SyntaxError: "use strict" not allowed in function with non-simple parameters
The JavaScript exception"use strict" not allowed in function
occurs when ause strict
directive is used at the top of a function with default parameters, rest parameters, or destructuring parameters.(c) MDN
Checkout in πPutout Editor.
function x1(...a) {
'use strict';
}
function x2(a, b = 3) {
'use strict';
}
function x3({a}) {
'use strict';
}
function x4([a]) {
'use strict';
}
function x5(...a) {
'use strict';
}
function x1(...a) {}
function x2(a, b = 3) {}
function x3({a}) {}
function x4([a]) {}
function x5(...a) {}
Takes a function following the common error-first callback style, i.e. taking an (err, value) => ... callback as the last argument, and returns a version that returns promises.
(c) nodejs.org
Remove useless promisify()
. Checkout in πPutout Editor.
export const readSize = promisify(async (dir, options, callback) => {});
export const readSize = async (dir, options, callback) => {};
MIT
FAQs
πPutout plugin adds ability to transform code to new API of Node.js
The npm package @putout/plugin-nodejs receives a total of 10,155 weekly downloads. As such, @putout/plugin-nodejs popularity was classified as popular.
We found that @putout/plugin-nodejs demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago.Β It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.