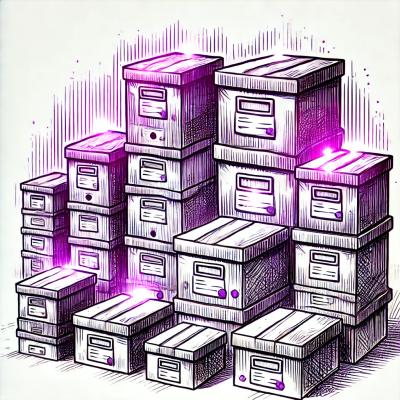
Security News
PyPI’s New Archival Feature Closes a Major Security Gap
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
@react-aria/tooltip
Advanced tools
@react-aria/tooltip is a React library that provides accessible tooltip components. It is part of the React Aria collection of hooks and components designed to help developers build accessible web applications. The package ensures that tooltips are compliant with ARIA (Accessible Rich Internet Applications) standards, making them usable for people with disabilities.
Basic Tooltip
This code demonstrates a basic tooltip implementation using @react-aria/tooltip. It includes a button that, when hovered over or focused, displays a tooltip with the text 'Tooltip content'. The tooltip is accessible and follows ARIA standards.
```jsx
import { useTooltip, useTooltipTrigger } from '@react-aria/tooltip';
import { useTooltipTriggerState } from '@react-stately/tooltip';
function TooltipExample() {
let state = useTooltipTriggerState();
let ref = React.useRef();
let { triggerProps, tooltipProps } = useTooltipTrigger({ state }, ref);
let { tooltipProps: ariaTooltipProps } = useTooltip({ state });
return (
<div>
<button {...triggerProps} ref={ref}>Hover or focus me</button>
{state.isOpen && (
<div {...ariaTooltipProps} {...tooltipProps}>
Tooltip content
</div>
)}
</div>
);
}
```
Custom Styling
This example shows how to apply custom styling to the tooltip. The tooltip content is styled with a black background, white text, padding, and rounded corners.
```jsx
import { useTooltip, useTooltipTrigger } from '@react-aria/tooltip';
import { useTooltipTriggerState } from '@react-stately/tooltip';
function CustomTooltipExample() {
let state = useTooltipTriggerState();
let ref = React.useRef();
let { triggerProps, tooltipProps } = useTooltipTrigger({ state }, ref);
let { tooltipProps: ariaTooltipProps } = useTooltip({ state });
return (
<div>
<button {...triggerProps} ref={ref}>Hover or focus me</button>
{state.isOpen && (
<div {...ariaTooltipProps} {...tooltipProps} style={{ backgroundColor: 'black', color: 'white', padding: '5px', borderRadius: '3px' }}>
Custom styled tooltip content
</div>
)}
</div>
);
}
```
Tooltip with Delay
This example demonstrates how to add a delay to the tooltip display. The tooltip will appear 500 milliseconds after the button is hovered over or focused.
```jsx
import { useTooltip, useTooltipTrigger } from '@react-aria/tooltip';
import { useTooltipTriggerState } from '@react-stately/tooltip';
function DelayedTooltipExample() {
let state = useTooltipTriggerState({ delay: 500 });
let ref = React.useRef();
let { triggerProps, tooltipProps } = useTooltipTrigger({ state }, ref);
let { tooltipProps: ariaTooltipProps } = useTooltip({ state });
return (
<div>
<button {...triggerProps} ref={ref}>Hover or focus me</button>
{state.isOpen && (
<div {...ariaTooltipProps} {...tooltipProps}>
Tooltip with delay
</div>
)}
</div>
);
}
```
react-tooltip is a popular library for creating tooltips in React applications. It offers a wide range of customization options and is easy to use. However, it may not be as focused on accessibility as @react-aria/tooltip.
tippy.js is a highly customizable tooltip and popover library that can be used with React. It provides extensive configuration options and supports various themes and animations. While it is very flexible, developers need to ensure accessibility compliance manually.
react-popper is a library for positioning tooltips and popovers in React applications. It is built on top of Popper.js and provides powerful positioning capabilities. Accessibility features are not as built-in as in @react-aria/tooltip, so additional work may be required to ensure compliance.
This package is part of react-spectrum. See the repo for more details.
FAQs
Spectrum UI components in React
The npm package @react-aria/tooltip receives a total of 722,056 weekly downloads. As such, @react-aria/tooltip popularity was classified as popular.
We found that @react-aria/tooltip demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now allows maintainers to archive projects, improving security and helping users make informed decisions about their dependencies.
Research
Security News
Malicious npm package postcss-optimizer delivers BeaverTail malware, targeting developer systems; similarities to past campaigns suggest a North Korean connection.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.