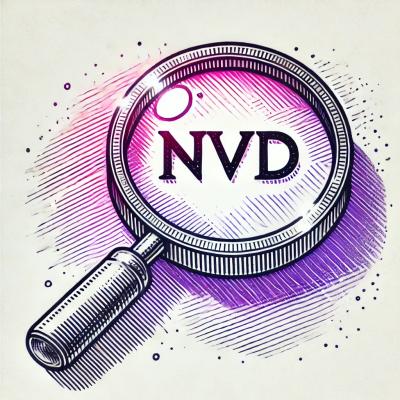
Security News
NIST Under Federal Audit for NVD Processing Backlog and Delays
As vulnerability data bottlenecks grow, the federal government is formally investigating NIST’s handling of the National Vulnerability Database.
@react-oauth/google
Advanced tools
@react-oauth/google is an npm package that provides a set of React components and hooks to easily integrate Google OAuth authentication into your React applications. It simplifies the process of authenticating users with their Google accounts and handling OAuth tokens.
Google Login Button
This feature allows you to add a Google Login button to your React application. When the user clicks the button, they can log in with their Google account. The `onSuccess` and `onFailure` callbacks handle the login response.
import { GoogleOAuthProvider, GoogleLogin } from '@react-oauth/google';
function App() {
const handleLoginSuccess = (response) => {
console.log('Login Success:', response);
};
const handleLoginFailure = (error) => {
console.log('Login Failed:', error);
};
return (
<GoogleOAuthProvider clientId="YOUR_GOOGLE_CLIENT_ID">
<GoogleLogin
onSuccess={handleLoginSuccess}
onFailure={handleLoginFailure}
/>
</GoogleOAuthProvider>
);
}
export default App;
Google Logout Button
This feature allows you to add a Google Logout button to your React application. When the user clicks the button, they will be logged out of their Google account. The `onLogoutSuccess` callback handles the logout response.
import { GoogleOAuthProvider, GoogleLogout } from '@react-oauth/google';
function App() {
const handleLogoutSuccess = () => {
console.log('Logout Success');
};
return (
<GoogleOAuthProvider clientId="YOUR_GOOGLE_CLIENT_ID">
<GoogleLogout
onLogoutSuccess={handleLogoutSuccess}
/>
</GoogleOAuthProvider>
);
}
export default App;
Fetching User Profile
This feature allows you to fetch the user's profile information after they have logged in with their Google account. The `useGoogleLogin` hook is used to initiate the login process, and the user's profile information is fetched using the access token.
import { GoogleOAuthProvider, useGoogleLogin } from '@react-oauth/google';
import axios from 'axios';
function App() {
const login = useGoogleLogin({
onSuccess: async (tokenResponse) => {
const userInfo = await axios.get('https://www.googleapis.com/oauth2/v3/userinfo', {
headers: {
Authorization: `Bearer ${tokenResponse.access_token}`,
},
});
console.log('User Info:', userInfo.data);
},
});
return (
<GoogleOAuthProvider clientId="YOUR_GOOGLE_CLIENT_ID">
<button onClick={() => login()}>Login with Google</button>
</GoogleOAuthProvider>
);
}
export default App;
react-google-login is a popular package for integrating Google login into React applications. It provides a Google login button and handles the OAuth flow. Compared to @react-oauth/google, it offers similar functionality but may have a different API and fewer features.
react-oauth is a more general OAuth package for React that supports multiple OAuth providers, including Google. It provides hooks and components for handling OAuth authentication. Compared to @react-oauth/google, it offers broader support for different providers but may require more configuration.
react-social-login is a package that supports multiple social login providers, including Google. It provides a unified API for handling social logins. Compared to @react-oauth/google, it offers a more comprehensive solution for integrating various social logins but may have a more complex setup.
FAQs
Google OAuth2 using Google Identity Services for React 🚀
The npm package @react-oauth/google receives a total of 315,864 weekly downloads. As such, @react-oauth/google popularity was classified as popular.
We found that @react-oauth/google demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
As vulnerability data bottlenecks grow, the federal government is formally investigating NIST’s handling of the National Vulnerability Database.
Research
Security News
Socket’s Threat Research Team has uncovered 60 npm packages using post-install scripts to silently exfiltrate hostnames, IP addresses, DNS servers, and user directories to a Discord-controlled endpoint.
Security News
TypeScript Native Previews offers a 10x faster Go-based compiler, now available on npm for public testing with early editor and language support.